FFmpeg
|
libavcodec/flacdec.c
Go to the documentation of this file.
static unsigned int show_bits_long(GetBitContext *s, int n)
Show 0-32 bits.
Definition: get_bits.h:352
static int get_sr_golomb_flac(GetBitContext *gb, int k, int limit, int esc_len)
read signed golomb rice code (flac).
Definition: golomb.h:358
int av_samples_get_buffer_size(int *linesize, int nb_channels, int nb_samples, enum AVSampleFormat sample_fmt, int align)
Get the required buffer size for the given audio parameters.
Definition: samplefmt.c:125
void avpriv_flac_parse_block_header(const uint8_t *block_header, int *last, int *type, int *size)
Parse the metadata block parameters from the header.
Definition: flac.c:236
uint32_t av_crc(const AVCRC *ctx, uint32_t crc, const uint8_t *buffer, size_t length)
Calculate the CRC of a block.
Definition: crc.c:275
struct FLACContext FLACContext
av_cold void ff_flacdsp_init(FLACDSPContext *c, enum AVSampleFormat fmt, int bps)
Definition: flacdsp.c:88
int ff_flac_get_max_frame_size(int blocksize, int ch, int bps)
Calculate an estimate for the maximum frame size based on verbatim mode.
Definition: flac.c:148
Definition: libavcodec/flacdec.c:48
void(* lpc)(int32_t *samples, const int coeffs[32], int order, int qlevel, int len)
Definition: flacdsp.h:28
Definition: libavcodec/avcodec.h:393
int avpriv_flac_is_extradata_valid(AVCodecContext *avctx, enum FLACExtradataFormat *format, uint8_t **streaminfo_start)
Validate the FLAC extradata.
Definition: flac.c:169
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
static int decode_residuals(FLACContext *s, int32_t *decoded, int pred_order)
Definition: libavcodec/flacdec.c:205
Definition: flac.h:79
static int get_sbits_long(GetBitContext *s, int n)
Read 0-32 bits as a signed integer.
Definition: get_bits.h:344
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
int av_samples_fill_arrays(uint8_t **audio_data, int *linesize, const uint8_t *buf, int nb_channels, int nb_samples, enum AVSampleFormat sample_fmt, int align)
Fill plane data pointers and linesize for samples with sample format sample_fmt.
Definition: samplefmt.c:155
Definition: samplefmt.h:50
const AVCRC * av_crc_get_table(AVCRCId crc_id)
Get an initialized standard CRC table.
Definition: crc.c:261
Definition: flac.h:41
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
bitstream reader API header.
Definition: flac.h:83
FLAC (Free Lossless Audio Codec) decoder/demuxer common functions.
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static int decode_subframe_lpc(FLACContext *s, int32_t *decoded, int pred_order, int bps)
Definition: libavcodec/flacdec.c:300
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
Definition: flac.h:47
static int get_metadata_size(const uint8_t *buf, int buf_size)
Determine the size of an inline header.
Definition: libavcodec/flacdec.c:184
GetBitContext gb
GetBitContext initialized to start at the current frame.
Definition: libavcodec/flacdec.c:52
static void dump_headers(AVCodecContext *avctx, FLACStreaminfo *s)
Definition: libavcodec/flacdec.c:118
void avpriv_flac_parse_streaminfo(AVCodecContext *avctx, struct FLACStreaminfo *s, const uint8_t *buffer)
Parse the Streaminfo metadata block.
Definition: flac.c:204
#define FLACSTREAMINFO
Data needed from the Streaminfo header for use by the raw FLAC demuxer and/or the FLAC decoder...
Definition: flac.h:72
int ff_flac_decode_frame_header(AVCodecContext *avctx, GetBitContext *gb, FLACFrameInfo *fi, int log_level_offset)
Validate and decode a frame header.
Definition: flac.c:50
external API header
static int decode_subframe_fixed(FLACContext *s, int32_t *decoded, int pred_order, int bps)
Definition: libavcodec/flacdec.c:249
audio channel layout utility functions
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
Definition: flac.h:43
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
int sample_shift
shift required to make output samples 16-bit or 32-bit
Definition: libavcodec/flacdec.c:55
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Return number of bytes per sample.
Definition: samplefmt.c:104
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
void ff_flac_set_channel_layout(AVCodecContext *avctx)
Definition: flac.c:196
int av_sample_fmt_is_planar(enum AVSampleFormat sample_fmt)
Check if the sample format is planar.
Definition: samplefmt.c:118
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
static int parse_streaminfo(FLACContext *s, const uint8_t *buf, int buf_size)
Parse the STREAMINFO from an inline header.
Definition: libavcodec/flacdec.c:154
void avpriv_report_missing_feature(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
static int decode_subframe(FLACContext *s, int channel)
Definition: libavcodec/flacdec.c:336
Definition: get_bits.h:54
common internal api header.
Definition: crc.h:32
Definition: flacdsp.h:25
static av_cold int flac_decode_init(AVCodecContext *avctx)
Definition: libavcodec/flacdec.c:90
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Definition: flac.h:42
Definition: flac.h:51
exp golomb vlc stuff
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
void(* decorrelate[4])(uint8_t **out, int32_t **in, int channels, int len, int shift)
Definition: flacdsp.h:26
static av_cold int flac_decode_close(AVCodecContext *avctx)
Definition: libavcodec/flacdec.c:569
static int flac_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: libavcodec/flacdec.c:488
Generated on Wed May 7 2025 06:52:41 for FFmpeg by
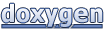