FFmpeg
|
apedec.c
Go to the documentation of this file.
97 int16_t *adaptcoeffs; ///< adaptive filter coefficients used for correcting of actual filter coefficients
1551 { "max_samples", "maximum number of samples decoded per call", OFFSET(blocks_per_loop), AV_OPT_TYPE_INT, { .i64 = 4608 }, 1, INT_MAX, PAR, "max_samples" },
1552 { "all", "no maximum. decode all samples for each packet at once", 0, AV_OPT_TYPE_CONST, { .i64 = INT_MAX }, INT_MIN, INT_MAX, PAR, "max_samples" },
static void decode_array_0000(APEContext *ctx, GetBitContext *gb, int32_t *out, APERice *rice, int blockstodecode)
Definition: apedec.c:587
static av_always_inline int filter_3800(APEPredictor *p, const int decoded, const int filter, const int delayA, const int delayB, const int start, const int shift)
Definition: apedec.c:843
Definition: start.py:1
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
static void apply_filter(APEContext *ctx, APEFilter *f, int32_t *data0, int32_t *data1, int count, int order, int fracbits)
Definition: apedec.c:1310
static void entropy_decode_stereo_0000(APEContext *ctx, int blockstodecode)
Definition: apedec.c:637
void(* entropy_decode_mono)(struct APEContext *ctx, int blockstodecode)
Definition: apedec.c:170
Definition: opt.h:222
void(* entropy_decode_stereo)(struct APEContext *ctx, int blockstodecode)
Definition: apedec.c:171
static int APESIGN(int32_t x)
Get inverse sign of integer (-1 for positive, 1 for negative and 0 for zero)
Definition: apedec.c:813
static void entropy_decode_stereo_3900(APEContext *ctx, int blockstodecode)
Definition: apedec.c:673
static void entropy_decode_stereo_3930(APEContext *ctx, int blockstodecode)
Definition: apedec.c:689
static av_always_inline int predictor_update_3930(APEPredictor *p, const int decoded, const int filter, const int delayA)
Definition: apedec.c:1035
static void predictor_decode_mono_3800(APEContext *ctx, int count)
Definition: apedec.c:989
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: apedec.c:68
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
static int ape_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: apedec.c:1395
Definition: opt.h:229
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: samplefmt.h:50
AVOptions.
static void do_init_filter(APEFilter *f, int16_t *buf, int order)
Definition: apedec.c:1239
static void entropy_decode_stereo_3860(APEContext *ctx, int blockstodecode)
Definition: apedec.c:653
static void entropy_decode_mono_3990(APEContext *ctx, int blockstodecode)
Definition: apedec.c:700
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
static const uint8_t ape_filter_fracbits[5][APE_FILTER_LEVELS]
Filter fraction bits depending on compression level.
Definition: apedec.c:85
Definition: apedec.c:65
static void ape_apply_filters(APEContext *ctx, int32_t *decoded0, int32_t *decoded1, int count)
Definition: apedec.c:1319
bitstream reader API header.
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
static const uint16_t counts_3970[22]
Fixed probabilities for symbols in Monkey Audio version 3.97.
Definition: apedec.c:390
static const uint16_t counts_diff_3980[21]
Probability ranges for symbols in Monkey Audio version 3.98.
Definition: apedec.c:417
void(* predictor_decode_mono)(struct APEContext *ctx, int count)
Definition: apedec.c:172
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
static int ape_decode_value_3900(APEContext *ctx, APERice *rice)
Definition: apedec.c:508
int32_t historybuffer[HISTORY_SIZE+PREDICTOR_SIZE]
Definition: apedec.c:127
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
static int range_decode_culshift(APEContext *ctx, int shift)
Decode value with given size in bits.
Definition: apedec.c:356
Definition: apedec.c:104
external API header
static int range_decode_bits(APEContext *ctx, int n)
Decode n bits (n <= 16) without modelling.
Definition: apedec.c:377
audio channel layout utility functions
static void predictor_decode_mono_3930(APEContext *ctx, int count)
Definition: apedec.c:1093
static const uint16_t ape_filter_orders[5][APE_FILTER_LEVELS]
Filter orders depending on compression level.
