FFmpeg
|
vaapi_mpeg4.c
Go to the documentation of this file.
42 static int vaapi_mpeg4_start_frame(AVCodecContext *avctx, av_unused const uint8_t *buffer, av_unused uint32_t size)
82 pic_param->vop_fields.bits.backward_reference_vop_coding_type = s->pict_type == AV_PICTURE_TYPE_B ? s->next_picture.f.pict_type - AV_PICTURE_TYPE_I : 0;
118 static int vaapi_mpeg4_decode_slice(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
134 slice_param = (VASliceParameterBufferMPEG4 *)ff_vaapi_alloc_slice(avctx->hwaccel_context, buffer, size);
HW decoding through VA API, Picture.data[3] contains a vaapi_render_state struct which contains the b...
Definition: pixfmt.h:126
Definition: libavcodec/avcodec.h:115
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
This structure is used to share data between the FFmpeg library and the client video application...
Definition: vaapi.h:50
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
int intra_dc_threshold
QP above whch the ac VLC should be used for intra dc.
Definition: mpegvideo.h:595
static int mpeg4_get_intra_dc_vlc_thr(MpegEncContext *s)
Reconstruct bitstream intra_dc_vlc_thr.
Definition: vaapi_mpeg4.c:27
int no_rounding
apply no rounding to motion compensation (MPEG4, msmpeg4, ...) for b-frames rounding mode is always 0...
Definition: mpegvideo.h:439
static int vaapi_mpeg4_decode_slice(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
Definition: vaapi_mpeg4.c:118
void * ff_vaapi_alloc_pic_param(struct vaapi_context *vactx, unsigned int size)
Allocate a new picture parameter buffer.
Definition: vaapi.c:133
int ff_h263_get_gob_height(MpegEncContext *s)
Get the GOB height based on picture height.
Definition: h263.c:377
Definition: libavcodec/avcodec.h:107
VASliceParameterBufferBase * ff_vaapi_alloc_slice(struct vaapi_context *vactx, const uint8_t *buffer, uint32_t size)
Allocate a new slice descriptor for the input slice.
Definition: vaapi.c:148
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
static int vaapi_mpeg4_start_frame(AVCodecContext *avctx, av_unused const uint8_t *buffer, av_unused uint32_t size)
Definition: vaapi_mpeg4.c:42
void * ff_vaapi_alloc_iq_matrix(struct vaapi_context *vactx, unsigned int size)
Allocate a new IQ matrix buffer.
Definition: vaapi.c:138
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
static VASurfaceID ff_vaapi_get_surface_id(Picture *pic)
Extract VASurfaceID from a Picture.
Definition: vaapi_internal.h:39
Definition: avutil.h:143
Generated on Fri Aug 1 2025 06:53:48 for FFmpeg by
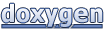