FFmpeg
|
amrwbdec.c
Go to the documentation of this file.
62 float excitation_buf[AMRWB_P_DELAY_MAX + LP_ORDER + 2 + AMRWB_SFR_SIZE]; ///< current excitation and all necessary excitation history
68 float prediction_error[4]; ///< quantified prediction errors {20log10(^gamma_gc)} for previous four subframes
74 float prev_sparse_fixed_gain; ///< previous fixed gain; used by anti-sparseness to determine "onset"
75 uint8_t prev_ir_filter_nr; ///< previous impulse response filter "impNr": 0 - strong, 1 - medium, 2 - none
78 float samples_az[LP_ORDER + AMRWB_SFR_SIZE]; ///< low-band samples and memory from synthesis at 12.8kHz
79 float samples_up[UPS_MEM_SIZE + AMRWB_SFR_SIZE]; ///< low-band samples and memory processed for upsampling
80 float samples_hb[LP_ORDER_16k + AMRWB_SFR_SIZE_16k]; ///< high-band samples and memory from synthesis at 16kHz
1102 float spare_vector[AMRWB_SFR_SIZE]; // extra stack space to hold result from anti-sparseness processing
Definition: amrwbdata.h:69
float samples_up[UPS_MEM_SIZE+AMRWB_SFR_SIZE]
low-band samples and memory processed for upsampling
Definition: amrwbdec.c:79
Definition: libavcodec/avcodec.h:368
static const uint8_t pulses_nb_per_mode_tr[][4]
[i][j] is the number of pulses present in track j at mode i
Definition: amrwbdata.h:1656
static const int16_t qua_gain_6b[64][2]
Tables for decoding quantized gains { pitch (Q14), fixed factor (Q11) }.
Definition: amrwbdata.h:1663
Definition: amrwbdata.h:78
static void hb_fir_filter(float *out, const float fir_coef[HB_FIR_SIZE+1], float mem[HB_FIR_SIZE], const float *in)
Apply a 15th order filter to high-band samples.
Definition: amrwbdec.c:1054
float fixed_gain[2]
quantified fixed gains for the current and previous subframes
Definition: amrwbdec.c:70
static void decode_pitch_lag_low(int *lag_int, int *lag_frac, int pitch_index, uint8_t *base_lag_int, int subframe, enum Mode mode)
Decode an adaptive codebook index into pitch lag for 8k85 and 6k60 modes.
Definition: amrwbdec.c:292
float pitch_vector[AMRWB_SFR_SIZE]
adaptive codebook (pitch) vector for current subframe
Definition: amrwbdec.c:65
float prev_tr_gain
previous initial gain used by noise enhancer for threshold
Definition: amrwbdec.c:76
void(* acelp_interpolatef)(float *out, const float *in, const float *filter_coeffs, int precision, int frac_pos, int filter_length, int length)
Floating point version of ff_acelp_interpolate()
Definition: acelp_filters.h:32
float(* dot_productf)(const float *a, const float *b, int length)
Return the dot product.
Definition: celp_math.h:37
static void decode_5p_track(int *out, int code, int m, int off)
code: 5m bits
Definition: amrwbdec.c:441
static void extrapolate_isf(float isf[LP_ORDER_16k])
Extrapolate a ISF vector to the 16kHz range (20th order LP) used at mode 6k60 LP filter for the high ...
Definition: amrwbdec.c:926
static void decode_6p_track(int *out, int code, int m, int off)
code: 6m-2 bits
Definition: amrwbdec.c:451
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
static float stability_factor(const float *isf, const float *isf_past)
Calculate a stability factor {teta} based on distance between current and past isf.
Definition: amrwbdec.c:694
static void isf_add_mean_and_past(float *isf_q, float *isf_past)
Apply mean and past ISF values using the prediction factor.
Definition: amrwbdec.c:217
float isf_past_final[LP_ORDER]
final processed ISF vector of the previous frame
Definition: amrwbdec.c:53
static void lpc_weighting(float *out, const float *lpc, float gamma, int size)
Spectral expand the LP coefficients using the equation: y[i] = x[i] * (gamma ** i) ...
