FFmpeg
|
libavcodec/flacenc.c
Go to the documentation of this file.
300 s->options.block_time_ms = ((int[]){ 27, 27, 27,105,105,105,105,105,105,105,105,105,105})[level];
310 s->options.max_prediction_order = ((int[]){ 3, 4, 4, 6, 8, 8, 8, 8, 12, 12, 12, 32, 32})[level];
313 s->options.prediction_order_method = ((int[]){ ORDER_METHOD_EST, ORDER_METHOD_EST, ORDER_METHOD_EST,
1312 { "lpc_coeff_precision", "LPC coefficient precision", offsetof(FlacEncodeContext, options.lpc_coeff_precision), AV_OPT_TYPE_INT, {.i64 = 15 }, 0, MAX_LPC_PRECISION, FLAGS },
1313 { "lpc_type", "LPC algorithm", offsetof(FlacEncodeContext, options.lpc_type), AV_OPT_TYPE_INT, {.i64 = FF_LPC_TYPE_DEFAULT }, FF_LPC_TYPE_DEFAULT, FF_LPC_TYPE_NB-1, FLAGS, "lpc_type" },
1314 { "none", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = FF_LPC_TYPE_NONE }, INT_MIN, INT_MAX, FLAGS, "lpc_type" },
1315 { "fixed", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = FF_LPC_TYPE_FIXED }, INT_MIN, INT_MAX, FLAGS, "lpc_type" },
1316 { "levinson", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = FF_LPC_TYPE_LEVINSON }, INT_MIN, INT_MAX, FLAGS, "lpc_type" },
1317 { "cholesky", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = FF_LPC_TYPE_CHOLESKY }, INT_MIN, INT_MAX, FLAGS, "lpc_type" },
1318 { "lpc_passes", "Number of passes to use for Cholesky factorization during LPC analysis", offsetof(FlacEncodeContext, options.lpc_passes), AV_OPT_TYPE_INT, {.i64 = 2 }, 1, INT_MAX, FLAGS },
1319 { "min_partition_order", NULL, offsetof(FlacEncodeContext, options.min_partition_order), AV_OPT_TYPE_INT, {.i64 = -1 }, -1, MAX_PARTITION_ORDER, FLAGS },
1320 { "max_partition_order", NULL, offsetof(FlacEncodeContext, options.max_partition_order), AV_OPT_TYPE_INT, {.i64 = -1 }, -1, MAX_PARTITION_ORDER, FLAGS },
1321 { "prediction_order_method", "Search method for selecting prediction order", offsetof(FlacEncodeContext, options.prediction_order_method), AV_OPT_TYPE_INT, {.i64 = -1 }, -1, ORDER_METHOD_LOG, FLAGS, "predm" },
1322 { "estimation", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = ORDER_METHOD_EST }, INT_MIN, INT_MAX, FLAGS, "predm" },
1323 { "2level", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = ORDER_METHOD_2LEVEL }, INT_MIN, INT_MAX, FLAGS, "predm" },
1324 { "4level", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = ORDER_METHOD_4LEVEL }, INT_MIN, INT_MAX, FLAGS, "predm" },
1325 { "8level", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = ORDER_METHOD_8LEVEL }, INT_MIN, INT_MAX, FLAGS, "predm" },
1326 { "search", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = ORDER_METHOD_SEARCH }, INT_MIN, INT_MAX, FLAGS, "predm" },
1327 { "log", NULL, 0, AV_OPT_TYPE_CONST, {.i64 = ORDER_METHOD_LOG }, INT_MIN, INT_MAX, FLAGS, "predm" },
1328 { "ch_mode", "Stereo decorrelation mode", offsetof(FlacEncodeContext, options.ch_mode), AV_OPT_TYPE_INT, { .i64 = -1 }, -1, FLAC_CHMODE_MID_SIDE, FLAGS, "ch_mode" },
1330 { "indep", NULL, 0, AV_OPT_TYPE_CONST, { .i64 = FLAC_CHMODE_INDEPENDENT }, INT_MIN, INT_MAX, FLAGS, "ch_mode" },
1331 { "left_side", NULL, 0, AV_OPT_TYPE_CONST, { .i64 = FLAC_CHMODE_LEFT_SIDE }, INT_MIN, INT_MAX, FLAGS, "ch_mode" },
1332 { "right_side", NULL, 0, AV_OPT_TYPE_CONST, { .i64 = FLAC_CHMODE_RIGHT_SIDE }, INT_MIN, INT_MAX, FLAGS, "ch_mode" },
1333 { "mid_side", NULL, 0, AV_OPT_TYPE_CONST, { .i64 = FLAC_CHMODE_MID_SIDE }, INT_MIN, INT_MAX, FLAGS, "ch_mode" },
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
uint32_t av_crc(const AVCRC *ctx, uint32_t crc, const uint8_t *buffer, size_t length)
Calculate the CRC of a block.
