FFmpeg
|
mpc7.c
Go to the documentation of this file.
300 av_log(avctx, AV_LOG_ERROR, "Error decoding frame: used %i of %i bits\n", bits_used, bits_avail);
void ff_mpc_dequantize_and_synth(MPCContext *c, int maxband, int16_t **out, int channels)
Definition: libavcodec/mpc.c:61
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
static const uint16_t mpc7_quant_vlc[MPC7_QUANT_VLC_TABLES][2][64 *2]
Definition: mpc7data.h:62
Definition: libavcodec/avcodec.h:409
void av_fast_padded_malloc(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_malloc but the buffer has additional FF_INPUT_BUFFER_PADDING_SIZE at the end w...
Definition: libavcodec/utils.c:101
static const uint8_t mpc7_quant_vlc_sizes[MPC7_QUANT_VLC_TABLES *2]
Definition: mpc7data.h:54
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static int mpc7_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: mpc7.c:193
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: samplefmt.h:50
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
bitstream reader API header.
static const struct endianess table[]
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: avutil.h:144
static const uint8_t mpc7_quant_vlc_off[MPC7_QUANT_VLC_TABLES]
Definition: mpc7data.h:58
external API header
Definition: get_bits.h:63
common internal API header
audio channel layout utility functions
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
Musepack decoder MPEG Audio Layer 1/2 -like codec with frames of 1152 samples divided into 32 subband...
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
static void idx_to_quant(MPCContext *c, GetBitContext *gb, int idx, int *dst)
Fill samples for given subband.
Definition: mpc7.c:143
#define init_vlc(vlc, nb_bits, nb_codes,bits, bits_wrap, bits_size,codes, codes_wrap, codes_size,flags)
Definition: get_bits.h:426
static unsigned int av_lfg_get(AVLFG *c)
Get the next random unsigned 32-bit number using an ALFG.
Definition: lfg.h:38
void(* bswap_buf)(uint32_t *dst, const uint32_t *src, int w)
Definition: dsputil.h:208
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: get_bits.h:54
common internal api header.
DSP utils.
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
struct MPCContext MPCContext
Definition: mpc.h:52
Generated on Thu May 29 2025 06:52:50 for FFmpeg by
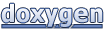