FFmpeg
|
indeo4.c
Go to the documentation of this file.
204 av_log(avctx, AV_LOG_ERROR, "Scalability: unsupported subdivision! Luma bands: %d, chroma bands: %d\n",
470 if (((tile->width + band->mb_size-1)/band->mb_size) * ((tile->height + band->mb_size-1)/band->mb_size) != tile->num_MBs) {
471 av_log(avctx, AV_LOG_ERROR, "num_MBs mismatch %d %d %d %d\n", tile->width, tile->height, band->mb_size, tile->num_MBs);
562 av_log(avctx, AV_LOG_ERROR, "motion vector %d %d outside reference\n", x*s + mb->mv_x, y*s + mb->mv_y);
static const uint16_t ivi4_quant_8x8_intra[9][64]
Indeo 4 dequant tables.
Definition: indeo4data.h:89
Definition: ivi_common.h:62
av_cold int ff_ivi_decode_close(AVCodecContext *avctx)
Close Indeo5 decoder and clean up its context.
Definition: ivi_common.c:931
This file contains data needed for the Indeo 4 decoder.
int(* is_nonnull_frame)(struct IVI45DecContext *ctx)
Definition: ivi_common.h:248
int ff_ivi_dec_huff_desc(GetBitContext *gb, int desc_coded, int which_tab, IVIHuffTab *huff_tab, AVCodecContext *avctx)
Decode a huffman codebook descriptor from the bitstream and select specified huffman table...
Definition: ivi_common.c:147
static int decode_pic_hdr(IVI45DecContext *ctx, AVCodecContext *avctx)
Decode Indeo 4 picture header.
Definition: indeo4.c:117
static int decode_plane_subdivision(GetBitContext *gb)
Decode subdivision of a plane.
Definition: indeo4.c:88
void( DCTransformPtr)(const int32_t *in, int16_t *out, uint32_t pitch, int blk_size)
Definition: ivi_common.h:79
void ff_ivi_dc_haar_2d(const int32_t *in, int16_t *out, uint32_t pitch, int blk_size)
DC-only two-dimensional inverse Haar transform for Indeo 4.
Definition: ivi_dsp.c:323
void ff_ivi_put_dc_pixel_8x8(const int32_t *in, int16_t *out, uint32_t pitch, int blk_size)
Copy the DC coefficient into the first pixel of the block and zero all others.
Definition: ivi_dsp.c:569
DSP functions (inverse transforms, motion compensations, wavelet recompostion) for Indeo Video Intera...
int is_halfpel
precision of the motion compensation: 0 - fullpel, 1 - halfpel
Definition: ivi_common.h:145
int(* decode_pic_hdr)(struct IVI45DecContext *ctx, AVCodecContext *avctx)
Definition: ivi_common.h:244
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
void(* switch_buffers)(struct IVI45DecContext *ctx)
Definition: ivi_common.h:247
Definition: af_biquads.c:76
av_cold int ff_ivi_init_planes(IVIPlaneDesc *planes, const IVIPicConfig *cfg)
Initialize planes (prepares descriptors, allocates buffers etc).
Definition: ivi_common.c:222
void ff_ivi_put_pixels_8x8(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
Copy the pixels into the frame buffer.
Definition: ivi_dsp.c:559
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
static const uint16_t ivi4_common_pic_sizes[14]
standard picture dimensions
Definition: indeo4data.h:37
int inherit_mv
tells if motion vector is inherited from reference macroblock
Definition: ivi_common.h:146
static int decode_band_hdr(IVI45DecContext *ctx, IVIBandDesc *band, AVCodecContext *avctx)
Decode Indeo 4 band header.
Definition: indeo4.c:281
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
bitstream reader API header.
void( InvTransformPtr)(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
Declare inverse transform function types.
Definition: ivi_common.h:78
int(* decode_band_hdr)(struct IVI45DecContext *ctx, IVIBandDesc *band, AVCodecContext *avctx)
Definition: ivi_common.h:245
void ff_ivi_row_slant8(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
inverse 1D row slant transform
Definition: ivi_dsp.c:480
void ff_ivi_dc_row_slant(const int32_t *in, int16_t *out, uint32_t pitch, int blk_size)
DC-only inverse row slant transform.
Definition: ivi_dsp.c:500
static const struct @57 transforms[18]
static int ivi_pic_config_cmp(IVIPicConfig *str1, IVIPicConfig *str2)
compare some properties of two pictures
Definition: ivi_common.h:255
void ff_ivi_inverse_haar_8x8(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
two-dimensional inverse Haar 8x8 transform for Indeo 4
Definition: ivi_dsp.c:268
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
This file contains structures and macros shared by both Indeo4 and Indeo5 decoders.
void ff_ivi_dc_col_slant(const int32_t *in, int16_t *out, uint32_t pitch, int blk_size)
DC-only inverse column slant transform.
Definition: ivi_dsp.c:545
struct IVI45DecContext IVI45DecContext
external API header
int inherit_qdelta
tells if quantiser delta is inherited from reference macroblock
Definition: ivi_common.h:147
int ff_ivi_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: ivi_common.c:844
Definition: indeo4.c:41
static void switch_buffers(IVI45DecContext *ctx)
Rearrange decoding and reference buffers.
Definition: indeo4.c:586
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
void ff_ivi_init_static_vlc(void)
Initialize static codes used for macroblock and block decoding.
Definition: ivi_common.c:103
static const uint8_t quant_index_to_tab[22]
Table for mapping quant matrix index from the bitstream into internal quant table number...
Definition: indeo4data.h:345
void ff_ivi_inverse_slant_8x8(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
two-dimensional inverse slant 8x8 transform
Definition: ivi_dsp.c:387
int is_2d_trans
1 indicates that the two-dimensional inverse transform is used
Definition: ivi_common.h:164
av_cold int ff_ivi_init_tiles(IVIPlaneDesc *planes, int tile_width, int tile_height)
Initialize tile and macroblock descriptors.
Definition: ivi_common.c:286
planar YUV 4:1:0, 9bpp, (1 Cr & Cb sample per 4x4 Y samples)
Definition: pixfmt.h:74
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
IVIMbInfo * ref_mbs
ptr to the macroblock descriptors of the reference tile
Definition: ivi_common.h:123
void ff_ivi_inverse_slant_4x4(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
two-dimensional inverse slant 4x4 transform
Definition: ivi_dsp.c:427
Definition: ivi_common.h:187
Definition: get_bits.h:54
static int decode_mb_info(IVI45DecContext *ctx, IVIBandDesc *band, IVITile *tile, AVCodecContext *avctx)
Decode information (block type, cbp, quant delta, motion vector) for all macroblocks in the current t...
Definition: indeo4.c:451
Definition: libavcodec/avcodec.h:214
void ff_ivi_dc_slant_2d(const int32_t *in, int16_t *out, uint32_t pitch, int blk_size)
DC-only two-dimensional inverse slant transform.
Definition: ivi_dsp.c:467
int(* decode_mb_info)(struct IVI45DecContext *ctx, IVIBandDesc *band, IVITile *tile, AVCodecContext *avctx)
Definition: ivi_common.h:246
Definition: ivi_common.h:198
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
#define IVI_TOSIGNED(val)
convert unsigned values into signed ones (the sign is in the LSB)
Definition: ivi_common.h:271
void ff_ivi_col_slant8(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
inverse 1D column slant transform
Definition: ivi_dsp.c:518
Generated on Wed May 7 2025 06:52:42 for FFmpeg by
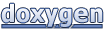