FFmpeg
|
ID3v2 header parser. More...
#include "config.h"
#include "id3v2.h"
#include "id3v1.h"
#include "libavutil/avstring.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/dict.h"
#include "avio_internal.h"
#include "internal.h"
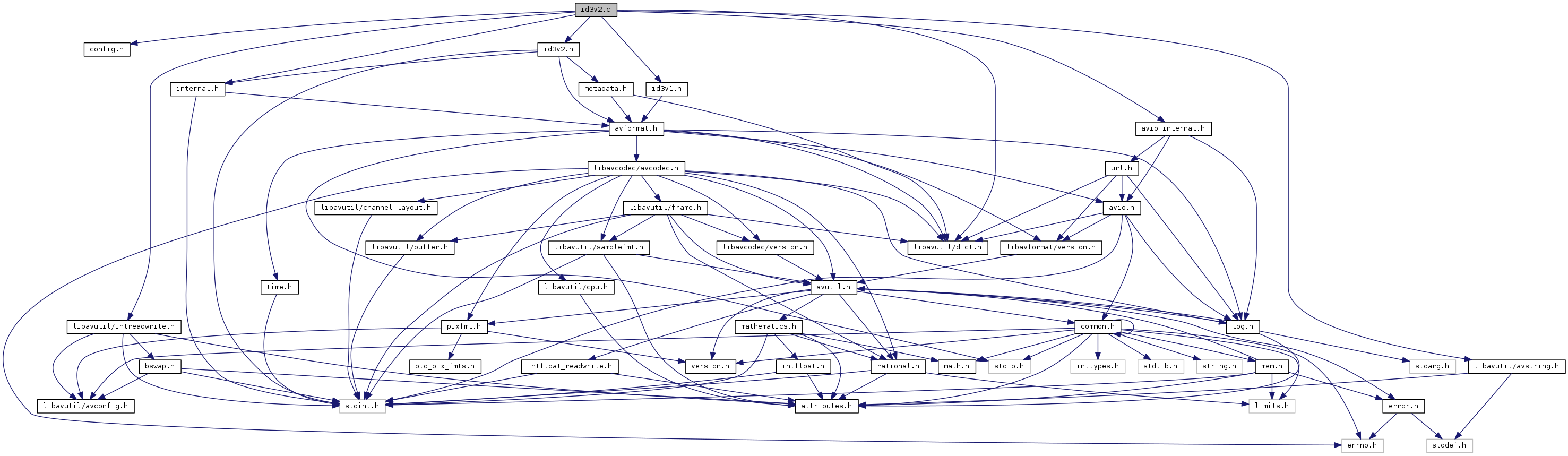
Go to the source code of this file.
Data Structures | |
struct | ID3v2EMFunc |
Typedefs | |
typedef struct ID3v2EMFunc | ID3v2EMFunc |
Functions | |
int | ff_id3v2_match (const uint8_t *buf, const char *magic) |
Detect ID3v2 Header. More... | |
int | ff_id3v2_tag_len (const uint8_t *buf) |
Get the length of an ID3v2 tag. More... | |
static unsigned int | get_size (AVIOContext *s, int len) |
static void | free_geobtag (void *obj) |
Free GEOB type extra metadata. More... | |
static int | decode_str (AVFormatContext *s, AVIOContext *pb, int encoding, uint8_t **dst, int *maxread) |
Decode characters to UTF-8 according to encoding type. More... | |
static void | read_ttag (AVFormatContext *s, AVIOContext *pb, int taglen, const char *key) |
Parse a text tag. More... | |
static void | read_geobtag (AVFormatContext *s, AVIOContext *pb, int taglen, char *tag, ID3v2ExtraMeta **extra_meta) |
Parse GEOB tag into a ID3v2ExtraMetaGEOB struct. More... | |
static int | is_number (const char *str) |
static AVDictionaryEntry * | get_date_tag (AVDictionary *m, const char *tag) |
static void | merge_date (AVDictionary **m) |
static void | free_apic (void *obj) |
static void | read_apic (AVFormatContext *s, AVIOContext *pb, int taglen, char *tag, ID3v2ExtraMeta **extra_meta) |
static void | read_chapter (AVFormatContext *s, AVIOContext *pb, int taglen, char *tag, ID3v2ExtraMeta **extra_meta) |
static const ID3v2EMFunc * | get_extra_meta_func (const char *tag, int isv34) |
Get the corresponding ID3v2EMFunc struct for a tag. More... | |
static void | ff_id3v2_parse (AVFormatContext *s, int len, uint8_t version, uint8_t flags, ID3v2ExtraMeta **extra_meta) |
void | ff_id3v2_read (AVFormatContext *s, const char *magic, ID3v2ExtraMeta **extra_meta) |
Read an ID3v2 tag, including supported extra metadata. More... | |
void | ff_id3v2_free_extra_meta (ID3v2ExtraMeta **extra_meta) |
Free memory allocated parsing special (non-text) metadata. More... | |
int | ff_id3v2_parse_apic (AVFormatContext *s, ID3v2ExtraMeta **extra_meta) |
Create a stream for each APIC (attached picture) extracted from the ID3v2 header. More... | |
