FFmpeg
|
libschroedingerdec.c
Go to the documentation of this file.
Definition: pixfmt.h:67
misc image utilities
memory handling functions
SchroFrame * ff_create_schro_frame(AVCodecContext *avctx, SchroFrameFormat schro_frame_fmt)
Create a Schro frame based on the dimensions and frame format passed.
Definition: libschroedinger.c:178
static av_cold int libschroedinger_decode_init(AVCodecContext *avctx)
Definition: libschroedingerdec.c:147
static SchroBuffer * find_next_parse_unit(SchroParseUnitContext *parse_ctx)
Definition: libschroedingerdec.c:90
static enum AVPixelFormat get_chroma_format(SchroChromaFormat schro_pix_fmt)
Returns FFmpeg chroma format.
Definition: libschroedingerdec.c:135
data structures common to libschroedinger decoder and encoder
static void libschroedinger_decode_buffer_free(SchroBuffer *schro_buf, void *priv)
Definition: libschroedingerdec.c:77
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
Definition: libavcodec/avcodec.h:219
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
void * ff_schro_queue_pop(FFSchroQueue *queue)
Return the first element in the queue.
Definition: libschroedinger.c:100
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
external API header
static av_cold int libschroedinger_decode_close(AVCodecContext *avctx)
Definition: libschroedingerdec.c:345
static void libschroedinger_flush(AVCodecContext *avctx)
Definition: libschroedingerdec.c:359
int ff_get_schro_frame_format(SchroChromaFormat schro_pix_fmt, SchroFrameFormat *schro_frame_fmt)
Sets the Schroedinger frame format corresponding to the Schro chroma format passed.
Definition: libschroedinger.c:150
common internal API header
int av_image_check_size(unsigned int w, unsigned int h, int log_offset, void *log_ctx)
Check if the given dimension of an image is valid, meaning that all bytes of the image can be address...
Definition: imgutils.c:231
int ff_schro_queue_push_back(FFSchroQueue *queue, void *p_data)
Add an element to the end of the queue.
Definition: libschroedinger.c:81
void ff_schro_queue_free(FFSchroQueue *queue, void(*free_func)(void *))
Free the queue resources.
Definition: libschroedinger.c:75
Definition: libschroedingerdec.c:71
static const struct @67 schro_pixel_format_map[]
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
static int libschroedinger_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: libschroedingerdec.c:202
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int64_t pkt_pts
PTS copied from the AVPacket that was decoded to produce this frame.
Definition: frame.h:164
static void parse_context_init(SchroParseUnitContext *parse_ctx, const uint8_t *buf, int buf_size)
Definition: libschroedingerdec.c:83
common internal api header.
struct SchroParseUnitContext SchroParseUnitContext
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
static void libschroedinger_decode_frame_free(void *frame)
Definition: libschroedingerdec.c:166
static void libschroedinger_handle_first_access_unit(AVCodecContext *avctx)
Definition: libschroedingerdec.c:171
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
Generated on Wed May 8 2024 06:52:07 for FFmpeg by
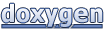