FFmpeg
|
mpeg12.h
Go to the documentation of this file.
72 int ff_mpeg1_find_frame_end(ParseContext *pc, const uint8_t *buf, int buf_size, AVCodecParserContext *s);
Definition: parser.h:28
mpegvideo header.
uint8_t ff_mpeg12_static_rl_table_store[2][2][2 *MAX_RUN+MAX_LEVEL+3]
Definition: mpeg12.c:48
Definition: get_bits.h:63
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
Definition: libavcodec/avcodec.h:3747
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
static int get_xbits(GetBitContext *s, int n)
read mpeg1 dc style vlc (sign bit + mantisse with no MSB).
Definition: get_bits.h:212
int ff_mpeg1_decode_block_intra(MpegEncContext *s, int16_t *block, int n)
Definition: mpeg12dec.c:158
Definition: get_bits.h:54
int ff_mpeg1_find_frame_end(ParseContext *pc, const uint8_t *buf, int buf_size, AVCodecParserContext *s)
Find the end of the current frame in the bitstream.
Definition: mpeg12.c:165
Generated on Fri May 30 2025 06:53:58 for FFmpeg by
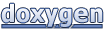