FFmpeg
|
alsdec.c
Go to the documentation of this file.
177 int chan_config_info; ///< mapping of channels to loudspeaker locations. Unused until setting channel configuration is implemented.
222 int32_t *lpc_cof_reversed_buffer; ///< temporary buffer to set up a reversed versio of lpc_cof_buffer
static int decode_var_block_data(ALSDecContext *ctx, ALSBlockData *bd)
Decode the block data for a non-constant block.
Definition: alsdec.c:871
Definition: start.py:1
int msb_first
1 = original CRC calculated on big-endian system, 0 = little-endian
Definition: alsdec.c:161
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
uint32_t av_crc(const AVCRC *ctx, uint32_t crc, const uint8_t *buffer, size_t length)
Calculate the CRC of a block.
Definition: crc.c:275
Definition: alsdec.c:153
uint8_t * crc_buffer
buffer of byte order corrected samples used for CRC check
Definition: alsdec.c:229
static const int16_t mcc_weightings[]
Inter-channel weighting factors for multi-channel correlation.
Definition: alsdec.c:120
if max(w)>1 w=0.9 *w/max(w)
int rlslms
use "Recursive Least Square-Least Mean Square" predictor: 1 = on, 0 = off
Definition: alsdec.c:176
static int check_specific_config(ALSDecContext *ctx)
Check the ALSSpecificConfig for unsupported features.
Definition: alsdec.c:427
static int read_frame_data(ALSDecContext *ctx, unsigned int ra_frame)
Read the frame data.
Definition: alsdec.c:1311
int32_t * lpc_cof_reversed_buffer
temporary buffer to set up a reversed versio of lpc_cof_buffer
Definition: alsdec.c:222
Block Gilbert-Moore decoder header.
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
static int read_decode_block(ALSDecContext *ctx, ALSBlockData *bd)
Read and decode block data successively.
Definition: alsdec.c:1023
#define INTERLEAVE_OUTPUT(bps)
static int32_t decode_rice(GetBitContext *gb, unsigned int k)
Read and decode a Rice codeword.
Definition: alsdec.c:475
static int get_sbits_long(GetBitContext *s, int n)
Read 0-32 bits as a signed integer.
Definition: get_bits.h:344
Definition: alsdec.c:193
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
static const uint8_t ltp_gain_values[4][4]
Gain values of p(0) for long-term prediction.
Definition: alsdec.c:109
static av_cold int decode_init(AVCodecContext *avctx)
Initialize the ALS decoder.
Definition: alsdec.c:1610
Definition: alsdec.c:233
static void decode_const_block_data(ALSDecContext *ctx, ALSBlockData *bd)
Decode the block data for a constant block.
Definition: alsdec.c:596
const AVCRC * av_crc_get_table(AVCRCId crc_id)
Get an initialized standard CRC table.
Definition: crc.c:261
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
bitstream reader API header.
static int decode_blocks_ind(ALSDecContext *ctx, unsigned int ra_frame, unsigned int c, const unsigned int *div_blocks, unsigned int *js_blocks)
Decode blocks independently.
Definition: alsdec.c:1056
void ff_bgmc_decode_init(GetBitContext *gb, unsigned int *h, unsigned int *l, unsigned int *v)
Initialize decoding and reads the first value.
Definition: bgmc.c:486
static void zero_remaining(unsigned int b, unsigned int b_max, const unsigned int *div_blocks, int32_t *buf)
Compute the number of samples left to decode for the current frame and sets these samples to zero...
Definition: alsdec.c:1041
static av_cold void dprint_specific_config(ALSDecContext *ctx)
Definition: alsdec.c:252
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: alsdec.c:183
static const int16_t parcor_scaled_values[]
Scaled PARCOR values used for the first two PARCOR coefficients.
Definition: alsdec.c:70
int32_t ** lpc_cof
coefficients of the direct form prediction filter for a channel
Definition: alsdec.c:220
int chan_config_info
mapping of channels to loudspeaker locations. Unused until setting channel configuration is implement...
Definition: alsdec.c:177
Definition: avutil.h:144
void ff_bgmc_end(uint8_t **cf_lut, int **cf_lut_status)
Release the lookup table arrays.
Definition: bgmc.c:478
Definition: crc.h:35
int32_t * prev_raw_samples
contains unshifted raw samples from the previous block
Definition: alsdec.c:226
static int decode_blocks(ALSDecContext *ctx, unsigned int ra_frame, unsigned int c, const unsigned int *div_blocks, unsigned int *js_blocks)
Decode blocks dependently.
Definition: alsdec.c:1095
external API header
Definition: mpeg4audio.h:29
Definition: alsdec.c:157
int ff_bgmc_init(AVCodecContext *avctx, uint8_t **cf_lut, int **cf_lut_status)
Initialize the lookup table arrays.
