FFmpeg
|
asfdec.c
Go to the documentation of this file.
47 char stream_languages[128][6]; ///< max number of streams, language for each (RFC1766, e.g. en-US)
85 { "no_resync_search", "Don't try to resynchronize by looking for a certain optional start code", offsetof(ASFContext, no_resync_search), AV_OPT_TYPE_INT, { .i64 = 0 }, 0, 1, AV_OPT_FLAG_DECODING_PARAM },
381 if (fsize <= 0 || (int64_t)asf->hdr.file_size <= 0 || FFABS(fsize - (int64_t)asf->hdr.file_size) < 10000)
532 avio_rl32(pb); // flags (reliable,seekable,no_cleanpoints?,resend-live-cleanpoints, rest of bits reserved)
790 av_get_packet(pb, &pkt, len); av_hex_dump_log(s, AV_LOG_DEBUG, pkt.data, pkt.size); av_free_packet(&pkt);
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
#define AVFMT_NOBINSEARCH
Format does not allow to fallback to binary search via read_timestamp.
Definition: avformat.h:358
Definition: libavcodec/avcodec.h:100
void av_buffer_unref(AVBufferRef **buf)
Free a given reference and automatically free the buffer if there are no more references to it...
Definition: libavutil/buffer.c:105
int av_add_index_entry(AVStream *st, int64_t pos, int64_t timestamp, int size, int distance, int flags)
Add an index entry into a sorted list.
Definition: libavformat/utils.c:1768
enum AVCodecID ff_codec_get_id(const AVCodecTag *tags, unsigned int tag)
Definition: libavformat/utils.c:2614
static int asf_read_ext_stream_properties(AVFormatContext *s, int64_t size)
Definition: asfdec.c:514
void av_shrink_packet(AVPacket *pkt, int size)
Reduce packet size, correctly zeroing padding.
Definition: avpacket.c:97
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
Definition: opt.h:222
static int read_seek(AVFormatContext *ctx, int stream_index, int64_t timestamp, int flags)
Definition: libcdio.c:153
static int asf_read_metadata(AVFormatContext *s, int64_t size)
Definition: asfdec.c:645
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
AVIndexEntry * index_entries
Only used if the format does not support seeking natively.
Definition: avformat.h:822
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
Definition: avformat.h:456
int ff_id3v2_parse_apic(AVFormatContext *s, ID3v2ExtraMeta **extra_meta)
Create a stream for each APIC (attached picture) extracted from the ID3v2 header. ...
Definition: id3v2.c:827
Definition: libavcodec/avcodec.h:130
int avio_get_str16le(AVIOContext *pb, int maxlen, char *buf, int buflen)
Read a UTF-16 string from pb and convert it to UTF-8.
static int asf_read_file_properties(AVFormatContext *s, int64_t size)
Definition: asfdec.c:323
Macro definitions for various function/variable attributes.
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
AVChapter * avpriv_new_chapter(AVFormatContext *s, int id, AVRational time_base, int64_t start, int64_t end, const char *title)
Add a new chapter.
Definition: libavformat/utils.c:3449
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Public dictionary API.
AVOptions.
Definition: libavcodec/avcodec.h:383
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
uint64_t send_time
time to send file, in 100-nanosecond units invalid if broadcasting (could be ignored) ...
Definition: asf.h:68
int av_get_packet(AVIOContext *s, AVPacket *pkt, int size)
Allocate and read the payload of a packet and initialize its fields with default values.
Definition: libavformat/utils.c:328
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: libavcodec/avcodec.h:110
Definition: libavformat/internal.h:40
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
Definition: avformat.h:581
int av_new_packet(AVPacket *pkt, int size)
Allocate the payload of a packet and initialize its fields with default values.
Definition: avpacket.c:73
Definition: libavcodec/avcodec.h:918
int av_index_search_timestamp(AVStream *st, int64_t timestamp, int flags)
Get the index for a specific timestamp.
Definition: libavformat/utils.c:1811
Definition: id3v2.h:55
int ff_get_wav_header(AVIOContext *pb, AVCodecContext *codec, int size)
const char * av_convert_lang_to(const char *lang, enum AVLangCodespace target_codespace)
Convert a language code to a target codespace.
Definition: avlanguage.c:736
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: asf.h:31
void ff_asfcrypt_dec(const uint8_t key[20], uint8_t *data, int len)
Definition: asfcrypt.c:147
AVBufferRef * buf
A reference to the reference-counted buffer where the packet data is stored.
Definition: libavcodec/avcodec.h:1034
void ff_id3v2_free_extra_meta(ID3v2ExtraMeta **extra_meta)
Free memory allocated parsing special (non-text) metadata.
Definition: id3v2.c:813
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
size_t av_strlcpy(char *dst, const char *src, size_t size)
Copy the string src to dst, but no more than size - 1 bytes, and null-terminate dst.
Definition: avstring.c:82
uint32_t max_pktsize
shall be the same as for min_pktsize invalid if broadcasting
Definition: asf.h:78
int av_reduce(int *dst_num, int *dst_den, int64_t num, int64_t den, int64_t max)
Reduce a fraction.
Definition: rational.c:36
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
Definition: avlanguage.h:28
int64_t av_rescale(int64_t a, int64_t b, int64_t c)
Rescale a 64-bit integer with rounding to nearest.
