FFmpeg
|
mss12.c
Go to the documentation of this file.
int coded_width
Bitstream width / height, may be different from width/height e.g.
Definition: libavcodec/avcodec.h:1312
av_cold int ff_mss12_decode_init(MSS12Context *c, int version, SliceContext *sc1, SliceContext *sc2)
Definition: mss12.c:562
static av_always_inline float av_int2float(uint32_t i)
Reinterpret a 32-bit integer as a float.
Definition: intfloat.h:40
Definition: mss12.h:40
static int decode_region_inter(SliceContext *sc, ArithCoder *acoder, int x, int y, int width, int height)
Definition: mss12.c:489
int ff_mss12_decode_rect(SliceContext *sc, ArithCoder *acoder, int x, int y, int width, int height)
Definition: mss12.c:526
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: mss12.h:58
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
static av_cold void slicecontext_init(SliceContext *sc, int version, int full_model_syms)
Definition: mss12.c:411
static int decode_region_intra(SliceContext *sc, ArithCoder *acoder, int x, int y, int width, int height)
Definition: mss12.c:457
Definition: mss12.h:75
Definition: mss12.h:68
external API header
static int decode_pivot(SliceContext *sc, ArithCoder *acoder, int base)
Definition: mss12.c:437
static av_cold void pixctx_init(PixContext *ctx, int cache_size, int full_model_syms, int special_initial_cache)
Definition: mss12.c:138
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
Definition: mss12.c:43
static int motion_compensation(MSS12Context const *c, int x, int y, int width, int height)
Definition: mss12.c:334
Definition: mss12.c:41
Definition: mss12.h:48
static int decode_pixel_in_context(ArithCoder *acoder, PixContext *pctx, uint8_t *src, int stride, int x, int y, int has_right)
Definition: mss12.c:197
Definition: mss12.c:32
static int decode_region(ArithCoder *acoder, uint8_t *dst, uint8_t *rgb_pic, int x, int y, int width, int height, int stride, int rgb_stride, PixContext *pctx, const uint32_t *pal)
Definition: mss12.c:290
static int decode_region_masked(MSS12Context const *c, ArithCoder *acoder, uint8_t *dst, int stride, uint8_t *mask, int mask_stride, int x, int y, int width, int height, PixContext *pctx)
Definition: mss12.c:368
Definition: mss12.c:33
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
Definition: mss12.c:40
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static av_cold void model_init(Model *m, int num_syms, int thr_weight)
Definition: mss12.c:69
void ff_mss12_slicecontext_reset(SliceContext *sc)
Definition: mss12.c:426
Definition: mss12.c:34
static av_always_inline int decode_pixel(ArithCoder *acoder, PixContext *pctx, uint8_t *ngb, int num_ngb, int any_ngb)
Definition: mss12.c:157
Definition: mss12.c:42
static void copy_rectangles(MSS12Context const *c, int x, int y, int width, int height)
Definition: mss12.c:318
Common header for Microsoft Screen 1 and 2.
Generated on Tue Aug 5 2025 06:52:55 for FFmpeg by
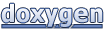