FFmpeg
|
Options definition for AVCodecContext. More...
#include "avcodec.h"
#include "internal.h"
#include "libavutil/avassert.h"
#include "libavutil/mem.h"
#include "libavutil/opt.h"
#include <float.h>
#include <string.h>
#include "options_table.h"
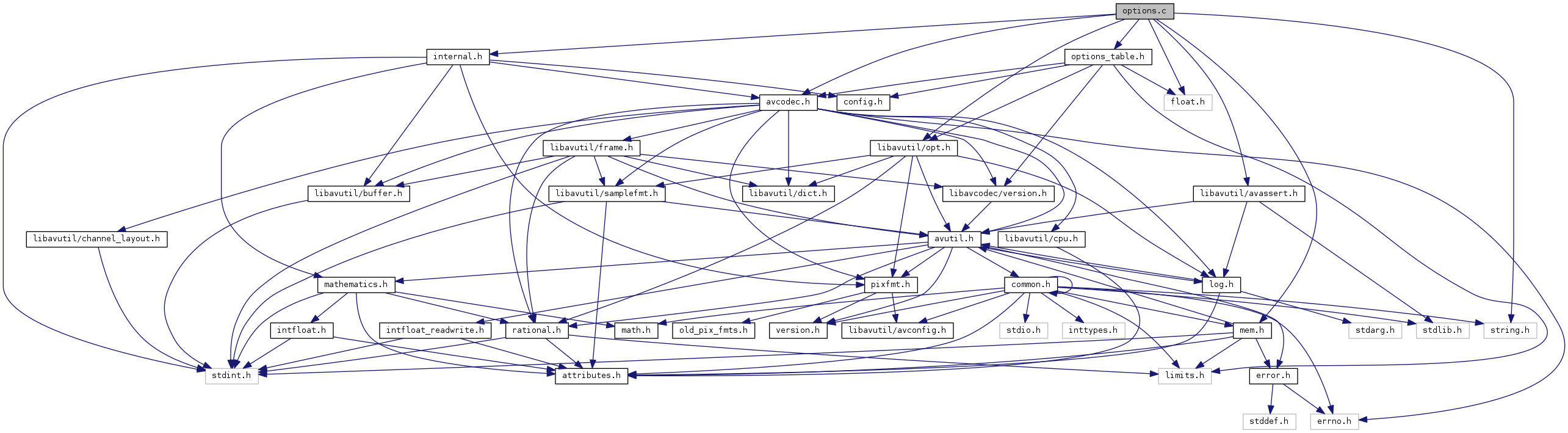
Go to the source code of this file.
Macros | |
#define | alloc_and_copy_or_fail(obj, size, pad) |
#define | FOFFSET(x) offsetof(AVFrame,x) |
#define | SROFFSET(x) offsetof(AVSubtitleRect,x) |
Variables | |
static const AVClass | av_codec_context_class |
static const AVOption | frame_options [] |
static const AVClass | av_frame_class |
static const AVOption | subtitle_rect_options [] |
static const AVClass | av_subtitle_rect_class |
Detailed Description
Options definition for AVCodecContext.
Definition in file libavcodec/options.c.
Macro Definition Documentation
#define alloc_and_copy_or_fail | ( | obj, | |
size, | |||
pad | |||
) |
Referenced by avcodec_copy_context().
Definition at line 244 of file libavcodec/options.c.
#define SROFFSET | ( | x | ) | offsetof(AVSubtitleRect,x) |
Definition at line 271 of file libavcodec/options.c.
Function Documentation
Definition at line 54 of file libavcodec/options.c.
Definition at line 46 of file libavcodec/options.c.
|
static |
Definition at line 37 of file libavcodec/options.c.
|
static |
Definition at line 70 of file libavcodec/options.c.
Variable Documentation
|
static |
Definition at line 77 of file libavcodec/options.c.
Referenced by avcodec_get_class(), and avcodec_get_context_defaults3().
|
static |
Definition at line 259 of file libavcodec/options.c.
Referenced by avcodec_get_frame_class().
|
static |
Definition at line 284 of file libavcodec/options.c.
Referenced by avcodec_get_subtitle_rect_class().
|
static |
Definition at line 246 of file libavcodec/options.c.
Generated on Mon Nov 18 2024 06:52:08 for FFmpeg by
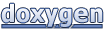