FFmpeg
|
libavcodec/h261dec.c
Go to the documentation of this file.
static int get_consumed_bytes(MpegEncContext *s, int buf_size)
returns the number of bytes consumed for building the current frame
Definition: libavcodec/h261dec.c:551
int coded_width
Bitstream width / height, may be different from width/height e.g.
Definition: libavcodec/avcodec.h:1312
av_cold int ff_MPV_common_init(MpegEncContext *s)
init common structure for both encoder and decoder.
Definition: mpegvideo.c:993
Definition: parser.h:28
void avcodec_set_dimensions(AVCodecContext *s, int width, int height)
Definition: libavcodec/utils.c:177
mpegvideo header.
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
static int h261_decode_picture_header(H261Context *h)
Decode the H.261 picture header.
Definition: libavcodec/h261dec.c:454
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
Definition: mpegvideo.h:52
#define INIT_VLC_STATIC(vlc, bits, a, b, c, d, e, f, g, static_size)
Definition: get_bits.h:445
static int h261_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: libavcodec/h261dec.c:562
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
static int h261_decode_block(H261Context *h, int16_t *block, int n, int coded)
Decode a macroblock.
Definition: libavcodec/h261dec.c:255
static int ff_h261_resync(H261Context *h)
Decode the group of blocks / video packet header.
Definition: libavcodec/h261dec.c:151
static av_cold void h261_decode_init_vlc(H261Context *h)
Definition: libavcodec/h261dec.c:47
static void ff_update_block_index(MpegEncContext *s)
Definition: mpegvideo.h:861
void ff_set_qscale(MpegEncContext *s, int qscale)
set qscale and update qscale dependent variables.
Definition: mpegvideo.c:3300
Definition: libavcodec/avcodec.h:106
static av_cold int h261_decode_init(AVCodecContext *avctx)
Definition: libavcodec/h261dec.c:69
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
simple assert() macros that are a bit more flexible than ISO C assert().
external API header
Definition: get_bits.h:63
void ff_mpeg_er_frame_start(MpegEncContext *s)
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
static av_cold int h261_decode_end(AVCodecContext *avctx)
Definition: libavcodec/h261dec.c:653
int ff_MPV_frame_start(MpegEncContext *s, AVCodecContext *avctx)
generic function for encode/decode called after coding/decoding the header and before a frame is code...
Definition: mpegvideo.c:1493
static int h261_decode_gob_header(H261Context *h)
Decode the group of blocks header or slice header.
Definition: libavcodec/h261dec.c:97
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
void ff_print_debug_info(MpegEncContext *s, Picture *p, AVFrame *pict)
Definition: mpegvideo.c:2164
void ff_MPV_decode_mb(MpegEncContext *s, int16_t block[12][64])
Definition: mpegvideo.c:2907
h261codec.
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
int mv[2][4][2]
motion vectors for a macroblock first coordinate : 0 = forward 1 = backward second " : depend...
Definition: mpegvideo.h:431
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
Definition: get_bits.h:54
int mb_stride
mb_width+1 used for some arrays to allow simple addressing of left & top MBs without sig11 ...
Definition: mpegvideo.h:278
static int decode_mv_component(GetBitContext *gb, int v)
Definition: libavcodec/h261dec.c:229
static int h261_decode_mb_skipped(H261Context *h, int mba1, int mba2)
Decode skipped macroblocks.
Definition: libavcodec/h261dec.c:192
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
int ff_find_unused_picture(MpegEncContext *s, int shared)
Definition: mpegvideo.c:1453
void ff_MPV_decode_defaults(MpegEncContext *s)
Set the given MpegEncContext to defaults for decoding.
Definition: mpegvideo.c:826
Generated on Tue Jan 21 2025 06:52:22 for FFmpeg by
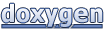