FFmpeg
|
wmv2enc.c
Go to the documentation of this file.
204 put_bits(&s->pb, ff_table_inter_intra[s->h263_aic_dir][1], ff_table_inter_intra[s->h263_aic_dir][0]);
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: pixfmt.h:67
Definition: put_bits.h:41
mpegvideo header.
int16_t * ff_h263_pred_motion(MpegEncContext *s, int block, int dir, int *px, int *py)
Definition: h263.c:315
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
Definition: wmv2.h:35
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
int no_rounding
apply no rounding to motion compensation (MPEG4, msmpeg4, ...) for b-frames rounding mode is always 0...
Definition: mpegvideo.h:439
MSMPEG4 data tables.
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
Definition: libavcodec/avcodec.h:121
void ff_msmpeg4_encode_motion(MpegEncContext *s, int mx, int my)
Definition: msmpeg4enc.c:287
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
struct Wmv2Context Wmv2Context
void ff_msmpeg4_handle_slices(MpegEncContext *s)
Definition: msmpeg4enc.c:325
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
const uint32_t(*const [WMV2_INTER_CBP_TABLE_COUNT] ff_wmv2_inter_table)[2]
Definition: msmpeg4data.c:1986
Definition: avutil.h:143
int ff_msmpeg4_coded_block_pred(MpegEncContext *s, int n, uint8_t **coded_block_ptr)
Definition: msmpeg4.c:153
void ff_wmv2_encode_mb(MpegEncContext *s, int16_t block[6][64], int motion_x, int motion_y)
Definition: wmv2enc.c:149
int ff_MPV_encode_picture(AVCodecContext *avctx, AVPacket *pkt, AVFrame *frame, int *got_packet)
Definition: mpegvideo_enc.c:1443
void ff_msmpeg4_encode_block(MpegEncContext *s, int16_t *block, int n)
Definition: msmpeg4enc.c:573
int ff_wmv2_encode_picture_header(MpegEncContext *s, int picture_number)
Definition: wmv2enc.c:69
Generated on Wed Apr 16 2025 06:53:23 for FFmpeg by
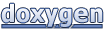