Definition: amrwbdec.c:991
static void pitch_enhancer(float *fixed_vector, float voice_fac)
Filter the fixed_vector to emphasize the higher frequencies.
Definition: amrwbdec.c:745
Reference: libavcodec/amrwbdec.c.
static void scaled_hb_excitation(AMRWBContext *ctx, float *hb_exc, const float *synth_exc, float hb_gain)
Generate the high-band excitation with the same energy from the lower one and scaled by the given gai...
Definition: amrwbdec.c:888
struct AMRWBContext AMRWBContext
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
float lpf_7_mem[HB_FIR_SIZE]
previous values in the high-band low pass filter
Definition: amrwbdec.c:85
static int amrwb_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: amrwbdec.c:1092
Definition: samplefmt.h:50
void ff_amrwb_lsp2lpc(const double *lsp, float *lp, int lp_order)
LSP to LP conversion (5.2.4 of AMR-WB)
Definition: lsp.c:145
Definition: acelp_vectors.h:28
Definition: acelp_filters.h:28
static const float energy_pred_fac[4]
4-tap moving average prediction coefficients in reverse order
Definition: amrnbdata.h:1463
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
static const float hpf_zeros[2]
High-pass filters coefficients for 31 Hz and 400 Hz cutoff.
Definition: amrwbdata.h:1813
static const float ac_inter[65]
Coefficients for FIR interpolation of excitation vector at pitch lag resulting the adaptive codebook ...
Definition: amrwbdata.h:1635
float bpf_6_7_mem[HB_FIR_SIZE]
previous values in the high-band band pass filter
Definition: amrwbdec.c:84
static const float bpf_6_7_coef[31]
High-band post-processing FIR filters coefficients from Q15.
Definition: amrwbdata.h:1856
static void ff_amr_bit_reorder(uint16_t *out, int size, const uint8_t *data, const R_TABLE_TYPE *ord_table)
Fill the frame structure variables from bitstream by parsing the given reordering table that uses the...
Definition: amr.h:51
static void decode_3p_track(int *out, int code, int m, int off)
code: 3m+1 bits
Definition: amrwbdec.c:396
void(* weighted_vector_sumf)(float *out, const float *in_a, const float *in_b, float weight_coeff_a, float weight_coeff_b, int length)
float implementation of weighted sum of two vectors.
Definition: acelp_vectors.h:40
uint8_t prev_ir_filter_nr
previous impulse response filter "impNr": 0 - strong, 1 - medium, 2 - none
Definition: amrwbdec.c:75
static float voice_factor(float *p_vector, float p_gain, float *f_vector, float f_gain, CELPMContext *ctx)
Calculate the voicing factor (-1.0 = unvoiced to 1.0 = voiced).
Definition: amrwbdec.c:606
static const float isfp_inter[4]
ISF/ISP interpolation coefficients for each subframe.
Definition: amrwbdata.h:1631
static void synthesis(AMRWBContext *ctx, float *lpc, float *excitation, float fixed_gain, const float *fixed_vector, float *samples)
Conduct 16th order linear predictive coding synthesis from excitation.
Definition: amrwbdec.c:773
static void de_emphasis(float *out, float *in, float m, float mem[1])
Apply to synthesis a de-emphasis filter of the form: H(z) = 1 / (1 - m * z^-1)
Definition: amrwbdec.c:810
static float * anti_sparseness(AMRWBContext *ctx, float *fixed_vector, float *buf)
Reduce fixed vector sparseness by smoothing with one of three IR filters, also known as "adaptive pha...
Definition: amrwbdec.c:630
static void decode_1p_track(int *out, int code, int m, int off)
The next six functions decode_[i]p_track decode exactly i pulses positions and amplitudes (-1 or 1) i...
Definition: amrwbdec.c:379
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
float prev_sparse_fixed_gain
previous fixed gain; used by anti-sparseness to determine "onset"
Definition: amrwbdec.c:74
void(* celp_lp_synthesis_filterf)(float *out, const float *filter_coeffs, const float *in, int buffer_length, int filter_length)
LP synthesis filter.
