FFmpeg
|
rtjpeg.c
Go to the documentation of this file.
static int get_block(GetBitContext *gb, int16_t *block, const uint8_t *scan, const uint32_t *quant)
read one block from stream
Definition: rtjpeg.c:46
#define BLOCK(quant, dst, stride)
int ff_rtjpeg_decode_frame_yuv420(RTJpegContext *c, AVFrame *f, const uint8_t *buf, int buf_size)
decode one rtjpeg YUV420 frame
Definition: rtjpeg.c:106
void ff_rtjpeg_decode_init(RTJpegContext *c, DSPContext *dsp, int width, int height, const uint32_t *lquant, const uint32_t *cquant)
initialize an RTJpegContext, may be called multiple times
Definition: rtjpeg.c:156
bitstream reader API header.
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
FIXME Range Coding of cr are mx and my are Motion Vector top and top right vectors is used as motion vector prediction the used motion vector is the sum of the predictor and(mvx_diff, mvy_diff)*mv_scale Intra DC Predicton block[y][x] dc[1]
Definition: snow.txt:392
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Definition: rtjpeg.h:32
Definition: get_bits.h:54
common internal and external API header
Generated on Wed Jul 2 2025 06:53:37 for FFmpeg by
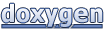