FFmpeg
|
lavfutils.c
Go to the documentation of this file.
Definition: frame.h:76
int avformat_open_input(AVFormatContext **ps, const char *filename, AVInputFormat *fmt, AVDictionary **options)
Definition: libavformat/utils.c:614
int av_image_alloc(uint8_t *pointers[4], int linesizes[4], int w, int h, enum AVPixelFormat pix_fmt, int align)
Definition: imgutils.c:190
Definition: avformat.h:456
Definition: libavcodec/avcodec.h:2854
Definition: avformat.h:944
int avcodec_decode_video2(AVCodecContext *avctx, AVFrame *picture, int *got_picture_ptr, const AVPacket *avpkt)
Definition: libavcodec/utils.c:1901
void av_image_copy(uint8_t *dst_data[4], int dst_linesizes[4], const uint8_t *src_data[4], const int src_linesizes[4], enum AVPixelFormat pix_fmt, int width, int height)
Definition: imgutils.c:257
AVInputFormat * av_find_input_format(const char *short_name)
Definition: libavformat/utils.c:248
int ff_load_image(uint8_t *data[4], int linesize[4], int *w, int *h, enum AVPixelFormat *pix_fmt, const char *filename, void *log_ctx)
Definition: lavfutils.c:24
Definition: libavcodec/avcodec.h:1134
AVCodec * avcodec_find_decoder(enum AVCodecID id)
Definition: libavcodec/utils.c:2397
int avcodec_open2(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
Definition: libavcodec/utils.c:1034
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int av_read_frame(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/utils.c:1530
void avformat_close_input(AVFormatContext **s)
Definition: libavformat/utils.c:3329
Definition: libavcodec/avcodec.h:1028
Generated on Wed Aug 6 2025 06:53:30 for FFmpeg by
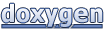