Variables | |
const AVMetadataConv | ff_id3v2_34_metadata_conv [] |
const AVMetadataConv | ff_id3v2_4_metadata_conv [] |
static const AVMetadataConv | id3v2_2_metadata_conv [] |
const char | ff_id3v2_tags [][4] |
A list of text information frames allowed in both ID3 v2.3 and v2.4 http://www.id3.org/id3v2.4.0-frames http://www.id3.org/id3v2.4.0-changes. More... | |
const char | ff_id3v2_4_tags [][4] |
ID3v2.4-only text information frames. More... | |
const char | ff_id3v2_3_tags [][4] |
ID3v2.3-only text information frames. More... | |
const char * | ff_id3v2_picture_types [21] |
const CodecMime | ff_id3v2_mime_tags [] |
static const ID3v2EMFunc | id3v2_extra_meta_funcs [] |
Detailed Description
ID3v2 header parser.
Specifications available at: http://id3.org/Developer_Information
Definition in file id3v2.c.
Typedef Documentation
typedef struct ID3v2EMFunc ID3v2EMFunc |
Function Documentation
|
static |
Decode characters to UTF-8 according to encoding type.
The decoded buffer is always null terminated. Stop reading when either *maxread bytes are read from pb or U+0000 character is found.
- Parameters
-
dst Pointer where the address of the buffer with the decoded bytes is stored. Buffer must be freed by caller. maxread Pointer to maximum number of characters to read from the AVIOContext. After execution the value is decremented by the number of bytes actually read.
- Returns
- 0 if no error occurred, dst is uninitialized on error
Definition at line 197 of file id3v2.c.
Referenced by read_apic(), read_geobtag(), and read_ttag().
void ff_id3v2_free_extra_meta | ( | ID3v2ExtraMeta ** | extra_meta | ) |
Free memory allocated parsing special (non-text) metadata.
- Parameters
-
extra_meta Pointer to a pointer to the head of a ID3v2ExtraMeta list, *extra_meta is set to NULL.
Definition at line 813 of file id3v2.c.
Referenced by aiff_read_header(), avformat_open_input(), get_id3_tag(), and oma_read_header().
int ff_id3v2_match | ( | const uint8_t * | buf, |
const char * | magic | ||
) |
Detect ID3v2 Header.
- Parameters
-
buf must be ID3v2_HEADER_SIZE byte long magic magic bytes to identify the header. If in doubt, use ID3v2_DEFAULT_MAGIC.
Definition at line 139 of file id3v2.c.
Referenced by av_probe_input_format3(), ff_id3v2_read(), mp3_read_probe(), and oma_read_probe().
|
static |
Definition at line 574 of file id3v2.c.
