FFmpeg
|
tiffenc.c
Go to the documentation of this file.
337 av_fast_padded_mallocz(&s->strip_sizes, &s->strip_sizes_size, sizeof(s->strip_sizes[0]) * strips);
338 av_fast_padded_mallocz(&s->strip_offsets, &s->strip_offsets_size, sizeof(s->strip_offsets[0]) * strips);
509 {"dpi", "set the image resolution (in dpi)", OFFSET(dpi), AV_OPT_TYPE_INT, {.i64 = 72}, 1, 0x10000, AV_OPT_FLAG_VIDEO_PARAM|AV_OPT_FLAG_ENCODING_PARAM},
510 { "compression_algo", NULL, OFFSET(compr), AV_OPT_TYPE_INT, {.i64 = TIFF_PACKBITS}, TIFF_RAW, TIFF_DEFLATE, VE, "compression_algo" },
511 { "packbits", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = TIFF_PACKBITS}, 0, 0, VE, "compression_algo" },
Definition: tiff.h:62
const AVPixFmtDescriptor * av_pix_fmt_desc_get(enum AVPixelFormat pix_fmt)
Definition: pixdesc.c:1778
Definition: tiff.h:103
Definition: tiff.h:96
Definition: pixfmt.h:67
static int check_size(TiffEncoderContext *s, uint64_t need)
Check free space in buffer.
Definition: tiffenc.c:89
planar YUV 4:4:4, 24bpp, (1 Cr & Cb sample per 1x1 Y samples)
Definition: pixfmt.h:73
misc image utilities
Definition: tiff.h:60
Definition: opt.h:222
int av_get_bits_per_pixel(const AVPixFmtDescriptor *pixdesc)
Return the number of bits per pixel used by the pixel format described by pixdesc.
Definition: pixdesc.c:1731
TIFF tables.
int ff_lzw_encode(struct LZWEncodeState *s, const uint8_t *inbuf, int insize)
LZW main compress function.
Definition: lzwenc.c:226
Definition: lzw.h:39
void av_fast_padded_malloc(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_malloc but the buffer has additional FF_INPUT_BUFFER_PADDING_SIZE at the end w...
Definition: libavcodec/utils.c:101
static void add_entry(TiffEncoderContext *s, enum TiffTags tag, enum TiffTypes type, int count, const void *ptr_val)
Add entry to directory in tiff header.
Definition: tiffenc.c:128
struct TiffEncoderContext TiffEncoderContext
Definition: tiff.h:37
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Definition: tiff.h:51
Definition: opt.h:229
AVComponentDescriptor comp[4]
Parameters that describe how pixels are packed.
Definition: pixdesc.h:86
AVOptions.
packed RGB 16:16:16, 48bpp, 16R, 16G, 16B, the 2-byte value for each R/G/B component is stored as lit...
Definition: pixfmt.h:112
static void add_entry1(TiffEncoderContext *s, enum TiffTags tag, enum TiffTypes type, int val)
Definition: tiffenc.c:151
static const uint8_t type_sizes2[14]
sizes of various TIFF field types (string size = 1)
Definition: tiffenc.c:48
static void pack_yuv(TiffEncoderContext *s, uint8_t *dst, int lnum)
Definition: tiffenc.c:199
#define CODEC_FLAG_BITEXACT
Use only bitexact stuff (except (I)DCT).
Definition: libavcodec/avcodec.h:715
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
enum AVChromaLocation chroma_sample_location
This defines the location of chroma samples.
Definition: libavcodec/avcodec.h:1843
#define AV_OPT_FLAG_ENCODING_PARAM
a generic parameter which can be set by the user for muxing or encoding
Definition: opt.h:281
int ff_lzw_encode_flush(struct LZWEncodeState *s, void(*lzw_flush_put_bits)(struct PutBitContext *))
Definition: tiff.h:49
Definition: tiff.h:38
Definition: tiff.h:102
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: tiff.h:95
Definition: tiff.h:42
static const uint8_t type_sizes[14]
sizes of various TIFF field types (string size = 100)
Definition: tiff.h:171
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: tiff.h:74
Definition: tiff.h:72
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
planar YUV 4:2:2, 16bpp, (1 Cr & Cb sample per 2x1 Y samples)
Definition: pixfmt.h:72
#define FF_MIN_BUFFER_SIZE
minimum encoding buffer size Used to avoid some checks during header writing.
Definition: libavcodec/avcodec.h:568
void avcodec_get_chroma_sub_sample(enum AVPixelFormat pix_fmt, int *h_shift, int *v_shift)
Utility function to access log2_chroma_w log2_chroma_h from the pixel format AVPixFmtDescriptor.
Definition: imgconvert.c:65
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
Definition: tiff.h:105
Definition: tiff.h:97
static int encode_frame(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *pict, int *got_packet)
Definition: tiffenc.c:239
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
Descriptor that unambiguously describes how the bits of a pixel are stored in the up to 4 data planes...
Definition: pixdesc.h:55
Definition: tiff.h:76
planar YUV 4:1:0, 9bpp, (1 Cr & Cb sample per 4x4 Y samples)
Definition: pixfmt.h:74
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static void tnput(uint8_t **p, int n, const uint8_t *val, enum TiffTypes type, int flip)
Put n values to buffer.
Definition: tiffenc.c:108
must be printed separately If there s no standard function for printing the type you need
Definition: tablegen.txt:45
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
Definition: libavcodec/avcodec.h:199
LZW decoding routines.
Y , 1bpp, 0 is black, 1 is white, in each byte pixels are ordered from the msb to the lsb...
Definition: pixfmt.h:78
Definition: tiff.h:41
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
void ff_lzw_encode_init(struct LZWEncodeState *s, uint8_t *outbuf, int outsize, int maxbits, enum FF_LZW_MODES mode, void(*lzw_put_bits)(struct PutBitContext *, int, unsigned int))
common internal api header.
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
Y , 1bpp, 0 is white, 1 is black, in each byte pixels are ordered from the msb to the lsb...
Definition: pixfmt.h:77
Definition: tiff.h:75
void av_fast_padded_mallocz(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_padded_malloc except that buffer will always be 0-initialized after call...
Definition: libavcodec/utils.c:113
planar YUV 4:1:1, 12bpp, (1 Cr & Cb sample per 4x1 Y samples)
Definition: pixfmt.h:75
static av_always_inline void bytestream_put_buffer(uint8_t **b, const uint8_t *src, unsigned int size)
Definition: bytestream.h:337
Definition: tiffenc.c:52
Definition: tiff.h:48
Definition: tiff.h:39
Definition: tiff.h:50
Definition: avutil.h:143
int ff_rle_encode(uint8_t *outbuf, int out_size, const uint8_t *ptr, int bpp, int w, int add_rep, int xor_rep, int add_raw, int xor_raw)
RLE compress the row, with maximum size of out_size.
Definition: rle.c:58
Definition: tiff.h:104
static int encode_strip(TiffEncoderContext *s, const int8_t *src, uint8_t *dst, int n, int compr)
Encode one strip in tiff file.
Definition: tiffenc.c:168
packed RGBA 16:16:16:16, 64bpp, 16R, 16G, 16B, 16A, the 2-byte value for each R/G/B/A component is st...
Definition: pixfmt.h:210
bitstream writer API
Generated on Wed May 7 2025 06:52:47 for FFmpeg by
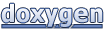