FFmpeg
|
vaapi_mpeg2.c
Go to the documentation of this file.
38 static int vaapi_mpeg2_start_frame(AVCodecContext *avctx, av_unused const uint8_t *buffer, av_unused uint32_t size)
65 pic_param->picture_coding_extension.bits.concealment_motion_vectors = s->concealment_motion_vectors;
101 static int vaapi_mpeg2_decode_slice(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
124 slice_param = (VASliceParameterBufferMPEG2 *)ff_vaapi_alloc_slice(avctx->hwaccel_context, buffer, size);
HW decoding through VA API, Picture.data[3] contains a vaapi_render_state struct which contains the b...
Definition: pixfmt.h:126
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
This structure is used to share data between the FFmpeg library and the client video application...
Definition: vaapi.h:50
static int mpeg2_get_f_code(MpegEncContext *s)
Reconstruct bitstream f_code.
Definition: vaapi_mpeg2.c:26
void * ff_vaapi_alloc_pic_param(struct vaapi_context *vactx, unsigned int size)
Allocate a new picture parameter buffer.
Definition: vaapi.c:133
static int vaapi_mpeg2_start_frame(AVCodecContext *avctx, av_unused const uint8_t *buffer, av_unused uint32_t size)
Definition: vaapi_mpeg2.c:38
VASliceParameterBufferBase * ff_vaapi_alloc_slice(struct vaapi_context *vactx, const uint8_t *buffer, uint32_t size)
Allocate a new slice descriptor for the input slice.
Definition: vaapi.c:148
static int vaapi_mpeg2_decode_slice(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
Definition: vaapi_mpeg2.c:101
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
static int mpeg2_get_is_frame_start(MpegEncContext *s)
Determine frame start: first field for field picture or frame picture.
Definition: vaapi_mpeg2.c:33
Definition: get_bits.h:54
void * ff_vaapi_alloc_iq_matrix(struct vaapi_context *vactx, unsigned int size)
Allocate a new IQ matrix buffer.
Definition: vaapi.c:138
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
static VASurfaceID ff_vaapi_get_surface_id(Picture *pic)
Extract VASurfaceID from a Picture.
Definition: vaapi_internal.h:39
Definition: avutil.h:143
Generated on Mon Nov 18 2024 06:52:02 for FFmpeg by
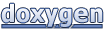