FFmpeg
|
vorbis_parser.c
Go to the documentation of this file.
int avpriv_vorbis_parse_frame(VorbisParseContext *s, const uint8_t *buf, int buf_size)
Get the duration for a Vorbis packet.
Definition: vorbis_parser.c:204
void avpriv_vorbis_parse_reset(VorbisParseContext *s)
Definition: vorbis_parser.c:237
int prev_mask
bitmask used to get the previous mode flag in each packet
Definition: vorbis_parser.h:42
struct VorbisParseContext VorbisParseContext
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: vorbis_parser.h:33
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
Vorbis audio parser.
bitstream reader API header.
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static int parse_id_header(AVCodecContext *avctx, VorbisParseContext *s, const uint8_t *buf, int buf_size)
Definition: vorbis_parser.c:33
Definition: libavcodec/avcodec.h:3883
Definition: libavcodec/avcodec.h:3747
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int parse_setup_header(AVCodecContext *avctx, VorbisParseContext *s, const uint8_t *buf, int buf_size)
Definition: vorbis_parser.c:65
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
int avpriv_split_xiph_headers(uint8_t *extradata, int extradata_size, int first_header_size, uint8_t *header_start[3], int header_len[3])
Split a single extradata buffer into the three headers that most Xiph codecs use. ...
Definition: xiph.c:24
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: get_bits.h:54
int avpriv_vorbis_parse_extradata(AVCodecContext *avctx, VorbisParseContext *s)
Initialize the Vorbis parser using headers in the extradata.
Definition: vorbis_parser.c:176
Definition: libavcodec/avcodec.h:386
Generated on Thu May 29 2025 06:52:55 for FFmpeg by
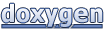