FFmpeg
|
amrnbdec.c
Go to the documentation of this file.
107 double prev_lsp_sub4[LP_FILTER_ORDER]; ///< lsp vector for the 4th subframe of the previous frame
116 float excitation_buf[PITCH_DELAY_MAX + LP_FILTER_ORDER + 1 + AMR_SUBFRAME_SIZE]; ///< current excitation and all necessary excitation history
120 float fixed_vector[AMR_SUBFRAME_SIZE]; ///< algebraic codebook (fixed) vector (must be kept zero between frames)
122 float prediction_error[4]; ///< quantified prediction errors {20log10(^gamma_gc)} for previous four subframes
130 float prev_sparse_fixed_gain; ///< previous fixed gain; used by anti-sparseness processing to determine "onset"
131 uint8_t prev_ir_filter_nr; ///< previous impulse response filter "impNr": 0 - strong, 1 - medium, 2 - none
239 static void interpolate_lsf(ACELPVContext *ctx, float lsf_q[4][LP_FILTER_ORDER], float *lsf_new)
346 lsf_q[i] = (lsf_r[i] + p->prev_lsf_r[i] * pred_fac[i]) * (LSF_R_FAC / 8000.0) + lsf_3_mean[i] * (1.0 / 8000.0);
960 float spare_vector[AMR_SUBFRAME_SIZE]; // extra stack space to hold result from anti-sparseness processing
Definition: libavcodec/avcodec.h:367
void ff_decode_pitch_lag(int *lag_int, int *lag_frac, int pitch_index, const int prev_lag_int, const int subframe, int third_as_first, int resolution)
Decode the adaptive codebook index to the integer and fractional parts of the pitch lag for one subfr...
Definition: acelp_pitch_delay.c:147
void ff_decode_10_pulses_35bits(const int16_t *fixed_index, AMRFixed *fixed_sparse, const uint8_t *gray_decode, int half_pulse_count, int bits)
Decode the algebraic codebook index to pulse positions and signs and construct the algebraic codebook...
Definition: acelp_vectors.c:151
static int amrnb_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: amrnbdec.c:948
static void pitch_sharpening(AMRContext *p, int subframe, enum Mode mode, AMRFixed *fixed_sparse)
Apply pitch lag to obtain the sharpened fixed vector (section 6.1.2)
Definition: amrnbdec.c:552
void(* acelp_interpolatef)(float *out, const float *in, const float *filter_coeffs, int precision, int frac_pos, int filter_length, int length)
Floating point version of ff_acelp_interpolate()
Definition: acelp_filters.h:32
float(* dot_productf)(const float *a, const float *b, int length)
Return the dot product.
Definition: celp_math.h:37
void ff_clear_fixed_vector(float *out, const AMRFixed *in, int size)
Clear array values set by set_fixed_vector.
Definition: acelp_vectors.c:251
static const uint8_t base_five_table[128][3]
Base-5 representation for values 0-124.
Definition: amrnbdata.h:367
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
static void decode_fixed_sparse(AMRFixed *fixed_sparse, const uint16_t *pulses, const enum Mode mode, const int subframe)
Decode the algebraic codebook index to pulse positions and signs, then construct the algebraic codebo...
Definition: amrnbdec.c:499
static const uint8_t track_position[16]
track start positions for algebraic code book routines
Definition: amrnbdata.h:1433
void ff_set_fixed_vector(float *out, const AMRFixed *in, float scale, int size)
Add fixed vector to an array from a sparse representation.
Definition: acelp_vectors.c:234
static float fixed_gain_smooth(AMRContext *p, const float *lsf, const float *lsf_avg, const enum Mode mode)
fixed gain smoothing Note that where the spec specifies the "spectrum in the q domain" in section 6...
Definition: amrnbdec.c:588
static int synthesis(AMRContext *p, float *lpc, float fixed_gain, const float *fixed_vector, float *samples, uint8_t overflow)
Conduct 10th order linear predictive coding synthesis.
