FFmpeg
|
ivi_common.h
Go to the documentation of this file.
37 #define IVI4_STREAM_ANALYSER 0
78 typedef void (InvTransformPtr)(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags);
89 int8_t valtab[256];
246 int (*decode_mb_info) (struct IVI45DecContext *ctx, IVIBandDesc *band, IVITile *tile, AVCodecContext *avctx);
Definition: ivi_common.h:62
static int decode_pic_hdr(IVI45DecContext *ctx, AVCodecContext *avctx)
Decode Indeo 4 picture header.
Definition: indeo4.c:117
void( DCTransformPtr)(const int32_t *in, int16_t *out, uint32_t pitch, int blk_size)
Definition: ivi_common.h:79
int16_t * ref_buf
pointer to the reference frame buffer (for motion compensation)
Definition: ivi_common.h:139
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
int is_halfpel
precision of the motion compensation: 0 - fullpel, 1 - halfpel
Definition: ivi_common.h:145
void ff_ivi_init_static_vlc(void)
Initialize static codes used for macroblock and block decoding.
Definition: ivi_common.c:103
Definition: af_biquads.c:76
const uint8_t ff_ivi_vertical_scan_8x8[64]
Common scan patterns (defined in ivi_common.c)
Definition: ivi_common.c:993
int ff_ivi_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: ivi_common.c:844
int inherit_mv
tells if motion vector is inherited from reference macroblock
Definition: ivi_common.h:146
static int decode_band_hdr(IVI45DecContext *ctx, IVIBandDesc *band, AVCodecContext *avctx)
Decode Indeo 4 band header.
Definition: indeo4.c:281
struct IVIPicConfig IVIPicConfig
int qdelta_present
tells if Qdelta signal is present in the bitstream (Indeo5 only)
Definition: ivi_common.h:148
bitstream reader API header.
void( InvTransformPtr)(const int32_t *in, int16_t *out, uint32_t pitch, const uint8_t *flags)
Declare inverse transform function types.
Definition: ivi_common.h:78
int ff_ivi_init_planes(IVIPlaneDesc *planes, const IVIPicConfig *cfg)
Initialize planes (prepares descriptors, allocates buffers etc).
Definition: ivi_common.c:222
static int ivi_pic_config_cmp(IVIPicConfig *str1, IVIPicConfig *str2)
compare some properties of two pictures
Definition: ivi_common.h:255
int ff_ivi_decode_close(AVCodecContext *avctx)
Close Indeo5 decoder and clean up its context.
Definition: ivi_common.c:931
int ff_ivi_init_tiles(IVIPlaneDesc *planes, int tile_width, int tile_height)
Initialize tile and macroblock descriptors.
Definition: ivi_common.c:286
struct IVI45DecContext IVI45DecContext
external API header
Definition: get_bits.h:63
int inherit_qdelta
tells if quantiser delta is inherited from reference macroblock
Definition: ivi_common.h:147
static void switch_buffers(IVI45DecContext *ctx)
Rearrange decoding and reference buffers.
Definition: indeo4.c:586
int is_2d_trans
1 indicates that the two-dimensional inverse transform is used
Definition: ivi_common.h:164
IVIMbInfo * ref_mbs
ptr to the macroblock descriptors of the reference tile
Definition: ivi_common.h:123
Definition: ivi_common.h:187
Definition: get_bits.h:54
static int decode_mb_info(IVI45DecContext *ctx, IVIBandDesc *band, IVITile *tile, AVCodecContext *avctx)
Decode information (block type, cbp, quant delta, motion vector) for all macroblocks in the current t...
Definition: indeo4.c:451
Definition: ivi_common.h:198
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
int ff_ivi_dec_huff_desc(GetBitContext *gb, int desc_coded, int which_tab, IVIHuffTab *huff_tab, AVCodecContext *avctx)
Decode a huffman codebook descriptor from the bitstream and select specified huffman table...
Definition: ivi_common.c:147
Generated on Thu Jun 19 2025 06:53:09 for FFmpeg by
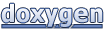