FFmpeg
|
dshow_capture.h
Go to the documentation of this file.
189 long WINAPI libAVMemInputPin_GetAllocatorRequirements(libAVMemInputPin *, ALLOCATOR_PROPERTIES *);
191 long WINAPI libAVMemInputPin_ReceiveMultiple (libAVMemInputPin *, IMediaSample **, long, long *);
234 long WINAPI libAVEnumMediaTypes_Next (libAVEnumMediaTypes *, unsigned long, AM_MEDIA_TYPE **, unsigned long *);
void libAVEnumPins_Destroy(libAVEnumPins *)
void libAVEnumMediaTypes_Destroy(libAVEnumMediaTypes *)
void libAVMemInputPin_Destroy(libAVMemInputPin *)
Definition: dshow_pin.c:357
long WINAPI libAVMemInputPin_GetAllocator(libAVMemInputPin *, IMemAllocator **)
Definition: dshow_pin.c:281
static void callback(void *priv_data, int index, uint8_t *buf, int buf_size, int64_t time)
Definition: dshow.c:200
libAVPin * libAVPin_Create(libAVFilter *filter)
void ff_print_VIDEO_STREAM_CONFIG_CAPS(const VIDEO_STREAM_CONFIG_CAPS *caps)
Definition: dshow_common.c:85
long WINAPI libAVMemInputPin_ReceiveMultiple(libAVMemInputPin *, IMediaSample **, long, long *)
Definition: dshow_pin.c:336
Definition: dshow_capture.h:63
Definition: basicDataTypeConversions.h:70
long WINAPI libAVMemInputPin_NotifyAllocator(libAVMemInputPin *, IMemAllocator *, BOOL)
Definition: dshow_pin.c:287
unsigned long WINAPI libAVPin_Release(libAVPin *)
long WINAPI libAVEnumMediaTypes_Skip(libAVEnumMediaTypes *, unsigned long)
Definition: dshow_enummediatypes.c:53
long WINAPI libAVFilter_EnumPins(libAVFilter *, IEnumPins **)
Definition: dshow_filter.c:96
long WINAPI libAVPin_ReceiveConnection(libAVPin *, IPin *, const AM_MEDIA_TYPE *)
Definition: dshow_pin.c:40
long WINAPI libAVMemInputPin_Receive(libAVMemInputPin *, IMediaSample *)
Definition: dshow_pin.c:301
unsigned long WINAPI libAVEnumMediaTypes_Release(libAVEnumMediaTypes *)
long WINAPI libAVPin_QueryPinInfo(libAVPin *, PIN_INFO *)
Definition: dshow_pin.c:108
long WINAPI libAVPin_NewSegment(libAVPin *, REFERENCE_TIME, REFERENCE_TIME, double)
Definition: dshow_pin.c:196
long WINAPI libAVFilter_SetSyncSource(libAVFilter *, IReferenceClock *)
Definition: dshow_filter.c:68
void libAVPin_Destroy(libAVPin *)
long WINAPI libAVEnumMediaTypes_Next(libAVEnumMediaTypes *, unsigned long, AM_MEDIA_TYPE **, unsigned long *)
long WINAPI libAVMemInputPin_ReceiveCanBlock(libAVMemInputPin *)
Definition: dshow_pin.c:349
long WINAPI libAVPin_EnumMediaTypes(libAVPin *, IEnumMediaTypes **)
Definition: dshow_pin.c:152
long WINAPI libAVFilter_QueryInterface(libAVFilter *, const GUID *, void **)
libAVFilter * libAVFilter_Create(void *, void *, enum dshowDeviceType)
Definition: dshow_capture.h:57
long WINAPI libAVPin_Connect(libAVPin *, IPin *, const AM_MEDIA_TYPE *)
long WINAPI libAVEnumMediaTypes_Clone(libAVEnumMediaTypes *, libAVEnumMediaTypes **)
Definition: dshow_enummediatypes.c:68
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
Definition: dshow_capture.h:156
long WINAPI libAVPin_QueryInternalConnections(libAVPin *, IPin **, unsigned long *)
Definition: dshow_pin.c:168
unsigned long WINAPI libAVEnumMediaTypes_AddRef(libAVEnumMediaTypes *)
Main libavdevice API header.
