FFmpeg
|
rv30.c
Go to the documentation of this file.
104 static const int rv30_p_types[6] = { RV34_MB_SKIP, RV34_MB_P_16x16, RV34_MB_P_8x8, -1, RV34_MB_TYPE_INTRA, RV34_MB_TYPE_INTRA16x16 };
105 static const int rv30_b_types[6] = { RV34_MB_SKIP, RV34_MB_B_DIRECT, RV34_MB_B_FORWARD, RV34_MB_B_BACKWARD, RV34_MB_TYPE_INTRA, RV34_MB_TYPE_INTRA16x16 };
#define C
static const uint8_t rv30_loop_filt_lim[32]
Loop filter limits are taken from this table.
Definition: rv30data.h:178
Bidirectionally predicted B-frame macroblock, no motion vectors.
Definition: rv34.h:50
mpegvideo header.
static av_cold int rv30_decode_init(AVCodecContext *avctx)
Initialize decoder.
Definition: rv30.c:248
RV30 and RV40 decoder common data declarations.
static int rv30_decode_intra_types(RV34DecContext *r, GetBitContext *gb, int8_t *dst)
Decode 4x4 intra types array.
Definition: rv30.c:74
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
static int rv30_decode_mb_info(RV34DecContext *r)
Decode macroblock information.
Definition: rv30.c:102
static const uint8_t rv30_itype_from_context[900]
This table is used for retrieving the current intra type based on its neighbors and adjustment provid...
Definition: rv30data.h:63
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
external API header
#define ONLY_IF_THREADS_ENABLED(x)
Define a function with only the non-default version specified.
Definition: libavutil/internal.h:162
int(* parse_slice_header)(struct RV34DecContext *r, GetBitContext *gb, SliceInfo *si)
Definition: rv34.h:124
static const uint8_t rv30_itype_code[9 *9 *2]
This table is used for storing the differences between the predicted and the real intra type...
Definition: rv30data.h:42
int ff_rv34_decode_init_thread_copy(AVCodecContext *avctx)
Definition: rv34.c:1516
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
static void rv30_weak_loop_filter(uint8_t *src, const int step, const int stride, const int lim)
Definition: rv30.c:124
Definition: libavcodec/avcodec.h:171
int ff_rv34_decode_frame(AVCodecContext *avctx, void *data, int *got_picture_ptr, AVPacket *avpkt)
Definition: rv34.c:1610
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
int(* decode_intra_types)(struct RV34DecContext *r, GetBitContext *gb, int8_t *dst)
Definition: rv34.h:126
Definition: get_bits.h:54
int mb_stride
mb_width+1 used for some arrays to allow simple addressing of left & top MBs without sig11 ...
Definition: mpegvideo.h:278
#define CODEC_CAP_FRAME_THREADS
Codec supports frame-level multithreading.
Definition: libavcodec/avcodec.h:810
int ff_rv34_decode_update_thread_context(AVCodecContext *dst, const AVCodecContext *src)
Definition: rv34.c:1534
int ff_rv34_get_start_offset(GetBitContext *gb, int mb_size)
Decode starting slice position.
Definition: rv34.c:329
static int rv30_parse_slice_header(RV34DecContext *r, GetBitContext *gb, SliceInfo *si)
Definition: rv30.c:35
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
miscellaneous RV30 tables
exp golomb vlc stuff
int uvlinesize
line size, for chroma in bytes, may be different from width
Definition: mpegvideo.h:284
void(* loop_filter)(struct RV34DecContext *r, int row)
Definition: rv34.h:127
trying all byte sequences megabyte in length and selecting the best looking sequence will yield cases to try But a word about which is also called distortion Distortion can be quantified by almost any quality measurement one chooses the sum of squared differences is used but more complex methods that consider psychovisual effects can be used as well It makes no difference in this discussion First step
Definition: rate_distortion.txt:12
Generated on Wed Jul 2 2025 06:53:38 for FFmpeg by
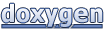