Referenced by ff_id3v2_read().
int ff_id3v2_parse_apic | ( | AVFormatContext * | s, |
ID3v2ExtraMeta ** | extra_meta | ||
) |
Create a stream for each APIC (attached picture) extracted from the ID3v2 header.
Definition at line 827 of file id3v2.c.
Referenced by aiff_read_header(), avformat_open_input(), and get_id3_tag().
void ff_id3v2_read | ( | AVFormatContext * | s, |
const char * | magic, | ||
ID3v2ExtraMeta ** | extra_meta | ||
) |
Read an ID3v2 tag, including supported extra metadata.
- Parameters
-
extra_meta If not NULL, extra metadata is parsed into a list of ID3v2ExtraMeta structs and *extra_meta points to the head of the list
Definition at line 780 of file id3v2.c.
Referenced by aiff_read_header(), avformat_open_input(), get_id3_tag(), and oma_read_header().
int ff_id3v2_tag_len | ( | const uint8_t * | buf | ) |
Get the length of an ID3v2 tag.
- Parameters
-
buf must be ID3v2_HEADER_SIZE bytes long and point to the start of an already detected ID3v2 tag
Definition at line 152 of file id3v2.c.
Referenced by av_probe_input_format3(), mp3_read_probe(), and oma_read_probe().
Definition at line 433 of file id3v2.c.
Referenced by read_apic().
Free GEOB type extra metadata.
Definition at line 175 of file id3v2.c.
Referenced by read_geobtag().
|
static |
Definition at line 393 of file id3v2.c.
Referenced by merge_date().
|
static |
Get the corresponding ID3v2EMFunc struct for a tag.
- Parameters
-
isv34 Determines if v2.2 or v2.3/4 strings are used
- Returns
- A pointer to the ID3v2EMFunc struct if found, NULL otherwise.
Definition at line 560 of file id3v2.c.
Referenced by ff_id3v2_free_extra_meta(), and ff_id3v2_parse().
|
static |
Definition at line 164 of file id3v2.c.
Referenced by ff_id3v2_parse().
|
static |
Definition at line 387 of file id3v2.c.
Referenced by get_date_tag().
|
static |
Definition at line 402 of file id3v2.c.
Referenced by ff_id3v2_read().
|
static |
|
static |
|
static |
Parse GEOB tag into a ID3v2ExtraMetaGEOB struct.
|
static |
Variable Documentation
const AVMetadataConv ff_id3v2_34_metadata_conv[] |
Definition at line 43 of file id3v2.c.
Referenced by ff_id3v2_write_metadata().
const char ff_id3v2_3_tags[][4] |
ID3v2.3-only text information frames.
Definition at line 98 of file id3v2.c.
Referenced by ff_id3v2_write_metadata().
const AVMetadataConv ff_id3v2_4_metadata_conv[] |
Definition at line 61 of file id3v2.c.
Referenced by ff_id3v2_write_metadata().
const char ff_id3v2_4_tags[][4] |
ID3v2.4-only text information frames.
Definition at line 92 of file id3v2.c.
Referenced by ff_id3v2_write_metadata().
const CodecMime ff_id3v2_mime_tags[] |
Definition at line 127 of file id3v2.c.
Referenced by asf_read_picture(), ff_id3v2_write_apic(), parse_picture(), query_codec(), and read_apic().
const char* ff_id3v2_picture_types[21] |
Definition at line 103 of file id3v2.c.
Referenced by asf_read_picture(), ff_id3v2_write_apic(), parse_picture(), and read_apic().
const char ff_id3v2_tags[][4] |
A list of text information frames allowed in both ID3 v2.3 and v2.4 http://www.id3.org/id3v2.4.0-frames http://www.id3.org/id3v2.4.0-changes.
Definition at line 84 of file id3v2.c.
Referenced by ff_id3v2_write_metadata().
|
static |
|
static |
Generated on Tue Jan 21 2025 06:52:33 for FFmpeg by
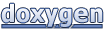