FFmpeg
|
MJPEG decoder. More...
#include "libavutil/imgutils.h"
#include "libavutil/avassert.h"
#include "libavutil/opt.h"
#include "avcodec.h"
#include "copy_block.h"
#include "internal.h"
#include "mjpeg.h"
#include "mjpegdec.h"
#include "jpeglsdec.h"
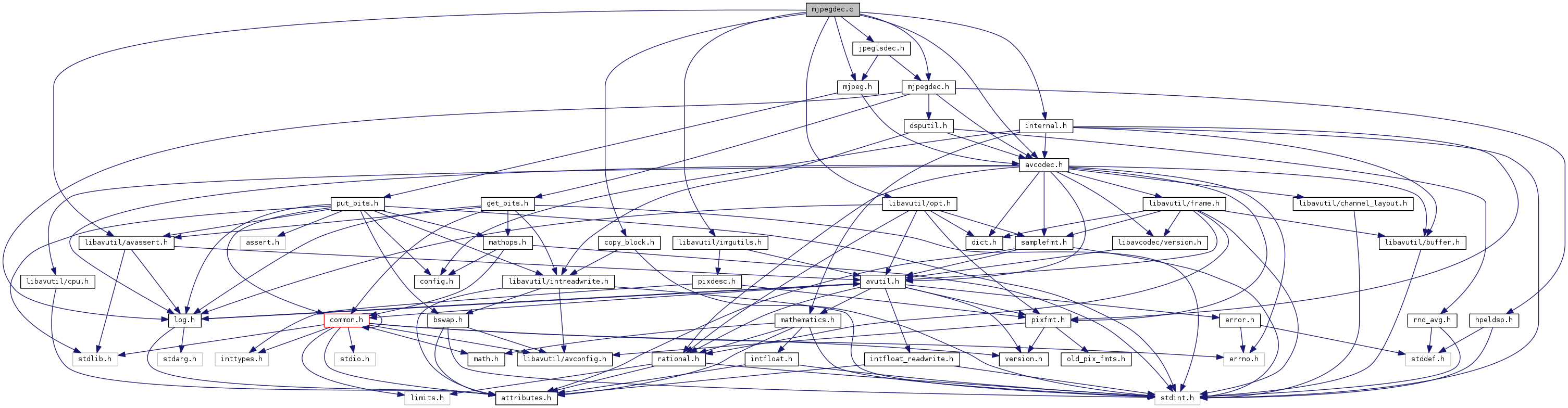
Go to the source code of this file.
Macros | |
#define | REFINE_BIT(j) |
#define | ZERO_RUN |
Functions | |
static int | build_vlc (VLC *vlc, const uint8_t *bits_table, const uint8_t *val_table, int nb_codes, int use_static, int is_ac) |
static void | build_basic_mjpeg_vlc (MJpegDecodeContext *s) |
av_cold int | ff_mjpeg_decode_init (AVCodecContext *avctx) |
int | ff_mjpeg_decode_dqt (MJpegDecodeContext *s) |
int | ff_mjpeg_decode_dht (MJpegDecodeContext *s) |
int | ff_mjpeg_decode_sof (MJpegDecodeContext *s) |
static int | mjpeg_decode_dc (MJpegDecodeContext *s, int dc_index) |
static int | decode_block (MJpegDecodeContext *s, int16_t *block, int component, int dc_index, int ac_index, int16_t *quant_matrix) |
static int | decode_dc_progressive (MJpegDecodeContext *s, int16_t *block, int component, int dc_index, int16_t *quant_matrix, int Al) |
static int | decode_block_progressive (MJpegDecodeContext *s, int16_t *block, uint8_t *last_nnz, int ac_index, int16_t *quant_matrix, int ss, int se, int Al, int *EOBRUN) |
static int | decode_block_refinement (MJpegDecodeContext *s, int16_t *block, uint8_t *last_nnz, int ac_index, int16_t *quant_matrix, int ss, int se, int Al, int *EOBRUN) |
static void | handle_rstn (MJpegDecodeContext *s, int nb_components) |
static int | ljpeg_decode_rgb_scan (MJpegDecodeContext *s, int nb_components, int predictor, int point_transform) |
static int | ljpeg_decode_yuv_scan (MJpegDecodeContext *s, int nb_components, int predictor, int point_transform) |
static av_always_inline void | mjpeg_copy_block (MJpegDecodeContext *s, uint8_t *dst, const uint8_t *src, int linesize, int lowres) |
static int | mjpeg_decode_scan (MJpegDecodeContext *s, int nb_components, int Ah, int Al, const uint8_t *mb_bitmask, const AVFrame *reference) |
static int | mjpeg_decode_scan_progressive_ac (MJpegDecodeContext *s, int ss, int se, int Ah, int Al) |
int | ff_mjpeg_decode_sos (MJpegDecodeContext *s, const uint8_t *mb_bitmask, const AVFrame *reference) |
static int | mjpeg_decode_dri (MJpegDecodeContext *s) |
static int | mjpeg_decode_app (MJpegDecodeContext *s) |
static int | mjpeg_decode_com (MJpegDecodeContext *s) |
static int | find_marker (const uint8_t **pbuf_ptr, const uint8_t *buf_end) |
int | ff_mjpeg_find_marker (MJpegDecodeContext *s, const uint8_t **buf_ptr, const uint8_t *buf_end, const uint8_t **unescaped_buf_ptr, int *unescaped_buf_size) |
int | ff_mjpeg_decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
av_cold int | ff_mjpeg_decode_end (AVCodecContext *avctx) |
static void | decode_flush (AVCodecContext *avctx) |
Detailed Description
MJPEG decoder.
