FFmpeg
|
libopusdec.c
Go to the documentation of this file.
static int libopus_decode(AVCodecContext *avc, void *data, int *got_frame_ptr, AVPacket *pkt)
Definition: libopusdec.c:127
Definition: samplefmt.h:50
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
external API header
Definition: libopusdec.c:33
static av_cold int libopus_decode_init(AVCodecContext *avc)
Definition: libopusdec.c:43
Harmonics mapping(fx) Xmag(ploc)
Definition: libavcodec/avcodec.h:449
union libopus_context::@66 gain
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
int skip_samples
Number of audio samples to skip at the start of the next decoded frame.
Definition: libavcodec/internal.h:109
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
common internal api header.
struct AVCodecInternal * internal
Private context used for internal data.
Definition: libavcodec/avcodec.h:1178
static av_cold int libopus_decode_close(AVCodecContext *avc)
Definition: libopusdec.c:117
const uint8_t ff_vorbis_channel_layout_offsets[8][8]
Definition: vorbis_data.c:25
Generated on Wed May 7 2025 06:52:42 for FFmpeg by
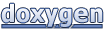