Definition: celp_filters.h:45
Definition: avutil.h:144
void ff_scale_vector_to_given_sum_of_squares(float *out, const float *in, float sum_of_squares, const int n)
Set the sum of squares of a signal by scaling.
Definition: acelp_vectors.c:223
external API header
ACELPVContext acelpv_ctx
context for vector operations for ACELP-based codecs
Definition: amrwbdec.c:90
static void upsample_5_4(float *out, const float *in, int o_size, CELPMContext *ctx)
Upsample a signal by 5/4 ratio (from 12.8kHz to 16kHz) using a FIR interpolation filter.
Definition: amrwbdec.c:831
static void interpolate_isp(double isp_q[4][LP_ORDER], const double *isp4_past)
Interpolate the fourth ISP vector from current and past frames to obtain an ISP vector for each subfr...
Definition: amrwbdec.c:237
static void decode_pitch_vector(AMRWBContext *ctx, const AMRWBSubFrame *amr_subframe, const int subframe)
Find the pitch vector by interpolating the past excitation at the pitch delay, which is obtained in t...
Definition: amrwbdec.c:321
Definition: celp_filters.h:28
audio channel layout utility functions
Definition: celp_math.h:28
Definition: amrwbdec.c:47
double isp_sub4_past[LP_ORDER]
ISP vector for the 4th subframe of the previous frame.
Definition: amrwbdec.c:55
float samples_az[LP_ORDER+AMRWB_SFR_SIZE]
low-band samples and memory from synthesis at 12.8kHz
Definition: amrwbdec.c:78
float prediction_error[4]
quantified prediction errors {20log10(^gamma_gc)} for previous four subframes
Definition: amrwbdec.c:68
static void hb_synthesis(AMRWBContext *ctx, int subframe, float *samples, const float *exc, const float *isf, const float *isf_past)
Conduct 20th order linear predictive coding synthesis for the high frequency band excitation at 16kHz...
Definition: amrwbdec.c:1013
static void decode_2p_track(int *out, int code, int m, int off)
code: 2m+1 bits
Definition: amrwbdec.c:386
float pitch_gain[6]
quantified pitch gains for the current and previous five subframes
Definition: amrwbdec.c:69
void(* acelp_apply_order_2_transfer_function)(float *out, const float *in, const float zero_coeffs[2], const float pole_coeffs[2], float gain, float mem[2], int n)
Apply an order 2 rational transfer function in-place.
Definition: acelp_filters.h:47
static const uint16_t * amr_bit_orderings_by_mode[]
Reordering array addresses for each mode.
Definition: amrwbdata.h:676
static void decode_isf_indices_46b(uint16_t *ind, float *isf_q)
Decode quantized ISF vectors using 46-bit indexes (except 6K60 mode).
Definition: amrwbdec.c:183
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
void ff_celp_circ_addf(float *out, const float *in, const float *lagged, int lag, float fac, int n)
Add an array to a rotated array.
Definition: celp_filters.c:50
static void decode_fixed_vector(float *fixed_vector, const uint16_t *pulse_hi, const uint16_t *pulse_lo, const enum Mode mode)
Decode the algebraic codebook index to pulse positions and signs, then construct the algebraic codebo...
Definition: amrwbdec.c:495
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
static unsigned int av_lfg_get(AVLFG *c)
Get the next random unsigned 32-bit number using an ALFG.
Definition: lfg.h:38
float excitation_buf[AMRWB_P_DELAY_MAX+LP_ORDER+2+AMRWB_SFR_SIZE]
current excitation and all necessary excitation history
Definition: amrwbdec.c:62
static av_cold int amrwb_decode_init(AVCodecContext *avctx)
Definition: amrwbdec.c:96
void ff_acelp_lsf2lspd(double *lsp, const float *lsf, int lp_order)
Floating point version of ff_acelp_lsf2lsp()
Definition: lsp.c:93
static float noise_enhancer(float fixed_gain, float *prev_tr_gain, float voice_fac, float stab_fac)
Apply a non-linear fixed gain smoothing in order to reduce fluctuation in the energy of excitation...
