FFmpeg
|
xfacedec.c
Go to the documentation of this file.
static av_cold int xface_decode_init(AVCodecContext *avctx)
Definition: xfacedec.c:93
Definition: pixfmt.h:67
Definition: xface.h:81
static void decode_block(BigInt *b, char *bitmap, int w, int h, int level)
Definition: xfacedec.c:69
const ProbRange ff_xface_probranges_per_level[4][3]
Definition: xface.c:124
X-Face common definitions.
void ff_xface_generate_face(uint8_t *dst, uint8_t *const src)
Definition: xface.c:281
Definition: xface.h:57
void ff_big_mul(BigInt *b, uint8_t a)
Multiply a by b storing the result in b.
Definition: xface.c:90
static int xface_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: xfacedec.c:111
struct XFaceContext XFaceContext
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: xfacedec.c:89
external API header
void ff_big_div(BigInt *b, uint8_t a, uint8_t *r)
Divide b by a storing the result in b and the remainder in the word pointed to by r...
Definition: xface.c:51
Definition: libavcodec/avcodec.h:290
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
Definition: xface.h:86
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
common internal api header.
Y , 1bpp, 0 is white, 1 is black, in each byte pixels are ordered from the msb to the lsb...
Definition: pixfmt.h:77
Definition: xface.h:81
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Generated on Wed Jul 9 2025 06:53:41 for FFmpeg by
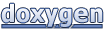