FFmpeg
|
evrcdec.c
Go to the documentation of this file.
Data tables for the EVRC decoder.
static evrc_packet_rate determine_bitrate(AVCodecContext *avctx, int *buf_size, const uint8_t **buf)
Determine the bitrate from the frame size and/or the first byte of the frame.
Definition: evrcdec.c:183
void ff_weighted_vector_sumf(float *out, const float *in_a, const float *in_b, float weight_coeff_a, float weight_coeff_b, int length)
float implementation of weighted sum of two vectors.
Definition: acelp_vectors.c:192
Definition: evrcdec.c:49
static void fcb_excitation(EVRCContext *e, const uint16_t *codebook, float *excitation, float pitch_gain, int pitch_lag, int subframe_size)
Definition: evrcdec.c:471
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
FFT size for synthesis(even) H
static av_cold int evrc_decode_init(AVCodecContext *avctx)
Initialize the speech codec according to the specification.
Definition: evrcdec.c:226
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
static evrc_packet_rate buf_size2bitrate(const int buf_size)
Definition: evrcdec.c:160
static void interpolate_lsp(float *ilsp, const float *lsp, const float *prev, int index)
Definition: evrcdec.c:310
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
static const uint8_t *const evrc_lspq_codebooks_row_sizes[]
Definition: evrcdata.h:1488
static const float evrc_energy_quant[][3]
Rate 1/8 frame energy quantization.
Definition: evrcdata.h:38
bitstream reader API header.
Definition: evrcdec.c:48
static void acb_excitation(EVRCContext *e, float *excitation, float gain, const float delay[3], int length)
Definition: evrcdec.c:399
float postfilter_residual[ACB_SIZE+SUBFRAME_SIZE]
Definition: evrcdec.c:79
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static void unpack_frame(EVRCContext *e)
Frame unpacking for RATE_FULL, RATE_HALF and RATE_QUANT.
Definition: evrcdec.c:103
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several the filter must be ready for frames arriving randomly on any input any filter with several inputs will most likely require some kind of queuing mechanism It is perfectly acceptable to have a limited queue and to drop frames when the inputs are too unbalanced request_frame This method is called when a frame is wanted on an output For an input
Definition: filter_design.txt:216
Definition: avutil.h:144
Definition: evrcdec.c:548
Definition: evrcdec.c:68
static int evrc_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: evrcdec.c:737
external API header
struct EVRCContext EVRCContext
static void postfilter(EVRCContext *e, float *in, const float *coeff, float *out, int idx, const struct PfCoeff *pfc, int length)
Definition: evrcdec.c:566
static void bandwidth_expansion(float *coeff, const float *inbuf, float gamma)
Definition: evrcdec.c:515
static void decode_8_pulses_35bits(const uint16_t *fixed_index, float *cod)
Definition: evrcdec.c:426
Definition: evrcdec.c:45
1i.*Xphase exp()
Definition: evrcdec.c:50
static const struct PfCoeff postfilter_coeffs[5]
static void decode_3_pulses_10bits(uint16_t fixed_index, float *cod)
Definition: evrcdec.c:451
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
Definition: evrcdec.c:46
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
void ff_acelp_lsf2lspd(double *lsp, const float *lsf, int lp_order)
Floating point version of ff_acelp_lsf2lsp()
Definition: lsp.c:93
static void synthesis_filter(const float *in, const float *filter_coeffs, float *memory, int buffer_length, float *samples)
Synthesis of the decoder output signal.
Definition: evrcdec.c:499
Definition: libavcodec/avcodec.h:451
static void decode_predictor_coeffs(const float *ilspf, float *ilpc)
Definition: evrcdec.c:341
static void warn_insufficient_frame_quality(AVCodecContext *avctx, const char *message)
Definition: evrcdec.c:214
Definition: get_bits.h:54
common internal api header.
these buffered frames must be flushed immediately if a new input produces new output(Example:frame rate-doubling filter:filter_frame must(1) flush the second copy of the previous frame, if it is still there,(2) push the first copy of the incoming frame,(3) keep the second copy for later.) If the input frame is not enough to produce output
static int decode_lspf(EVRCContext *e)
Decode the 10 vector quantized line spectral pair frequencies from the LSP transmission codes of any ...
Definition: evrcdec.c:277
Definition: evrcdec.c:47
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
static void residual_filter(float *output, const float *input, const float *coef, float *memory, int length)
Definition: evrcdec.c:526
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static void interpolate_delay(float *dst, float current, float prev, int index)
Definition: evrcdec.c:324
Generated on Fri May 30 2025 06:53:54 for FFmpeg by
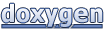