Definition: bgmc.c:459
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
static int read_var_block_data(ALSDecContext *ctx, ALSBlockData *bd)
Read the block data for a non-constant block.
Definition: alsdec.c:610
Definition: alsdec.c:152
void ff_bgmc_decode(GetBitContext *gb, unsigned int num, int32_t *dst, int delta, unsigned int sx, unsigned int *h, unsigned int *l, unsigned int *v, uint8_t *cf_lut, int *cf_lut_status)
Read and decode a block Gilbert-Moore coded symbol.
Definition: bgmc.c:503
static av_cold int decode_end(AVCodecContext *avctx)
Uninitialize the ALS decoder.
Definition: alsdec.c:1575
static int read_const_block_data(ALSDecContext *ctx, ALSBlockData *bd)
Read the block data for a constant block.
Definition: alsdec.c:566
int32_t * raw_other
decoded raw samples of the other channel of a channel pair
Definition: alsdec.c:248
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Return number of bytes per sample.
Definition: samplefmt.c:104
static void parcor_to_lpc(unsigned int k, const int32_t *par, int32_t *cof)
Convert PARCOR coefficient k to direct filter coefficient.
Definition: alsdec.c:493
static int init_get_bits8(GetBitContext *s, const uint8_t *buffer, int byte_size)
Initialize GetBitContext.
Definition: get_bits.h:410
static int decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Decode an ALS frame.
Definition: alsdec.c:1449
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
#define MISSING_ERR(cond, str, errval)
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
void(* bswap_buf)(uint32_t *dst, const uint32_t *src, int w)
Definition: dsputil.h:208
int32_t * lpc_cof_buffer
contains all coefficients of the direct form prediction filter
Definition: alsdec.c:221
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
static const int8_t parcor_rice_table[3][20][2]
Rice parameters and corresponding index offsets for decoding the indices of scaled PARCOR values...
Definition: alsdec.c:49
static av_cold int read_specific_config(ALSDecContext *ctx)
Read an ALSSpecificConfig from a buffer into the output struct.
Definition: alsdec.c:282
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int32_t * raw_buffer
contains all decoded raw samples including carryover samples
Definition: alsdec.c:228
#define CODEC_CAP_SUBFRAMES
Codec can output multiple frames per AVPacket Normally demuxers return one frame at a time...
Definition: libavcodec/avcodec.h:791
int mc_coding
extended inter-channel coding (multi channel coding): 1 = on, 0 = off
Definition: alsdec.c:173
static const uint8_t tail_code[16][6]
Tail codes used in arithmetic coding using block Gilbert-Moore codes.
Definition: alsdec.c:130
Definition: get_bits.h:54
common internal api header.
int32_t * prev_raw_samples
contains unshifted raw samples from the previous block
Definition: alsdec.c:247
static int get_unary(GetBitContext *gb, int stop, int len)
Get unary code of limited length.
Definition: unary.h:33
static av_cold void flush(AVCodecContext *avctx)
Flush (reset) the frame ID after seeking.
Definition: alsdec.c:1767
Definition: alsdec.c:151
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
int avpriv_mpeg4audio_get_config(MPEG4AudioConfig *c, const uint8_t *buf, int bit_size, int sync_extension)
Parse MPEG-4 systems extradata to retrieve audio configuration.
Definition: mpeg4audio.c:81
DSP utils.
static void parse_bs_info(const uint32_t bs_info, unsigned int n, unsigned int div, unsigned int **div_blocks, unsigned int *num_blocks)
Parse the bs_info field to extract the block partitioning used in block switching mode...
Definition: alsdec.c:452
static int decode_block(ALSDecContext *ctx, ALSBlockData *bd)
Decode the block data.
Definition: alsdec.c:1001
Definition: libavcodec/avcodec.h:427
static int read_block(ALSDecContext *ctx, ALSBlockData *bd)
Read the block data.
Definition: alsdec.c:980
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
static int read_channel_data(ALSDecContext *ctx, ALSChannelData *cd, int c)
Read the channel data.
Definition: alsdec.c:1179
static void get_block_sizes(ALSDecContext *ctx, unsigned int *div_blocks, uint32_t *bs_info)
Read block switching field if necessary and set actual block sizes.
Definition: alsdec.c:513
static int revert_channel_correlation(ALSDecContext *ctx, ALSBlockData *bd, ALSChannelData **cd, int *reverted, unsigned int offset, int c)
Recursively reverts the inter-channel correlation for a block.
Definition: alsdec.c:1226
Generated on Mon Jun 30 2025 06:53:04 for FFmpeg by
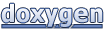