Definition: amrwbdec.c:718
static float auto_correlation(float *diff_isf, float mean, int lag)
Calculate the auto-correlation for the ISF difference vector.
Definition: amrwbdec.c:907
static void update_sub_state(AMRWBContext *ctx)
Update context state before the next subframe.
Definition: amrwbdec.c:1076
void ff_acelp_vectors_init(ACELPVContext *c)
Initialize ACELPVContext.
Definition: acelp_vectors.c:266
void avpriv_report_missing_feature(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
static void decode_pitch_lag_high(int *lag_int, int *lag_frac, int pitch_index, uint8_t *base_lag_int, int subframe)
Decode an adaptive codebook index into pitch lag (except 6k60, 8k85 modes).
Definition: amrwbdec.c:259
static float find_hb_gain(AMRWBContext *ctx, const float *synth, uint16_t hb_idx, uint8_t vad)
Calculate the high-band gain based on encoded index (23k85 mode) or on the low-band speech signal and...
Definition: amrwbdec.c:863
float samples_hb[LP_ORDER_16k+AMRWB_SFR_SIZE_16k]
high-band samples and memory from synthesis at 16kHz
Definition: amrwbdec.c:80
static const float upsample_fir[4][24]
Interpolation coefficients for 5/4 signal upsampling Table from the reference source was reordered fo...
Definition: amrwbdata.h:1822
uint8_t base_pitch_lag
integer part of pitch lag for the next relative subframe
Definition: amrwbdec.c:59
static int decode_mime_header(AMRWBContext *ctx, const uint8_t *buf)
Decode the frame header in the "MIME/storage" format.
Definition: amrwbdec.c:140
common internal api header.
common internal and external API header
void ff_set_min_dist_lsf(float *lsf, double min_spacing, int size)
Adjust the quantized LSFs so they are increasing and not too close.
Definition: lsp.c:51
#define BIT_STR(x, lsb, len)
Get x bits in the index interval [lsb,lsb+len-1] inclusive.
Definition: amrwbdec.c:361
static const int16_t dico1_isf[256][9]
Indexed tables for retrieval of quantized ISF vectors in Q15.
Definition: amrwbdata.h:692
void ff_acelp_filter_init(ACELPFContext *c)
Initialize ACELPFContext.
Definition: acelp_filters.c:148
static void decode_gains(const uint8_t vq_gain, const enum Mode mode, float *fixed_gain_factor, float *pitch_gain)
Decode pitch gain and fixed gain correction factor.
Definition: amrwbdec.c:566
float fixed_vector[AMRWB_SFR_SIZE]
algebraic codebook (fixed) vector for current subframe
Definition: amrwbdec.c:66
AMR wideband data and definitions.
static void pitch_sharpening(AMRWBContext *ctx, float *fixed_vector)
Apply pitch sharpening filters to the fixed codebook vector.
Definition: amrwbdec.c:584
static const int16_t isf_init[LP_ORDER]
Initialization tables for the processed ISF vector in Q15.
Definition: amrwbdata.h:1625
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
static void decode_isf_indices_36b(uint16_t *ind, float *isf_q)
Decode quantized ISF vectors using 36-bit indexes (6K60 mode only).
Definition: amrwbdec.c:156
static const uint16_t qua_hb_gain[16]
High band quantized gains for 23k85 in Q14.
Definition: amrwbdata.h:1850
static void decode_4p_track(int *out, int code, int m, int off)
code: 4m bits
Definition: amrwbdec.c:405
float ff_amr_set_fixed_gain(float fixed_gain_factor, float fixed_mean_energy, float *prediction_error, float energy_mean, const float *pred_table)
Calculate fixed gain (part of section 6.1.3 of AMR spec)
Definition: acelp_pitch_delay.c:126
Generated on Wed Apr 16 2025 06:53:11 for FFmpeg by
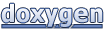