FFmpeg
|
alsa-audio-enc.c
Go to the documentation of this file.
Definition: libavcodec/avcodec.h:100
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
av_cold int ff_alsa_close(AVFormatContext *s1)
Close the ALSA PCM.
Definition: alsa-audio-common.c:299
int ff_alsa_extend_reorder_buf(AlsaData *s, int min_size)
Definition: alsa-audio-common.c:331
static void audio_get_output_timestamp(AVFormatContext *s1, int stream, int64_t *dts, int64_t *wall)
Definition: alsa-audio-enc.c:108
Main libavdevice API header.
Definition: alsa-audio.h:48
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
av_cold int ff_alsa_open(AVFormatContext *ctx, snd_pcm_stream_t mode, unsigned int *sample_rate, int channels, enum AVCodecID *codec_id)
Open an ALSA PCM.
Definition: alsa-audio-common.c:167
static av_cold int audio_write_header(AVFormatContext *s1)
Definition: alsa-audio-enc.c:47
int ff_alsa_xrun_recover(AVFormatContext *s1, int err)
Try to recover from ALSA buffer underrun.
Definition: alsa-audio-common.c:310
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
struct AlsaData AlsaData
static int audio_write_packet(AVFormatContext *s1, AVPacket *pkt)
Definition: alsa-audio-enc.c:75
#define AVFMT_NOFILE
Demuxer will use avio_open, no opened file should be provided by the caller.
Definition: avformat.h:345
Definition: avformat.h:377
ALSA input and output: definitions and structures.
Generated on Tue May 27 2025 07:04:01 for FFmpeg by
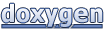