FFmpeg
|
h264idct_template.c
Go to the documentation of this file.
173 void FUNCC(ff_h264_idct_add16)(uint8_t *dst, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15*8]){
178 if(nnz==1 && ((dctcoef*)block)[i*16]) FUNCC(ff_h264_idct_dc_add)(dst + block_offset[i], block + i*16*sizeof(pixel), stride);
184 void FUNCC(ff_h264_idct_add16intra)(uint8_t *dst, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15*8]){
187 if(nnzc[ scan8[i] ]) FUNCC(ff_h264_idct_add )(dst + block_offset[i], block + i*16*sizeof(pixel), stride);
188 else if(((dctcoef*)block)[i*16]) FUNCC(ff_h264_idct_dc_add)(dst + block_offset[i], block + i*16*sizeof(pixel), stride);
192 void FUNCC(ff_h264_idct8_add4)(uint8_t *dst, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15*8]){
197 if(nnz==1 && ((dctcoef*)block)[i*16]) FUNCC(ff_h264_idct8_dc_add)(dst + block_offset[i], block + i*16*sizeof(pixel), stride);
203 void FUNCC(ff_h264_idct_add8)(uint8_t **dest, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15*8]){
215 void FUNCC(ff_h264_idct_add8_422)(uint8_t **dest, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15*8]){
void FUNCC() ff_h264_idct8_dc_add(uint8_t *_dst, int16_t *_block, int stride)
Definition: h264idct_template.c:158
void FUNCC() ff_h264_idct8_add(uint8_t *_dst, int16_t *_block, int stride)
Definition: h264idct_template.c:68
H.264 / AVC / MPEG4 part10 codec.
void FUNCC() ff_h264_idct_add16(uint8_t *dst, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15 *8])
Definition: h264idct_template.c:173
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several the filter must be ready for frames arriving randomly on any input any filter with several inputs will most likely require some kind of queuing mechanism It is perfectly acceptable to have a limited queue and to drop frames when the inputs are too unbalanced request_frame This method is called when a frame is wanted on an output For an input
Definition: filter_design.txt:216
void FUNCC() ff_h264_idct_add(uint8_t *_dst, int16_t *_block, int stride)
Definition: h264idct_template.c:32
void FUNCC() ff_h264_chroma_dc_dequant_idct(int16_t *_block, int qmul)
Definition: h264idct_template.c:303
void FUNCC() ff_h264_idct8_add4(uint8_t *dst, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15 *8])
Definition: h264idct_template.c:192
FIXME Range Coding of cr are mx and my are Motion Vector top and top right vectors is used as motion vector prediction the used motion vector is the sum of the predictor and(mvx_diff, mvy_diff)*mv_scale Intra DC Predicton block[y][x] dc[1]
Definition: snow.txt:392
void FUNCC() ff_h264_luma_dc_dequant_idct(int16_t *_output, int16_t *_input, int qmul)
IDCT transforms the 16 dc values and dequantizes them.
Definition: h264idct_template.c:241
void FUNCC() ff_h264_idct_dc_add(uint8_t *_dst, int16_t *_block, int stride)
Definition: h264idct_template.c:143
common internal and external API header
void FUNCC() ff_h264_chroma422_dc_dequant_idct(int16_t *_block, int qmul)
Definition: h264idct_template.c:276
void FUNCC() ff_h264_idct_add8_422(uint8_t **dest, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15 *8])
Definition: h264idct_template.c:215
these buffered frames must be flushed immediately if a new input produces new output(Example:frame rate-doubling filter:filter_frame must(1) flush the second copy of the previous frame, if it is still there,(2) push the first copy of the incoming frame,(3) keep the second copy for later.) If the input frame is not enough to produce output
void FUNCC() ff_h264_idct_add8(uint8_t **dest, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15 *8])
Definition: h264idct_template.c:203
#define stride
void FUNCC() ff_h264_idct_add16intra(uint8_t *dst, const int *block_offset, int16_t *block, int stride, const uint8_t nnzc[15 *8])
Definition: h264idct_template.c:184
Generated on Fri Aug 1 2025 06:53:41 for FFmpeg by
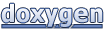