FFmpeg
|
swfenc.c
Go to the documentation of this file.
323 av_log(s, AV_LOG_ERROR, "swf does not support that sample rate, choose from (44100, 22050, 11025).\n");
Definition: swf.h:126
Definition: put_bits.h:41
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
unsigned int ff_codec_get_tag(const AVCodecTag *tags, enum AVCodecID id)
Definition: libavformat/utils.c:2604
#define AVFMT_TS_NONSTRICT
Format does not require strictly increasing timestamps, but they must still be monotonic.
Definition: avformat.h:363
int av_fifo_generic_write(AVFifoBuffer *f, void *src, int size, int(*func)(void *, void *, int))
Feed data from a user-supplied callback to an AVFifoBuffer.
Definition: libavutil/fifo.c:96
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
Definition: swf.h:60
Definition: libavcodec/avcodec.h:124
static void put_swf_rect(AVIOContext *pb, int xmin, int xmax, int ymin, int ymax)
Definition: swfenc.c:82
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
Definition: libavcodec/avcodec.h:110
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
static void put_swf_line_edge(PutBitContext *pb, int dx, int dy)
Definition: swfenc.c:109
static int swf_write_video(AVFormatContext *s, AVCodecContext *enc, const uint8_t *buf, int size)
Definition: swfenc.c:342
static uint8_t * put_bits_ptr(PutBitContext *s)
Return the pointer to the byte where the bitstream writer will put the next bit.
Definition: put_bits.h:199
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
int av_fifo_generic_read(AVFifoBuffer *f, void *dest, int buf_size, void(*func)(void *, void *, int))
Feed data from an AVFifoBuffer to a user-supplied callback.
Definition: libavutil/fifo.c:124
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
Definition: swf.h:83
int seekable
A combination of AVIO_SEEKABLE_ flags or 0 when the stream is not seekable.
Definition: avio.h:117
int void avio_flush(AVIOContext *s)
Force flushing of buffered data to the output s.
Definition: aviobuf.c:193
struct SWFContext SWFContext
static int swf_write_audio(AVFormatContext *s, AVCodecContext *enc, uint8_t *buf, int size)
Definition: swfenc.c:447
Definition: swf.h:45
static int swf_write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: swfenc.c:471
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
Definition: swf.h:43
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: swf.h:47
Definition: swf.h:89
Main libavformat public API header.
int av_fifo_size(AVFifoBuffer *f)
Return the amount of data in bytes in the AVFifoBuffer, that is the amount of data you can read from ...
Definition: libavutil/fifo.c:52
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
Definition: swf.h:66
Definition: avformat.h:377
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
Definition: swf.h:44
AVFifoBuffer * av_fifo_alloc(unsigned int size)
Initialize an AVFifoBuffer.
Definition: libavutil/fifo.c:25
Definition: swf.h:46
Definition: avutil.h:143
Definition: swf.h:76
Definition: libavcodec/avcodec.h:195
static void put_swf_matrix(AVIOContext *pb, int a, int b, int c, int d, int tx, int ty)
Definition: swfenc.c:138
Definition: swf.h:84
bitstream writer API
Generated on Fri Aug 1 2025 06:53:47 for FFmpeg by
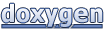