long ff_copy_dshow_media_type(AM_MEDIA_TYPE *dst, const AM_MEDIA_TYPE *src)
Definition: dshow_common.c:24
unsigned long WINAPI libAVEnumPins_AddRef(libAVEnumPins *)
long WINAPI libAVEnumPins_QueryInterface(libAVEnumPins *, const GUID *, void **)
long WINAPI libAVFilter_FindPin(libAVFilter *, const wchar_t *, IPin **)
Definition: dshow_filter.c:111
unsigned long WINAPI libAVPin_AddRef(libAVPin *)
long WINAPI libAVMemInputPin_GetAllocatorRequirements(libAVMemInputPin *, ALLOCATOR_PROPERTIES *)
Definition: dshow_pin.c:294
long WINAPI libAVEnumPins_Next(libAVEnumPins *, unsigned long, IPin **, unsigned long *)
void ff_print_AUDIO_STREAM_CONFIG_CAPS(const AUDIO_STREAM_CONFIG_CAPS *caps)
Definition: dshow_common.c:115
unsigned long WINAPI libAVMemInputPin_AddRef(libAVMemInputPin *)
Definition: dshow_pin.c:267
long WINAPI libAVFilter_GetSyncSource(libAVFilter *, IReferenceClock **)
Definition: dshow_filter.c:83
Definition: dshow_capture.h:64
long WINAPI libAVFilter_JoinFilterGraph(libAVFilter *, IFilterGraph *, const wchar_t *)
Definition: dshow_filter.c:142
long WINAPI libAVMemInputPin_QueryInterface(libAVMemInputPin *, const GUID *, void **)
Definition: dshow_pin.c:259
long WINAPI libAVFilter_QueryFilterInfo(libAVFilter *, FILTER_INFO *)
Definition: dshow_filter.c:129
unsigned long WINAPI libAVMemInputPin_Release(libAVMemInputPin *)
Definition: dshow_pin.c:274
unsigned long WINAPI libAVFilter_AddRef(libAVFilter *)
Definition: dshow_capture.h:224
#define type
libAVEnumPins * libAVEnumPins_Create(libAVPin *pin, libAVFilter *filter)
long WINAPI libAVPin_QueryAccept(libAVPin *, const AM_MEDIA_TYPE *)
Definition: dshow_pin.c:146
long WINAPI libAVPin_QueryInterface(libAVPin *, const GUID *, void **)
long WINAPI libAVPin_QueryDirection(libAVPin *, PIN_DIRECTION *)
Definition: dshow_pin.c:125
unsigned long WINAPI libAVFilter_Release(libAVFilter *)
long WINAPI libAVFilter_Run(libAVFilter *, REFERENCE_TIME)
Definition: dshow_filter.c:51
long WINAPI libAVEnumMediaTypes_Reset(libAVEnumMediaTypes *)
Definition: dshow_enummediatypes.c:61
long WINAPI libAVPin_ConnectionMediaType(libAVPin *, AM_MEDIA_TYPE *)
Definition: dshow_pin.c:96
long WINAPI libAVEnumPins_Skip(libAVEnumPins *, unsigned long)
Definition: dshow_enumpins.c:50
unsigned long WINAPI libAVEnumPins_Release(libAVEnumPins *)
Definition: dshow_capture.h:202
libAVEnumMediaTypes * libAVEnumMediaTypes_Create(const AM_MEDIA_TYPE *type)
Definition: dshow_capture.h:245
long WINAPI libAVFilter_GetState(libAVFilter *, DWORD, FILTER_STATE *)
Definition: dshow_filter.c:59
void ff_print_AM_MEDIA_TYPE(const AM_MEDIA_TYPE *type)
Definition: dshow_common.c:134
long WINAPI libAVFilter_GetClassID(libAVFilter *, CLSID *)
void libAVFilter_Destroy(libAVFilter *)
long WINAPI libAVFilter_QueryVendorInfo(libAVFilter *, wchar_t **)
Definition: dshow_filter.c:154
long WINAPI libAVEnumMediaTypes_QueryInterface(libAVEnumMediaTypes *, const GUID *, void **)
long WINAPI libAVEnumPins_Clone(libAVEnumPins *, libAVEnumPins **)
Definition: dshow_enumpins.c:65
Generated on Wed Apr 16 2025 06:53:13 for FFmpeg by
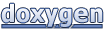