Definition: crc.c:275
Definition: put_bits.h:41
Definition: lpc.h:50
static uint64_t calc_optimal_rice_params(RiceContext *rc, int porder, uint64_t *sums, int n, int pred_order)
Definition: libavcodec/flacenc.c:583
Definition: libavcodec/flacenc.c:95
int ff_lpc_calc_coefs(LPCContext *s, const int32_t *samples, int blocksize, int min_order, int max_order, int precision, int32_t coefs[][MAX_LPC_ORDER], int *shift, enum FFLPCType lpc_type, int lpc_passes, int omethod, int max_shift, int zero_shift)
Calculate LPC coefficients for multiple orders.
Definition: lpc.c:170
Definition: flac.h:40
Definition: opt.h:222
av_cold void ff_flacdsp_init(FLACDSPContext *c, enum AVSampleFormat fmt, int bps)
Definition: flacdsp.c:88
int ff_flac_get_max_frame_size(int blocksize, int ch, int bps)
Calculate an estimate for the maximum frame size based on verbatim mode.
Definition: flac.c:148
Definition: libavcodec/flacenc.c:53
Definition: libavcodec/avcodec.h:393
#define PUT_UTF8(val, tmp, PUT_BYTE)
Convert a 32-bit Unicode character to its UTF-8 encoded form (up to 4 bytes long).
Definition: common.h:349
void(* bswap16_buf)(uint16_t *dst, const uint16_t *src, int len)
Definition: dsputil.h:209
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
static int flac_encode_frame(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Definition: libavcodec/flacenc.c:1229
static uint64_t calc_rice_params(RiceContext *rc, int pmin, int pmax, int32_t *data, int n, int pred_order)
Definition: libavcodec/flacenc.c:636
static uint64_t rice_count_exact(int32_t *res, int n, int k)
Definition: libavcodec/flacenc.c:504
Definition: libavcodec/flacenc.c:50
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
static int select_blocksize(int samplerate, int block_time_ms)
Set blocksize based on samplerate.
Definition: libavcodec/flacenc.c:151
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
Definition: opt.h:229
Definition: crc.h:31
Definition: samplefmt.h:50
struct CompressionOptions CompressionOptions
AVOptions.
const AVCRC * av_crc_get_table(AVCRCId crc_id)
Get an initialized standard CRC table.
Definition: crc.c:261
Definition: flac.h:41
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
static uint64_t find_subframe_rice_params(FlacEncodeContext *s, FlacSubframe *sub, int pred_order)
Definition: libavcodec/flacenc.c:682
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
static void write_subframes(FlacEncodeContext *s)
Definition: libavcodec/flacenc.c:1110
static int estimate_stereo_mode(int32_t *left_ch, int32_t *right_ch, int n, int max_rice_param)
Definition: libavcodec/flacenc.c:977
FLAC (Free Lossless Audio Codec) decoder/demuxer common functions.
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
#define CODEC_CAP_SMALL_LAST_FRAME
Codec can be fed a final frame with a smaller size.
Definition: libavcodec/avcodec.h:775
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
#define COPY_SAMPLES(bits)
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
static void remove_wasted_bits(FlacEncodeContext *s)
Definition: libavcodec/flacenc.c:945
Definition: alacenc.c:46
struct FlacSubframe FlacSubframe
void(* lpc_encode)(int32_t *res, const int32_t *smp, int len, int order, const int32_t *coefs, int shift)
Definition: flacdsp.h:30
struct RiceContext RiceContext
static int encode_residual_ch(FlacEncodeContext *s, int ch)
Definition: libavcodec/flacenc.c:750
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
Definition: flac.h:43
int prediction_order_method
Definition: libavcodec/flacenc.c:61
static int get_max_p_order(int max_porder, int n, int order)
Definition: libavcodec/flacenc.c:673
static int write_frame(FlacEncodeContext *s, AVPacket *avpkt)
Definition: libavcodec/flacenc.c:1181
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Return number of bytes per sample.
