FFmpeg
|
lagarith.c
Go to the documentation of this file.
42 FRAME_OLD_ARITH_RGB = 7, /**< obsolete arithmetic coded RGB (no longer encoded by upstream since version 1.1.0) */
151 av_log(rac->avctx, AV_LOG_ERROR, "Integer overflow encountered in cumulative probability calculation.\n");
static uint8_t lag_get_rac(lag_rac *l)
Decode a single byte from the compressed plane described by *l.
Definition: lagarithrac.h:73
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
void ff_lag_rac_init(lag_rac *l, GetBitContext *gb, int length)
Definition: lagarithrac.c:33
static int lag_decode_arith_plane(LagarithContext *l, uint8_t *dst, int width, int height, int stride, const uint8_t *src, int src_size)
Definition: lagarith.c:406
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static int lag_decode_zero_run_line(LagarithContext *l, uint8_t *dst, const uint8_t *src, const uint8_t *src_end, int width, int esc_count)
Definition: lagarith.c:352
Lagarith range decoder.
static void lag_pred_line(LagarithContext *l, uint8_t *buf, int width, int stride, int line)
Definition: lagarith.c:245
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
bitstream reader API header.
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
struct LagarithContext LagarithContext
Definition: libavcodec/avcodec.h:250
Definition: lagarith.c:49
void(* add_hfyu_median_prediction)(uint8_t *dst, const uint8_t *top, const uint8_t *diff, int w, int *left, int *left_top)
Definition: dsputil.h:204
Multithreading support functions.
static void add_lag_median_prediction(uint8_t *dst, uint8_t *src1, uint8_t *diff, int w, int *left, int *left_top)
Definition: lagarith.c:221
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: graph2dot.c:48
external API header
planar YUV 4:2:2, 16bpp, (1 Cr & Cb sample per 2x1 Y samples)
Definition: pixfmt.h:72
static uint64_t softfloat_reciprocal(uint32_t denom)
Compute the 52bit mantissa of 1/(double)denom.
Definition: lagarith.c:66
obsolete arithmetic coded RGB (no longer encoded by upstream since version 1.1.0) ...
Definition: lagarith.c:42
static int lag_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Decode a frame.
Definition: lagarith.c:508
Definition: lagarithrac.h:39
Definition: thread.h:35
static int lag_decode_prob(GetBitContext *gb, uint32_t *value)
Definition: lagarith.c:101
static int lag_decode_line(LagarithContext *l, lag_rac *rac, uint8_t *dst, int width, int stride, int esc_count)
Definition: lagarith.c:310
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
static uint32_t softfloat_mul(uint32_t x, uint64_t mantissa)
(uint32_t)(x*f), where f has the given mantissa, and exponent 0 Used in combination with softfloat_re...
Definition: lagarith.c:85
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
int ff_thread_get_buffer(AVCodecContext *avctx, ThreadFrame *f, int flags)
Wrapper around get_buffer() for frame-multithreaded codecs.
Definition: pthread.c:1066
static int lag_read_prob_header(lag_rac *rac, GetBitContext *gb)
Definition: lagarith.c:135
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
Definition: get_bits.h:54
#define CODEC_CAP_FRAME_THREADS
Codec supports frame-level multithreading.
Definition: libavcodec/avcodec.h:810
int(* add_hfyu_left_prediction)(uint8_t *dst, const uint8_t *src, int w, int left)
Definition: dsputil.h:205
DSP utils.
static void lag_pred_line_yuy2(LagarithContext *l, uint8_t *buf, int width, int stride, int line, int is_luma)
Definition: lagarith.c:272
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Generated on Tue Jul 1 2025 06:52:26 for FFmpeg by
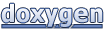