FFmpeg
|
dshow_pin.c
Go to the documentation of this file.
long WINAPI libAVPin_ReceiveConnection(libAVPin *this, IPin *pin, const AM_MEDIA_TYPE *type)
Definition: dshow_pin.c:40
Definition: start.py:1
long WINAPI libAVMemInputPin_GetAllocatorRequirements(libAVMemInputPin *this, ALLOCATOR_PROPERTIES *props)
Definition: dshow_pin.c:294
Definition: dshow_capture.h:63
Definition: basicDataTypeConversions.h:70
unsigned long WINAPI libAVPin_Release(libAVPin *)
long WINAPI libAVPin_QueryAccept(libAVPin *this, const AM_MEDIA_TYPE *type)
Definition: dshow_pin.c:146
long WINAPI libAVPin_QueryPinInfo(libAVPin *this, PIN_INFO *info)
Definition: dshow_pin.c:108
void libAVPin_Destroy(libAVPin *)
long WINAPI libAVPin_QueryId(libAVPin *this, wchar_t **id)
Definition: dshow_pin.c:134
long WINAPI libAVPin_Connect(libAVPin *, IPin *, const AM_MEDIA_TYPE *)
long WINAPI libAVMemInputPin_GetAllocator(libAVMemInputPin *this, IMemAllocator **alloc)
Definition: dshow_pin.c:281
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
Definition: dshow_capture.h:156
DECLARE_QUERYINTERFACE(libAVPin,{{&IID_IUnknown, 0},{&IID_IPin, 0},{&IID_IMemInputPin, imemoffset}})
Definition: dshow_pin.c:27
long WINAPI libAVMemInputPin_ReceiveCanBlock(libAVMemInputPin *this)
Definition: dshow_pin.c:349
long WINAPI libAVPin_EnumMediaTypes(libAVPin *this, IEnumMediaTypes **enumtypes)
Definition: dshow_pin.c:152
long ff_copy_dshow_media_type(AM_MEDIA_TYPE *dst, const AM_MEDIA_TYPE *src)
Definition: dshow_common.c:24
long WINAPI libAVPin_NewSegment(libAVPin *this, REFERENCE_TIME start, REFERENCE_TIME stop, double rate)
Definition: dshow_pin.c:196
unsigned long WINAPI libAVPin_AddRef(libAVPin *)
long WINAPI libAVPin_ConnectedTo(libAVPin *this, IPin **pin)
Definition: dshow_pin.c:82
long WINAPI libAVMemInputPin_QueryInterface(libAVMemInputPin *this, const GUID *riid, void **ppvObject)
Definition: dshow_pin.c:259
long WINAPI libAVPin_QueryDirection(libAVPin *this, PIN_DIRECTION *dir)
Definition: dshow_pin.c:125
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
unsigned long WINAPI libAVFilter_AddRef(libAVFilter *)
long WINAPI libAVMemInputPin_Receive(libAVMemInputPin *this, IMediaSample *sample)
Definition: dshow_pin.c:301
void libAVMemInputPin_Destroy(libAVMemInputPin *this)
Definition: dshow_pin.c:357
static int libAVPin_Setup(libAVPin *this, libAVFilter *filter)
Definition: dshow_pin.c:205
unsigned long WINAPI libAVMemInputPin_AddRef(libAVMemInputPin *this)
Definition: dshow_pin.c:267
Definition: dshow_capture.h:224
#define type
long WINAPI libAVPin_QueryInterface(libAVPin *, const GUID *, void **)
void(* callback)(void *priv_data, int index, uint8_t *buf, int buf_size, int64_t time)
Definition: dshow_capture.h:257
unsigned long WINAPI libAVMemInputPin_Release(libAVMemInputPin *this)
Definition: dshow_pin.c:274
long WINAPI libAVMemInputPin_NotifyAllocator(libAVMemInputPin *this, IMemAllocator *alloc, BOOL rdwr)
Definition: dshow_pin.c:287
long WINAPI libAVMemInputPin_ReceiveMultiple(libAVMemInputPin *this, IMediaSample **samples, long n, long *nproc)
Definition: dshow_pin.c:336
long WINAPI libAVPin_QueryInternalConnections(libAVPin *this, IPin **pin, unsigned long *npin)
Definition: dshow_pin.c:168
libAVEnumMediaTypes * libAVEnumMediaTypes_Create(const AM_MEDIA_TYPE *type)
Definition: dshow_capture.h:245
void ff_print_AM_MEDIA_TYPE(const AM_MEDIA_TYPE *type)
Definition: dshow_common.c:134
long WINAPI libAVPin_ConnectionMediaType(libAVPin *this, AM_MEDIA_TYPE *type)
Definition: dshow_pin.c:96
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
Generated on Fri May 30 2025 06:53:54 for FFmpeg by
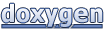