Definition: amrnbdec.c:790
static void weighted_vector_sumd(double *out, const double *in_a, const double *in_b, double weight_coeff_a, double weight_coeff_b, int length)
Double version of ff_weighted_vector_sumf()
Definition: amrnbdec.c:149
static av_cold int amrnb_decode_init(AVCodecContext *avctx)
Definition: amrnbdec.c:160
double prev_lsp_sub4[LP_FILTER_ORDER]
lsp vector for the 4th subframe of the previous frame
Definition: amrnbdec.c:107
static void postfilter(AMRContext *p, float *lpc, float *buf_out)
Perform adaptive post-filtering to enhance the quality of the speech.
Definition: amrnbdec.c:904
void(* celp_lp_zero_synthesis_filterf)(float *out, const float *filter_coeffs, const float *in, int buffer_length, int filter_length)
LP zero synthesis filter.
Definition: celp_filters.h:65
Sparse representation for the algebraic codebook (fixed) vector.
Definition: acelp_vectors.h:53
Definition: samplefmt.h:50
Definition: amrnbdec.c:100
static const uint16_t qua_gain_code[32]
scalar quantized fixed gain table for 7.95 and 12.2 kbps modes
Definition: amrnbdata.h:1450
Definition: acelp_vectors.h:28
Definition: acelp_filters.h:28
static const uint16_t qua_gain_pit[16]
scalar quantized pitch gain table for 7.95 and 12.2 kbps modes
Definition: amrnbdata.h:1444
static void lsf2lsp_3(AMRContext *p)
Decode a set of 3 split-matrix quantized lsf indexes into an lsp vector.
Definition: amrnbdec.c:327
static const float energy_pred_fac[4]
4-tap moving average prediction coefficients in reverse order
Definition: amrnbdata.h:1463
uint16_t fixed_gain
index to decode the fixed gain factor, for MODE_12k2 and MODE_7k95
Definition: amrnbdata.h:61
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
static const int8_t lsp_sub4_init[LP_FILTER_ORDER]
Values for the lsp vector from the 4th subframe of the previous subframe values.
Definition: amrnbdata.h:395
static void apply_ir_filter(float *out, const AMRFixed *in, const float *filter)
Circularly convolve a sparse fixed vector with a phase dispersion impulse response filter (D...
Definition: amrnbdec.c:672
AMRNBFrame frame
decoded AMR parameters (lsf coefficients, codebook indexes, etc)
Definition: amrnbdec.c:101
void ff_adaptive_gain_control(float *out, const float *in, float speech_energ, int size, float alpha, float *gain_mem)
Adaptive gain control (as used in AMR postfiltering)
Definition: acelp_vectors.c:202
static void ff_amr_bit_reorder(uint16_t *out, int size, const uint8_t *data, const R_TABLE_TYPE *ord_table)
Fill the frame structure variables from bitstream by parsing the given reordering table that uses the...
Definition: amr.h:51
uint16_t lsf[5]
lsf parameters: 5 parameters for MODE_12k2, only 3 for other modes
Definition: amrnbdata.h:69
void(* weighted_vector_sumf)(float *out, const float *in_a, const float *in_b, float weight_coeff_a, float weight_coeff_b, int length)
float implementation of weighted sum of two vectors.
Definition: acelp_vectors.h:40
uint8_t prev_ir_filter_nr
previous impulse response filter "impNr": 0 - strong, 1 - medium, 2 - none
Definition: amrnbdec.c:131
static void decode_pitch_vector(AMRContext *p, const AMRNBSubframe *amr_subframe, const int subframe)
Definition: amrnbdec.c:391
static void update_state(AMRContext *p)
Update buffers and history at the end of decoding a subframe.
Definition: amrnbdec.c:847
float fixed_vector[AMR_SUBFRAME_SIZE]
algebraic codebook (fixed) vector (must be kept zero between frames)
Definition: amrnbdec.c:120
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
void(* celp_lp_synthesis_filterf)(float *out, const float *filter_coeffs, const float *in, int buffer_length, int filter_length)
LP synthesis filter.
Definition: celp_filters.h:45
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
int16_t prev_lsf_r[LP_FILTER_ORDER]
residual LSF vector from previous subframe
Definition: amrnbdec.c:105
static const uint8_t frame_sizes_nb[N_MODES]
number of bytes for each mode
Definition: amrnbdata.h:357
void ff_scale_vector_to_given_sum_of_squares(float *out, const float *in, float sum_of_squares, const int n)
Set the sum of squares of a signal by scaling.