Definition in file mjpegdec.c.
Macro Definition Documentation
#define REFINE_BIT | ( | j | ) |
Definition at line 641 of file mjpegdec.c.
Referenced by decode_block_refinement().
#define ZERO_RUN |
Definition at line 649 of file mjpegdec.c.
Referenced by decode_block_refinement().
Function Documentation
|
static |
Definition at line 67 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_init().
|
static |
Definition at line 44 of file mjpegdec.c.
Referenced by build_basic_mjpeg_vlc(), and ff_mjpeg_decode_dht().
|
static |
Definition at line 503 of file mjpegdec.c.
Referenced by mjpeg_decode_scan().
|
static |
Definition at line 569 of file mjpegdec.c.
Referenced by mjpeg_decode_scan_progressive_ac().
|
static |
Definition at line 667 of file mjpegdec.c.
Referenced by mjpeg_decode_scan_progressive_ac().
|
static |
Definition at line 551 of file mjpegdec.c.
Referenced by mjpeg_decode_scan().
|
static |
Definition at line 1890 of file mjpegdec.c.
int ff_mjpeg_decode_dht | ( | MJpegDecodeContext * | s | ) |
Definition at line 159 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame(), ff_mjpeg_decode_init(), mjpegb_decode_frame(), and mxpeg_decode_frame().
int ff_mjpeg_decode_dqt | ( | MJpegDecodeContext * | s | ) |
Definition at line 126 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame(), mjpegb_decode_frame(), and mxpeg_decode_frame().
av_cold int ff_mjpeg_decode_end | ( | AVCodecContext * | avctx | ) |
Definition at line 1867 of file mjpegdec.c.
Referenced by decode_flush(), end(), mxpeg_decode_end(), and sp5x_decode_frame().
int ff_mjpeg_decode_frame | ( | AVCodecContext * | avctx, |
void * | data, | ||
int * | got_frame, | ||
AVPacket * | avpkt | ||
) |
Definition at line 1629 of file mjpegdec.c.
Referenced by decode_flush(), decode_frame(), and sp5x_decode_frame().
av_cold int ff_mjpeg_decode_init | ( | AVCodecContext * | avctx | ) |
Definition at line 83 of file mjpegdec.c.
Referenced by decode_flush(), init(), mxpeg_decode_init(), and sp5x_decode_frame().
int ff_mjpeg_decode_sof | ( | MJpegDecodeContext * | s | ) |
Definition at line 213 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame(), mjpegb_decode_frame(), and mxpeg_decode_frame().
int ff_mjpeg_decode_sos | ( | MJpegDecodeContext * | s, |
const uint8_t * | mb_bitmask, | ||
const AVFrame * | reference | ||
) |
Definition at line 1177 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame(), mjpegb_decode_frame(), and mxpeg_decode_frame().
int ff_mjpeg_find_marker | ( | MJpegDecodeContext * | s, |
const uint8_t ** | buf_ptr, | ||
const uint8_t * | buf_end, | ||
const uint8_t ** | unescaped_buf_ptr, | ||
int * | unescaped_buf_size | ||
) |
Definition at line 1541 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame(), and mxpeg_decode_frame().
Definition at line 1517 of file mjpegdec.c.
Referenced by ff_mjpeg_find_marker().
|
static |
Definition at line 732 of file mjpegdec.c.
Referenced by mjpeg_decode_scan(), and mjpeg_decode_scan_progressive_ac().
|
static |
Definition at line 762 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_sos().
|
static |
Definition at line 849 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_sos().
|
static |
Definition at line 992 of file mjpegdec.c.
Referenced by mjpeg_decode_scan().
|
static |
Definition at line 1346 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame().
|
static |
Definition at line 1482 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame().
|
inlinestatic |
Definition at line 485 of file mjpegdec.c.
Referenced by decode_block(), decode_dc_progressive(), ljpeg_decode_rgb_scan(), and ljpeg_decode_yuv_scan().
|
static |
Definition at line 1334 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_frame().
|
static |
Definition at line 1008 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_sos().
|
static |
Definition at line 1119 of file mjpegdec.c.
Referenced by ff_mjpeg_decode_sos().
Generated on Wed May 8 2024 06:52:19 for FFmpeg by
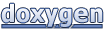