Definition: mathematics.c:118
#define LEN
#define AV_DICT_DONT_STRDUP_VAL
Take ownership of a value that's been allocated with av_malloc() and chilren.
Definition: dict.h:72
char stream_languages[128][6]
max number of streams, language for each (RFC1766, e.g. en-US)
Definition: asfdec.c:47
internal header for RIFF based (de)muxers do NOT include this in end user applications ...
const ff_asf_guid ff_asf_ext_stream_embed_stream_header
Definition: asf.c:100
#define AV_DISPOSITION_ATTACHED_PIC
The stream is stored in the file as an attached picture/"cover art" (e.g.
Definition: avformat.h:627
void ff_id3v2_read(AVFormatContext *s, const char *magic, ID3v2ExtraMeta **extra_meta)
Read an ID3v2 tag, including supported extra metadata.
Definition: id3v2.c:780
static int asf_read_stream_properties(AVFormatContext *s, int64_t size)
Definition: asfdec.c:347
static int ff_asf_parse_packet(AVFormatContext *s, AVIOContext *pb, AVPacket *pkt)
Parse data from individual ASF packets (which were previously loaded with asf_get_packet()).
Definition: asfdec.c:1113
uint32_t stream_bitrates[128]
max number of streams, bitrate for each (for streaming)
Definition: asfdec.c:45
uint64_t create_time
time of creation, in 100-nanosecond units since 1.1.1601 invalid if broadcasting
Definition: asf.h:64
AVBufferRef * av_buffer_alloc(int size)
Allocate an AVBuffer of the given size using av_malloc().
Definition: libavutil/buffer.c:65
static int64_t asf_read_pts(AVFormatContext *s, int stream_index, int64_t *ppos, int64_t pos_limit)
Definition: asfdec.c:1389
static int asf_read_seek(AVFormatContext *s, int stream_index, int64_t pts, int flags)
Definition: asfdec.c:1499
static int ff_asf_get_packet(AVFormatContext *s, AVIOContext *pb)
Load a single ASF packet into the demuxer.
Definition: asfdec.c:886
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
#define AVFMT_NOGENSEARCH
Format does not allow to fallback to generic search.
Definition: avformat.h:359
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
Definition: asfdec.c:41
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
static void asf_build_simple_index(AVFormatContext *s, int stream_index)
Definition: asfdec.c:1442
byte swapping routines
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
static int asf_read_ext_content_desc(AVFormatContext *s, int64_t size)
Definition: asfdec.c:591
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
int av_read_frame(AVFormatContext *s, AVPacket *pkt)
Return the next frame of a stream.
Definition: libavformat/utils.c:1530
static int asf_read_frame_header(AVFormatContext *s, AVIOContext *pb)
Definition: asfdec.c:985
full parsing and repack of the first frame only, only implemented for H.264 currently ...
Definition: avformat.h:585
static int asf_read_content_desc(AVFormatContext *s, int64_t size)
Definition: asfdec.c:572
Synth Windw Norm while(pin< pend)%Until the end...%---Analysis x_w
uint32_t preroll
timestamp of the first packet, in milliseconds if nonzero - subtract from time
Definition: asf.h:70
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
common internal and external API header
void ff_get_guid(AVIOContext *s, ff_asf_guid *g)
void ff_metadata_conv(AVDictionary **pm, const AVMetadataConv *d_conv, const AVMetadataConv *s_conv)
Definition: libavformat/metadata.c:26
int64_t avio_seek_time(AVIOContext *h, int stream_index, int64_t timestamp, int flags)
Seek to a given timestamp relative to some component stream.
Definition: aviobuf.c:866
static int asf_read_language_list(AVFormatContext *s, int64_t size)
Definition: asfdec.c:625
struct AVPacket::@35 * side_data
Additional packet data that can be provided by the container.
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
Definition: avutil.h:143
void av_hex_dump_log(void *avcl, int level, const uint8_t *buf, int size)
Send a nice hexadecimal dump of a buffer to the log.
Definition: libavformat/utils.c:3773
uint8_t * av_packet_new_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int size)
Allocate new information of a packet.
Definition: avpacket.c:264
static void get_tag(AVFormatContext *s, const char *key, int type, int len, int type2_size)
Definition: asfdec.c:277
enum AVDiscard discard
Selects which packets can be discarded at will and do not need to be demuxed.
Definition: avformat.h:702
static av_always_inline int ff_guidcmp(const void *g1, const void *g2)
Definition: riff.h:68
int request_probe
stream probing state -1 -> probing finished 0 -> no probing requested rest -> perform probing with re...
Definition: avformat.h:834
uint32_t max_bitrate
bandwidth of stream in bps should be the sum of bitrates of the individual media streams ...
Definition: asf.h:80
Definition: asf.h:60
AVPacket attached_pic
For streams with AV_DISPOSITION_ATTACHED_PIC disposition, this packet will contain the attached pictu...
Definition: avformat.h:725
static int asf_read_packet(AVFormatContext *s, AVPacket *pkt)
Definition: asfdec.c:1326
int ff_seek_frame_binary(AVFormatContext *s, int stream_index, int64_t target_ts, int flags)
Perform a binary search using av_index_search_timestamp() and AVInputFormat.read_timestamp().
Definition: libavformat/utils.c:1827
Generated on Mon Nov 18 2024 06:51:52 for FFmpeg by
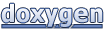