Definition: acelp_vectors.c:223
float pitch_gain[5]
quantified pitch gains for the current and previous four subframes
Definition: amrnbdec.c:123
external API header
float * excitation
pointer to the current excitation vector in excitation_buf
Definition: amrnbdec.c:117
static void interpolate_lsf(ACELPVContext *ctx, float lsf_q[4][LP_FILTER_ORDER], float *lsf_new)
Interpolate the LSF vector (used for fixed gain smoothing).
Definition: amrnbdec.c:239
Definition: celp_filters.h:28
static const float *const ir_filters_lookup_MODE_7k95[2]
Definition: amrnbdata.h:1661
audio channel layout utility functions
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
Definition: celp_math.h:28
float samples_in[LP_FILTER_ORDER+AMR_SUBFRAME_SIZE]
floating point samples
Definition: amrnbdec.c:139
static const uint16_t gains_MODE_4k75[512][2]
gain table for 4.75 kbps mode
Definition: amrnbdata.h:1469
void(* acelp_apply_order_2_transfer_function)(float *out, const float *in, const float zero_coeffs[2], const float pole_coeffs[2], float gain, float mem[2], int n)
Apply an order 2 rational transfer function in-place.
Definition: acelp_filters.h:47
void ff_tilt_compensation(float *mem, float tilt, float *samples, int size)
Apply tilt compensation filter, 1 - tilt * z-1.
Definition: acelp_filters.c:136
void ff_acelp_lspd2lpc(const double *lsp, float *lpc, int lp_half_order)
Reconstruct LPC coefficients from the line spectral pair frequencies.
Definition: lsp.c:209
static const float * anti_sparseness(AMRContext *p, AMRFixed *fixed_sparse, const float *fixed_vector, float fixed_gain, float *out)
Reduce fixed vector sparseness by smoothing with one of three IR filters.
Definition: amrnbdec.c:719
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
float lsf_q[4][LP_FILTER_ORDER]
Interpolated LSF vector for fixed gain smoothing.
Definition: amrnbdec.c:109
void ff_celp_circ_addf(float *out, const float *in, const float *lagged, int lag, float fac, int n)
Add an array to a rotated array.
Definition: celp_filters.c:50
float high_pass_mem[2]
previous intermediate values in the high-pass filter
Definition: amrnbdec.c:137
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
uint8_t diff_count
the number of subframes for which diff has been above 0.65
Definition: amrnbdec.c:127
static const uint8_t *const amr_unpacking_bitmaps_per_mode[N_MODES]
position of the bitmapping data for each packet type in the AMRNBFrame
Definition: amrnbdata.h:345
float prediction_error[4]
quantified prediction errors {20log10(^gamma_gc)} for previous four subframes
Definition: amrnbdec.c:122
static float tilt_factor(AMRContext *p, float *lpc_n, float *lpc_d)
Get the tilt factor of a formant filter from its transfer function.
Definition: amrnbdec.c:874
void ff_acelp_lsf2lspd(double *lsp, const float *lsf, int lp_order)
Floating point version of ff_acelp_lsf2lsp()
Definition: lsp.c:93
float fixed_gain[5]
quantified fixed gains for the current and previous four subframes
Definition: amrnbdec.c:124
#define AMR_TILT_RESPONSE
Number of impulse response coefficients used for tilt factor.
Definition: amrnbdec.c:94
void ff_acelp_vectors_init(ACELPVContext *c)
Initialize ACELPVContext.
Definition: acelp_vectors.c:266
static void decode_8_pulses_31bits(const int16_t *fixed_index, AMRFixed *fixed_sparse)
Decode the algebraic codebook index to pulse positions and signs and construct the algebraic codebook...
Definition: amrnbdec.c:453
static void decode_pitch_lag_1_6(int *lag_int, int *lag_frac, int pitch_index, const int prev_lag_int, const int subframe)
Like ff_decode_pitch_lag(), but with 1/6 resolution.
