FFmpeg
|
mxpegdec.c
Go to the documentation of this file.
Definition: mjpeg.h:115
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: mjpeg.h:74
static int mxpeg_check_dimensions(MXpegDecodeContext *s, MJpegDecodeContext *jpg, AVFrame *reference_ptr)
Definition: mxpegdec.c:133
MJPEG encoder and decoder.
static int mxpeg_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: mxpegdec.c:158
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
Definition: mxpegdec.c:32
Definition: mjpeg.h:77
Definition: mjpeg.h:83
static int mxpeg_decode_mxm(MXpegDecodeContext *s, const uint8_t *buf_ptr, int buf_size)
Definition: mxpegdec.c:65
Definition: mjpeg.h:43
av_cold int ff_mjpeg_decode_end(AVCodecContext *avctx)
Definition: mjpegdec.c:1867
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: mjpeg.h:60
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
Definition: mjpeg.h:76
Definition: libavcodec/avcodec.h:249
Definition: mjpegdec.h:41
Definition: mjpeg.h:75
static av_cold int mxpeg_decode_init(AVCodecContext *avctx)
Definition: mxpegdec.c:45
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
static int mxpeg_decode_com(MXpegDecodeContext *s, const uint8_t *buf_ptr, int buf_size)
Definition: mxpegdec.c:118
av_cold int ff_mjpeg_decode_init(AVCodecContext *avctx)
Definition: mjpegdec.c:83
int ff_mjpeg_decode_sos(MJpegDecodeContext *s, const uint8_t *mb_bitmask, const AVFrame *reference)
Definition: mjpegdec.c:1177
common internal api header.
static av_cold int mxpeg_decode_end(AVCodecContext *avctx)
Definition: mxpegdec.c:311
static int mxpeg_decode_app(MXpegDecodeContext *s, const uint8_t *buf_ptr, int buf_size)
Definition: mxpegdec.c:53
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
int ff_mjpeg_find_marker(MJpegDecodeContext *s, const uint8_t **buf_ptr, const uint8_t *buf_end, const uint8_t **unescaped_buf_ptr, int *unescaped_buf_size)
Definition: mjpegdec.c:1541
struct MXpegDecodeContext MXpegDecodeContext
MJPEG decoder.
#define AV_GET_BUFFER_FLAG_REF
The decoder will keep a reference to the frame and may reuse it later.
Definition: libavcodec/avcodec.h:909
Definition: mjpeg.h:98
Generated on Thu Jun 19 2025 06:53:11 for FFmpeg by
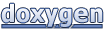