FFmpeg
|
acelp_pitch_delay.c
Go to the documentation of this file.
111 mr_energy += (((-6165LL * ff_log2(dsp->scalarproduct_int16(fc_v, fc_v, subframe_size, 0))) >> 3) & ~0x3ff);
void ff_decode_pitch_lag(int *lag_int, int *lag_frac, int pitch_index, const int prev_lag_int, const int subframe, int third_as_first, int resolution)
Decode the adaptive codebook index to the integer and fractional parts of the pitch lag for one subfr...
Definition: acelp_pitch_delay.c:147
static const uint16_t ma_prediction_coeff[4]
MA prediction coefficients (3.9.1 of G.729, near Equation 69)
Definition: g729data.h:343
int ff_exp2(uint16_t power)
fixed-point implementation of exp2(x) in [0; 1] domain.
Definition: celp_math.c:48
int ff_acelp_decode_6bit_to_2nd_delay6(int ac_index, int pitch_delay_min)
Decode pitch delay of the second subframe encoded by 6 bits with 1/6 precision.
Definition: acelp_pitch_delay.c:65
int ff_acelp_decode_4bit_to_2nd_delay3(int ac_index, int pitch_delay_min)
Decode pitch delay with 1/3 precision.
Definition: acelp_pitch_delay.c:39
int ff_acelp_decode_8bit_to_1st_delay3(int ac_index)
Decode pitch delay of the first subframe encoded by 8 bits with 1/3 resolution.
Definition: acelp_pitch_delay.c:31
int ff_acelp_decode_9bit_to_1st_delay6(int ac_index)
Decode pitch delay of the first subframe encoded by 9 bits with 1/6 precision.
Definition: acelp_pitch_delay.c:58
float avpriv_scalarproduct_float_c(const float *v1, const float *v2, int len)
Return the scalar product of two vectors.
Definition: float_dsp.c:107
int ff_acelp_decode_5_6_bit_to_2nd_delay3(int ac_index, int pitch_delay_min)
Decode pitch delay of the second subframe encoded by 5 or 6 bits with 1/3 precision.
Definition: acelp_pitch_delay.c:51
external API header
void ff_acelp_update_past_gain(int16_t *quant_energy, int gain_corr_factor, int log2_ma_pred_order, int erasure)
Update past quantized energies.
Definition: acelp_pitch_delay.c:72
int16_t ff_acelp_decode_gain_code(DSPContext *dsp, int gain_corr_factor, const int16_t *fc_v, int mr_energy, const int16_t *quant_energy, const int16_t *ma_prediction_coeff, int subframe_size, int ma_pred_order)
Decode the adaptive codebook gain and add correction (4.1.5 and 3.9.1 of G.729).
Definition: acelp_pitch_delay.c:93
1i.*Xphase exp()
The official guide to swscale for confused that consecutive non overlapping rectangles of slice_bottom special converter These generally are unscaled converters of common like for each output line the vertical scaler pulls lines from a ring buffer When the ring buffer does not contain the wanted then it is pulled from the input slice through the input converter and horizontal scaler The result is also stored in the ring buffer to serve future vertical scaler requests When no more output can be generated because lines from a future slice would be then all remaining lines in the current slice are horizontally scaled and put in the ring buffer[This is done for luma and chroma, each with possibly different numbers of lines per picture.] Input to YUV Converter When the input to the main path is not planar bits per component YUV or bit it is converted to planar bit YUV Two sets of converters exist for this the other leaves the full chroma resolution
Definition: swscale.txt:33
Replacements for frequently missing libm functions.
int32_t(* scalarproduct_int16)(const int16_t *v1, const int16_t *v2, int len)
Calculate scalar product of two vectors.
Definition: dsputil.h:274
common internal and external API header
static int bidir_sal(int value, int offset)
Shift value left or right depending on sign of offset parameter.
Definition: celp_math.h:71
static const float energy_mean[8]
desired mean innovation energy, indexed by active mode
Definition: amrnbdata.h:1458
float ff_amr_set_fixed_gain(float fixed_gain_factor, float fixed_mean_energy, float *prediction_error, float energy_mean, const float *pred_table)
Calculate fixed gain (part of section 6.1.3 of AMR spec)
Definition: acelp_pitch_delay.c:126
Generated on Fri May 30 2025 06:53:51 for FFmpeg by
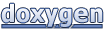