FFmpeg
|
omadec.c
Go to the documentation of this file.
76 static void hex_log(AVFormatContext *s, int level, const char *name, const uint8_t *value, int len)
233 hex_log(s, AV_LOG_DEBUG, "CBC-MAC", &gdata[OMA_ENC_HEADER_SIZE+oc->k_size+oc->e_size+oc->i_size], 8);
283 if (memcmp(buf, ((const uint8_t[]){'E', 'A', '3'}),3) || buf[4] != 0 || buf[5] != EA3_HEADER_SIZE) {
429 static int oma_read_seek(struct AVFormatContext *s, int stream_index, int64_t timestamp, int flags)
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
enum AVCodecID ff_codec_get_id(const AVCodecTag *tags, unsigned int tag)
Definition: libavformat/utils.c:2614
Definition: id3v2.h:61
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
static int read_seek(AVFormatContext *ctx, int stream_index, int64_t timestamp, int flags)
Definition: libcdio.c:153
void av_des_mac(AVDES *d, uint8_t *dst, const uint8_t *src, int count)
Calculates CBC-MAC using the DES algorithm.
Definition: des.c:335
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
struct OMAContext OMAContext
Definition: omadec.c:58
Definition: avformat.h:456
static int kset(AVFormatContext *s, const uint8_t *r_val, const uint8_t *n_val, int len)
Definition: omadec.c:87
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
static int oma_read_packet(AVFormatContext *s, AVPacket *pkt)
Definition: omadec.c:382
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: oma.h:34
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
Definition: libavformat/internal.h:35
int av_get_packet(AVIOContext *s, AVPacket *pkt, int size)
Allocate and read the payload of a packet and initialize its fields with default values.
Definition: libavformat/utils.c:328
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
full parsing and repack with timestamp and position generation by parser for raw this assumes that ea...
Definition: avformat.h:586
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
int av_get_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2690
Definition: oma.h:36
Definition: id3v2.h:55
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
int av_des_init(AVDES *d, const uint8_t *key, int key_bits, av_unused int decrypt)
Definition: des.c:289
void ff_id3v2_free_extra_meta(ID3v2ExtraMeta **extra_meta)
Free memory allocated parsing special (non-text) metadata.
Definition: id3v2.c:813
Definition: avutil.h:144
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
audio channel layout utility functions
int ff_pcm_read_seek(AVFormatContext *s, int stream_index, int64_t timestamp, int flags)
Definition: libavformat/pcm.c:46
void ff_id3v2_read(AVFormatContext *s, const char *magic, ID3v2ExtraMeta **extra_meta)
Read an ID3v2 tag, including supported extra metadata.
Definition: id3v2.c:780
static int decrypt_init(AVFormatContext *s, ID3v2ExtraMeta *em, uint8_t *header)
Definition: omadec.c:178
static void hex_log(AVFormatContext *s, int level, const char *name, const uint8_t *value, int len)
Definition: omadec.c:76
Definition: oma.h:33
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
Definition: oma.h:35
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static int oma_read_seek(struct AVFormatContext *s, int stream_index, int64_t timestamp, int flags)
Definition: omadec.c:429
void av_des_crypt(AVDES *d, uint8_t *dst, const uint8_t *src, int count, uint8_t *iv, int decrypt)
Encrypts / decrypts using the DES algorithm.
Definition: des.c:331
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
int ff_id3v2_match(const uint8_t *buf, const char *magic)
Detect ID3v2 Header.
Definition: id3v2.c:139
int64_t start_time
Decoding: pts of the first frame of the stream in presentation order, in stream time base...
Definition: avformat.h:689
static int nprobe(AVFormatContext *s, uint8_t *enc_header, int size, const uint8_t *n_val)
Definition: omadec.c:142
static int rprobe(AVFormatContext *s, uint8_t *enc_header, const uint8_t *r_val)
Definition: omadec.c:115
char * ff_data_to_hex(char *buf, const uint8_t *src, int size, int lowercase)
Definition: libavformat/utils.c:3895
Generated on Mon Nov 18 2024 06:51:58 for FFmpeg by
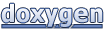