Definition: amrnbdec.c:372
void avpriv_report_missing_feature(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
static void decode_10bit_pulse(int code, int pulse_position[8], int i1, int i2, int i3)
Decode a 10-bit algebraic codebook index from a 10.2 kbit/s frame.
Definition: amrnbdec.c:435
static const uint8_t gray_decode[8]
3-bit Gray code to binary lookup table
Definition: amrnbdata.h:1438
static const float pred_fac[LP_FILTER_ORDER]
Prediction factor table for modes other than 12.2kbit/s.
Definition: amrnbdata.h:1420
float prev_sparse_fixed_gain
previous fixed gain; used by anti-sparseness processing to determine "onset"
Definition: amrnbdec.c:130
float postfilter_mem[10]
previous intermediate values in the formant filter
Definition: amrnbdec.c:134
common internal api header.
common internal and external API header
static void lsf2lsp_5(AMRContext *p)
Decode a set of 5 split-matrix quantized lsf indexes into 2 lsp vectors.
Definition: amrnbdec.c:298
static void lsf2lsp_for_mode12k2(AMRContext *p, double lsp[LP_FILTER_ORDER], const float lsf_no_r[LP_FILTER_ORDER], const int16_t *lsf_quantizer[5], const int quantizer_offset, const int sign, const int update)
Decode a set of 5 split-matrix quantized lsf indexes into an lsp vector.
Definition: amrnbdec.c:260
static const uint16_t gains_low[64][2]
gain table for 5.15 and 5.90 kbps modes
Definition: amrnbdata.h:1610
static void decode_gains(AMRContext *p, const AMRNBSubframe *amr_subframe, const enum Mode mode, const int subframe, float *fixed_gain_factor)
Decode pitch gain and fixed gain factor (part of section 6.1.3).
Definition: amrnbdec.c:630
void ff_set_min_dist_lsf(float *lsf, double min_spacing, int size)
Adjust the quantized LSFs so they are increasing and not too close.
Definition: lsp.c:51
void ff_acelp_filter_init(ACELPFContext *c)
Initialize ACELPFContext.
Definition: acelp_filters.c:148
const float ff_b60_sinc[61]
b60 hamming windowed sinc function coefficients
Definition: acelp_vectors.c:113
static const uint16_t gains_high[128][2]
gain table for 6.70, 7.40 and 10.2 kbps modes
Definition: amrnbdata.h:1578
uint8_t hang_count
the number of subframes since a hangover period started
Definition: amrnbdec.c:128
AMR narrowband data and definitions.
static const float energy_mean[8]
desired mean innovation energy, indexed by active mode
Definition: amrnbdata.h:1458
#define PITCH_LAG_MIN_MODE_12k2
Lower bound on decoded lag search in 12.2kbit/s mode.
Definition: amrnbdec.c:81
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
static const int8_t pulses[4]
Number of non-zero pulses in the MP-MLQ excitation.
Definition: g723_1_data.h:608
uint16_t pulses[10]
pulses: 10 for MODE_12k2, 7 for MODE_10k2, and index and sign for others
Definition: amrnbdata.h:62
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
struct AMRContext AMRContext
float excitation_buf[PITCH_DELAY_MAX+LP_FILTER_ORDER+1+AMR_SUBFRAME_SIZE]
current excitation and all necessary excitation history
Definition: amrnbdec.c:116
static enum Mode unpack_bitstream(AMRContext *p, const uint8_t *buf, int buf_size)
Unpack an RFC4867 speech frame into the AMR frame mode and parameters.
Definition: amrnbdec.c:207
#define AMR_TILT_GAMMA_T
Tilt factor = 1st reflection coefficient * gamma_t.
Definition: amrnbdec.c:96
float ff_amr_set_fixed_gain(float fixed_gain_factor, float fixed_mean_energy, float *prediction_error, float energy_mean, const float *pred_table)
Calculate fixed gain (part of section 6.1.3 of AMR spec)
Definition: acelp_pitch_delay.c:126
ACELPVContext acelpv_ctx
context for vector operations for ACELP-based codecs
Definition: amrnbdec.c:142
Generated on Wed Jun 18 2025 06:52:57 for FFmpeg by
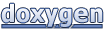