FFmpeg
|
mpegvideo_bfin.c
Go to the documentation of this file.
if max(w)>1 w=0.9 *w/max(w)
uint16_t(* q_chroma_intra_matrix16)[2][64]
Definition: mpegvideo.h:497
void ff_block_permute(int16_t *block, uint8_t *permutation, const uint8_t *scantable, int last)
Permute an 8x8 block.
Definition: mpegvideo.c:3045
mpegvideo header.
Macro definitions for various function/variable attributes.
external API header
uint16_t(* q_intra_matrix16)[2][64]
identical to the above but for MMX & these are not permutated, second 64 entries are bias ...
Definition: mpegvideo.h:496
FIXME Range Coding of cr are mx and my are Motion Vector top and top right vectors is used as motion vector prediction the used motion vector is the sum of the predictor and(mvx_diff, mvy_diff)*mv_scale Intra DC Predicton block[y][x] dc[1]
Definition: snow.txt:392
static int dct_quantize_bfin(MpegEncContext *s, int16_t *block, int n, int qscale, int *overflow)
Definition: mpegvideo_bfin.c:28
void(* denoise_dct)(struct MpegEncContext *s, int16_t *block)
Definition: mpegvideo.h:730
av_cold void ff_MPV_common_init_bfin(MpegEncContext *s)
Definition: mpegvideo_bfin.c:149
Generated on Wed May 8 2024 06:52:09 for FFmpeg by
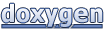