FFmpeg
|
libavcodec/bink.c
Go to the documentation of this file.
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
#define C
#define CHECK_READ_VAL(gb, b, t)
common check before starting decoding bundle data
Definition: libavcodec/bink.c:305
misc image utilities
static void binkb_init_bundle(BinkContext *c, int bundle_num)
Definition: libavcodec/bink.c:531
void(* scale_block)(const uint8_t src[64], uint8_t *dst, int linesize)
Definition: binkdsp.h:35
static int read_residue(GetBitContext *gb, int16_t block[64], int masks_count)
Read 8x8 block with residue after motion compensation.
Definition: libavcodec/bink.c:710
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
Definition: libavcodec/bink.c:113
static const uint8_t bink_scan[64]
Bink DCT and residue 8x8 block scan order.
Definition: binkdata.h:28
Tree col_high[16]
trees for decoding high nibble in "colours" data type
Definition: libavcodec/bink.c:124
16x16 block types (a subset of 8x8 block types)
Definition: libavcodec/bink.c:75
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
void(* idct_put)(uint8_t *dest, int line_size, int32_t *block)
Definition: binkdsp.h:33
void(* add_pixels8)(uint8_t *pixels, int16_t *block, int line_size)
Definition: dsputil.h:134
static int read_patterns(AVCodecContext *avctx, GetBitContext *gb, Bundle *b)
Definition: libavcodec/bink.c:405
planar YUV 4:2:0, 20bpp, (1 Cr & Cb sample per 2x2 Y & A samples)
Definition: pixfmt.h:105
static int binkb_get_value(BinkContext *c, int bundle_num)
Definition: libavcodec/bink.c:579
static void put_pixels8x8_overlapped(uint8_t *dst, uint8_t *src, int stride)
Copy 8x8 block from source to destination, where src and dst may be overlapped.
Definition: libavcodec/bink.c:795
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
Definition: libavcodec/bink.c:84
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
Definition: binkdsp.h:32
bitstream reader API header.
static int read_runs(AVCodecContext *avctx, GetBitContext *gb, Bundle *b)
Definition: libavcodec/bink.c:314
Bink DSP routines.
static int read_dct_coeffs(GetBitContext *gb, int32_t block[64], const uint8_t *scan, const int32_t quant_matrices[16][64], int q)
Read 8x8 block of DCT coefficients.
Definition: libavcodec/bink.c:602
static const struct endianess table[]
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static av_cold void free_bundles(BinkContext *c)
Free memory used by bundles.
Definition: libavcodec/bink.c:200
int col_lastval
value of last decoded high nibble in "colours" data type
Definition: libavcodec/bink.c:125
external API header
Definition: get_bits.h:63
block is composed from runs of colours with custom scan order
Definition: libavcodec/bink.c:135
common internal API header
static av_cold void binkb_calc_quant(void)
Caclulate quantization tables for version b.
Definition: libavcodec/bink.c:1239
int av_image_check_size(unsigned int w, unsigned int h, int log_offset, void *log_ctx)
Check if the given dimension of an image is valid, meaning that all bytes of the image can be address...
Definition: imgutils.c:231
int ff_reget_buffer(AVCodecContext *avctx, AVFrame *frame)
Identical in function to av_frame_make_writable(), except it uses ff_get_buffer() to allocate the buf...
Definition: libavcodec/utils.c:868
static int binkb_decode_plane(BinkContext *c, AVFrame *frame, GetBitContext *gb, int plane_idx, int is_key, int is_chroma)
Definition: libavcodec/bink.c:805
void(* idct_add)(uint8_t *dest, int line_size, int32_t *block)
Definition: binkdsp.h:34
static int bink_decode_plane(BinkContext *c, AVFrame *frame, GetBitContext *gb, int plane_idx, int is_chroma)
Definition: libavcodec/bink.c:951
uint8_t * cur_dec
pointer to the not yet decoded part of the buffer
Definition: libavcodec/bink.c:106
Half-pel DSP functions.
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
#define DC_START_BITS
number of bits used to store first DC value in bundle
Definition: libavcodec/bink.c:464
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
#define init_vlc(vlc, nb_bits, nb_codes,bits, bits_wrap, bits_size,codes, codes_wrap, codes_size,flags)
Definition: get_bits.h:426
op_pixels_func put_pixels_tab[4][4]
Halfpel motion compensation with rounding (a+b+1)>>1.
Definition: hpeldsp.h:56
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
block is filled with two colours following custom pattern
Definition: libavcodec/bink.c:140
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
Definition: libavcodec/bink.c:56
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
AVFrame * av_frame_alloc(void)
Allocate an AVFrame and set its fields to default values.
Definition: frame.c:95
static void merge(GetBitContext *gb, uint8_t *dst, uint8_t *src, int size)
Merge two consequent lists of equal size depending on bits read.
Definition: libavcodec/bink.c:215
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
uint8_t * cur_ptr
pointer to the data that is not read from buffer yet
Definition: libavcodec/bink.c:107
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
Definition: get_bits.h:54
static int binkb_read_bundle(BinkContext *c, GetBitContext *gb, int bundle_num)
Definition: libavcodec/bink.c:545
common internal api header.
static void init_lengths(BinkContext *c, int width, int bw)
Initialize length length in all bundles.
Definition: libavcodec/bink.c:151
static av_cold int init_bundles(BinkContext *c)
Allocate memory for bundles.
Definition: libavcodec/bink.c:176
void av_frame_free(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: frame.c:108
static int read_colors(GetBitContext *gb, Bundle *b, BinkContext *c)
Definition: libavcodec/bink.c:425
static int read_block_types(AVCodecContext *avctx, GetBitContext *gb, Bundle *b)
Definition: libavcodec/bink.c:370
static void read_tree(GetBitContext *gb, Tree *tree)
Read information about Huffman tree used to decode data.
Definition: libavcodec/bink.c:242
static int read_dcs(AVCodecContext *avctx, GetBitContext *gb, Bundle *b, int start_bits, int has_sign)
Definition: libavcodec/bink.c:466
Definition: libavcodec/avcodec.h:238
static int decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *pkt)
Definition: libavcodec/bink.c:1180
DSP utils.
static int read_motion_values(AVCodecContext *avctx, GetBitContext *gb, Bundle *b)
Definition: libavcodec/bink.c:336
struct BinkContext BinkContext
static const int binkb_bundle_signed[BINKB_NB_SRC]
Definition: libavcodec/bink.c:63
Definition: avutil.h:143
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
static void read_bundle(GetBitContext *gb, BinkContext *c, int bundle_num)
Prepare bundle for decoding data.
Definition: libavcodec/bink.c:283
static int get_value(BinkContext *c, int bundle)
Retrieve next value from bundle.
Definition: libavcodec/bink.c:518
#define AV_GET_BUFFER_FLAG_REF
The decoder will keep a reference to the frame and may reuse it later.
Definition: libavcodec/avcodec.h:909
OldSources
IDs for different data types used in old version of Bink video codec.
Definition: libavcodec/bink.c:44
Generated on Thu May 29 2025 06:52:45 for FFmpeg by
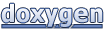