FFmpeg
|
mpeg12.c
Go to the documentation of this file.
165 int ff_mpeg1_find_frame_end(ParseContext *pc, const uint8_t *buf, int buf_size, AVCodecParserContext *s)
196 if (pc->frame_start_found == 0 && state >= SLICE_MIN_START_CODE && state <= SLICE_MAX_START_CODE) {
int last_mv[2][2][2]
last MV, used for MV prediction in MPEG1 & B-frame MPEG4
Definition: mpegvideo.h:433
Definition: parser.h:28
const unsigned char ff_mpeg12_vlc_dc_lum_bits[12]
Definition: mpeg12data.c:55
void ff_init_rl(RLTable *rl, uint8_t static_store[2][2 *MAX_RUN+MAX_LEVEL+3])
Definition: mpegvideo.c:1304
mpegvideo header.
Timecode helpers header.
#define INIT_VLC_STATIC(vlc, bits, a, b, c, d, e, f, g, static_size)
Definition: get_bits.h:445
const uint8_t * avpriv_find_start_code(const uint8_t *p, const uint8_t *end, uint32_t *state)
void ff_fetch_timestamp(AVCodecParserContext *s, int off, int remove)
Fetch timestamps for a specific byte within the current access unit.
Definition: parser.c:88
Multithreading support functions.
const uint16_t ff_mpeg12_vlc_dc_chroma_code[12]
Definition: mpeg12data.c:59
simple assert() macros that are a bit more flexible than ISO C assert().
external API header
Definition: get_bits.h:63
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
const unsigned char ff_mpeg12_vlc_dc_chroma_bits[12]
Definition: mpeg12data.c:62
Definition: libavcodec/avcodec.h:3747
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
MPEG1/2 tables.
MPEG1/2 decoder tables.
int ff_mpeg1_find_frame_end(ParseContext *pc, const uint8_t *buf, int buf_size, AVCodecParserContext *s)
Find the end of the current frame in the bitstream.
Definition: mpeg12.c:165
common internal api header.
uint8_t ff_mpeg12_static_rl_table_store[2][2][2 *MAX_RUN+MAX_LEVEL+3]
Definition: mpeg12.c:48
DSP utils.
const uint8_t ff_mpeg12_mbMotionVectorTable[17][2]
Definition: mpeg12data.c:288
Generated on Mon Nov 18 2024 06:51:57 for FFmpeg by
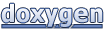