Definition: samplefmt.c:104
static void set_sr_golomb_flac(PutBitContext *pb, int i, int k, int limit, int esc_len)
write signed golomb rice code (flac).
Definition: golomb.h:548
static void channel_decorrelation(FlacEncodeContext *s)
Perform stereo channel decorrelation.
Definition: libavcodec/flacenc.c:1021
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
struct FlacEncodeContext FlacEncodeContext
static void write_frame_header(FlacEncodeContext *s)
Definition: libavcodec/flacenc.c:1073
static int count_frame_header(FlacEncodeContext *s)
Definition: libavcodec/flacenc.c:889
Definition: libavcodec/flacenc.c:49
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
struct FlacFrame FlacFrame
static void encode_residual_fixed(int32_t *res, const int32_t *smp, int n, int order)
Definition: libavcodec/flacenc.c:699
int max_encoded_framesize
Definition: libavcodec/flacenc.c:105
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: libavcodec/flacenc.c:73
static void write_utf8(PutBitContext *pb, uint32_t val)
Definition: libavcodec/flacenc.c:1066
av_cold int ff_lpc_init(LPCContext *s, int blocksize, int max_order, enum FFLPCType lpc_type)
Initialize LPCContext.
Definition: lpc.c:258
static void copy_samples(FlacEncodeContext *s, const void *samples)
Copy channel-interleaved input samples into separate subframes.
Definition: libavcodec/flacenc.c:482
static void write_frame_footer(FlacEncodeContext *s)
Definition: libavcodec/flacenc.c:1170
common internal api header.
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
Definition: crc.h:32
static void calc_sums(int pmin, int pmax, uint32_t *data, int n, int pred_order, uint64_t sums[][MAX_PARTITIONS])
Definition: libavcodec/flacenc.c:609
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
static av_cold int flac_encode_close(AVCodecContext *avctx)
Definition: libavcodec/flacenc.c:1297
static av_cold void dprint_compression_options(FlacEncodeContext *s)
Definition: libavcodec/flacenc.c:170
DSP utils.
static int find_optimal_param(uint64_t sum, int n, int max_param)
Solve for d/dk(rice_encode_count) = n-((sum-(n>>1))>>(k+1)) = 0.
Definition: libavcodec/flacenc.c:570
static uint64_t subframe_count_exact(FlacEncodeContext *s, FlacSubframe *sub, int pred_order)
Definition: libavcodec/flacenc.c:518
Definition: flacdsp.h:25
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
static void init_frame(FlacEncodeContext *s, int nb_samples)
Definition: libavcodec/flacenc.c:437
Definition: flac.h:42
static av_always_inline int64_t ff_samples_to_time_base(AVCodecContext *avctx, int64_t samples)
Rescale from sample rate to AVCodecContext.time_base.
Definition: libavcodec/internal.h:186
static int update_md5_sum(FlacEncodeContext *s, const void *samples)
Definition: libavcodec/flacenc.c:1191
exp golomb vlc stuff
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
static av_cold int flac_encode_init(AVCodecContext *avctx)
Definition: libavcodec/flacenc.c:228
static void write_streaminfo(FlacEncodeContext *s, uint8_t *header)
Write streaminfo metadata block to byte array.
Definition: libavcodec/flacenc.c:124
Definition: libavcodec/flacenc.c:86
trying all byte sequences megabyte in length and selecting the best looking sequence will yield cases to try But a word about which is also called distortion Distortion can be quantified by almost any quality measurement one chooses the sum of squared differences is used but more complex methods that consider psychovisual effects can be used as well It makes no difference in this discussion First step
Definition: rate_distortion.txt:12
bitstream writer API
Generated on Mon Aug 4 2025 06:54:20 for FFmpeg by
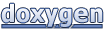