Definition: apedec.c:76
Definition: apedec.c:69
int32_t(* scalarproduct_and_madd_int16)(int16_t *v1, const int16_t *v2, const int16_t *v3, int len, int mul)
Calculate scalar product of v1 and v2, and v1[i] += v3[i] * mul.
Definition: dsputil.h:281
static void long_filter_high_3800(int32_t *buffer, int order, int shift, int32_t *coeffs, int32_t *delay, int length)
Definition: apedec.c:888
static av_always_inline int filter_fast_3320(APEPredictor *p, const int decoded, const int filter, const int delayA)
Definition: apedec.c:817
static int range_decode_culfreq(APEContext *ctx, int tot_f)
Calculate culmulative frequency for next symbol.
Definition: apedec.c:344
static void predictor_decode_stereo_3930(APEContext *ctx, int count)
Definition: apedec.c:1065
static void entropy_decode_stereo_3990(APEContext *ctx, int blockstodecode)
Definition: apedec.c:708
Definition: apedec.c:67
static int ape_decode_value_3860(APEContext *ctx, GetBitContext *gb, APERice *rice)
Definition: apedec.c:476
static void predictor_decode_stereo_3950(APEContext *ctx, int count)
Definition: apedec.c:1162
static void predictor_decode_stereo_3800(APEContext *ctx, int count)
Definition: apedec.c:933
static void init_filter(APEContext *ctx, APEFilter *f, int16_t *buf, int order)
Definition: apedec.c:1251
static int ape_decode_value_3990(APEContext *ctx, APERice *rice)
Definition: apedec.c:541
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
static const uint16_t counts_3980[22]
Fixed probabilities for symbols in Monkey Audio version 3.98.
Definition: apedec.c:408
static int range_get_symbol(APEContext *ctx, const uint16_t counts[], const uint16_t counts_diff[])
Decode symbol.
Definition: apedec.c:429
void(* bswap_buf)(uint32_t *dst, const uint32_t *src, int w)
Definition: dsputil.h:208
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
void(* predictor_decode_stereo)(struct APEContext *ctx, int count)
Definition: apedec.c:173
static void range_decode_update(APEContext *ctx, int sy_f, int lt_f)
Update decoding state.
Definition: apedec.c:370
static void entropy_decode_mono_3900(APEContext *ctx, int blockstodecode)
Definition: apedec.c:665
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static void do_apply_filter(APEContext *ctx, int version, APEFilter *f, int32_t *data, int count, int order, int fracbits)
Definition: apedec.c:1257
struct APERangecoder APERangecoder
static const uint16_t counts_diff_3970[21]
Probability ranges for symbols in Monkey Audio version 3.97.
Definition: apedec.c:399
#define CODEC_CAP_SUBFRAMES
Codec can output multiple frames per AVPacket Normally demuxers return one frame at a time...
Definition: libavcodec/avcodec.h:791
Definition: get_bits.h:54
common internal api header.
static int get_unary(GetBitContext *gb, int stop, int len)
Get unary code of limited length.
Definition: unary.h:33
static av_always_inline int predictor_update_filter(APEPredictor *p, const int decoded, const int filter, const int delayA, const int delayB, const int adaptA, const int adaptB)
Definition: apedec.c:1115
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
DSP utils.
Definition: apedec.c:109
static void long_filter_ehigh_3830(int32_t *buffer, int length)
Definition: apedec.c:911
static void predictor_decode_mono_3950(APEContext *ctx, int count)
Definition: apedec.c:1191
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Definition: apedec.c:66
struct APERice APERice
int16_t * adaptcoeffs
adaptive filter coefficients used for correcting of actual filter coefficients
Definition: apedec.c:97
Definition: libavcodec/avcodec.h:414
static void entropy_decode_mono_0000(APEContext *ctx, int blockstodecode)
Definition: apedec.c:631
static void entropy_decode_mono_3860(APEContext *ctx, int blockstodecode)
Definition: apedec.c:645
Generated on Thu May 29 2025 06:52:44 for FFmpeg by
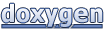