FFmpeg
|
tak_parser.c
Go to the documentation of this file.
int ff_tak_decode_frame_header(AVCodecContext *avctx, GetBitContext *gb, TAKStreamInfo *ti, int log_level_offset)
Validate and decode a frame header.
Definition: tak.c:143
Definition: tak_parser.c:30
Definition: parser.h:28
static int tak_parse(AVCodecParserContext *s, AVCodecContext *avctx, const uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size)
Definition: tak_parser.c:42
int ff_combine_frame(ParseContext *pc, int next, const uint8_t **buf, int *buf_size)
Combine the (truncated) bitstream to a complete frame.
Definition: parser.c:214
Definition: libavcodec/avcodec.h:3883
int overread
the number of bytes which where irreversibly read from the next frame
Definition: parser.h:35
Definition: libavcodec/avcodec.h:3747
Definition: tak.h:129
TAK (Tom's lossless Audio Kompressor) decoder/demuxer common functions.
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
struct TAKParseContext TAKParseContext
Definition: get_bits.h:54
Definition: libavcodec/avcodec.h:450
The official guide to swscale for confused that consecutive non overlapping rectangles of slice_bottom special converter These generally are unscaled converters of common like for each output line the vertical scaler pulls lines from a ring buffer When the ring buffer does not contain the wanted then it is pulled from the input slice through the input converter and horizontal scaler The result is also stored in the ring buffer to serve future vertical scaler requests When no more output can be generated because lines from a future slice would be needed
Definition: swscale.txt:33
Generated on Tue Jul 1 2025 06:52:31 for FFmpeg by
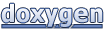