FFmpeg
|
libavcodec/g729dec.c
Go to the documentation of this file.
122 int16_t syn_filter_data[10];
135 int16_t past_gain_pitch[6];
138 int16_t past_gain_code[2];
154 int16_t hpf_z[2];
310 static int16_t g729d_voice_decision(int onset, int prev_voice_decision, const int16_t* past_gain_pitch)
353 av_log(avctx, AV_LOG_ERROR, "Only mono sound is supported (requested channels: %d).\n", avctx->channels);
637 ctx->voice_decision = g729d_voice_decision(ctx->onset, ctx->voice_decision, ctx->past_gain_pitch);
639 g729d_get_new_exc(exc_new, ctx->exc + i * SUBFRAME_SIZE, fc, ctx->voice_decision, ctx->past_gain_code[0], SUBFRAME_SIZE);
711 memmove(ctx->exc_base, ctx->exc_base + 2 * SUBFRAME_SIZE, (PITCH_DELAY_MAX+INTERPOL_LEN)*sizeof(int16_t));
void ff_acelp_high_pass_filter(int16_t *out, int hpf_f[2], const int16_t *in, int length)
high-pass filtering and upscaling (4.2.5 of G.729).
Definition: acelp_filters.c:99
void ff_acelp_fc_pulse_per_track(int16_t *fc_v, const uint8_t *tab1, const uint8_t *tab2, int pulse_indexes, int pulse_signs, int pulse_count, int bits)
Decode fixed-codebook vector (3.8 and D.5.8 of G.729, 5.7.1 of AMR).
Definition: acelp_vectors.c:127
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
static const int16_t cb_ma_predictor[2][MA_NP][10]
4th order Moving Average (MA) Predictor codebook (3.2.4 of G.729)
Definition: g729data.h:300
#define LSFQ_MIN
minimum quantized LSF value (3.2.4) 0.005 in Q13
Definition: libavcodec/g729dec.c:46
static const int16_t cb_gain_1st_6k4[1<< GC_1ST_IDX_BITS_6K4][2]
gain codebook (first stage), 6.4k mode (D.3.9.2 of G.729)
Definition: g729data.h:251
void ff_acelp_lsf2lsp(int16_t *lsp, const int16_t *lsf, int lp_order)
Convert LSF to LSP.
Definition: lsp.c:83
int16_t res_filter_data[SUBFRAME_SIZE+10]
previous speech data for residual calculation filter
Definition: libavcodec/g729dec.c:129
#define GC_2ND_IDX_BITS_8K
gain codebook (second stage) index, 8k mode (size in bits)
Definition: g729data.h:33
static const uint16_t ma_prediction_coeff[4]
MA prediction coefficients (3.9.1 of G.729, near Equation 69)
Definition: g729data.h:343
const int16_t ff_acelp_interp_filter[61]
low-pass Finite Impulse Response filter coefficients.
Definition: acelp_filters.c:30
#define MR_ENERGY
MR_ENERGY (mean removed energy) = mean_energy + 10 * log10(2^26 * subframe_size) in (7...
Definition: libavcodec/g729dec.c:81
int ff_celp_lp_synthesis_filter(int16_t *out, const int16_t *filter_coeffs, const int16_t *in, int buffer_length, int filter_length, int stop_on_overflow, int shift, int rounder)
LP synthesis filter.
Definition: celp_filters.c:60
const uint8_t ff_fc_2pulses_9bits_track1_gray[16]
Definition: acelp_vectors.c:41
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
int ff_acelp_decode_4bit_to_2nd_delay3(int ac_index, int pitch_delay_min)
Decode pitch delay with 1/3 precision.
Definition: acelp_pitch_delay.c:39
void ff_acelp_reorder_lsf(int16_t *lsfq, int lsfq_min_distance, int lsfq_min, int lsfq_max, int lp_order)
(I.F) means fixed-point value with F fractional and I integer bits
Definition: lsp.c:33
external API header
int ff_acelp_decode_8bit_to_1st_delay3(int ac_index)
Decode pitch delay of the first subframe encoded by 8 bits with 1/3 resolution.
Definition: acelp_pitch_delay.c:31
int16_t lsp_buf[2][10]
(0.15) LSP coefficients (previous and current frames) (3.2.5)
Definition: libavcodec/g729dec.c:116
int16_t past_quantizer_output_buf[MA_NP+1][10]
(2.13) LSP quantizer outputs
Definition: libavcodec/g729dec.c:112
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
static int32_t scalarproduct_int16_c(const int16_t *v1, const int16_t *v2, int order)
Definition: libavcodec/g729dec.c:337
uint8_t fc_indexes_bits
size (in bits) of fixed-codebook index entry
Definition: libavcodec/g729dec.c:99
const uint8_t ff_fc_4pulses_8bits_track_4[32]
Track|Pulse| Positions 4 | 3 | 3, 8, 13, 18, 23, 28, 33, 38, 43, 48, 53, 58, 63, 68, 73, 78 | | 4, 9, 14, 19, 24, 29, 34, 39, 44, 49, 54, 59, 64, 69, 74, 79
Definition: acelp_vectors.c:78
static void g729d_get_new_exc(int16_t *out, const int16_t *in, const int16_t *fc_cur, int dstate, int gain_code, int subframe_size)
Constructs new excitation signal and applies phase filter to it.
Definition: libavcodec/g729dec.c:266
Definition: libavcodec/g729dec.c:88
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
int ff_acelp_decode_5_6_bit_to_2nd_delay3(int ac_index, int pitch_delay_min)
Decode pitch delay of the second subframe encoded by 5 or 6 bits with 1/3 precision.
Definition: acelp_pitch_delay.c:51
int16_t past_gain_code[2]
(14.1) gain code from current and previous subframe
Definition: libavcodec/g729dec.c:138
bitstream reader API header.
Definition: libavcodec/g729dec.c:90
static int16_t g729d_voice_decision(int onset, int prev_voice_decision, const int16_t *past_gain_pitch)
Makes decision about voice presence in current subframe.
Definition: libavcodec/g729dec.c:310
int16_t ff_g729_adaptive_gain_control(int gain_before, int gain_after, int16_t *speech, int subframe_size, int16_t gain_prev)
Adaptive gain control (4.2.4)
Definition: g729postfilter.c:574
int16_t * past_quantizer_outputs[MA_NP+1]
Definition: libavcodec/g729dec.c:113
static void lsf_decode(int16_t *lsfq, int16_t *past_quantizer_outputs[MA_NP+1], int16_t ma_predictor, int16_t vq_1st, int16_t vq_2nd_low, int16_t vq_2nd_high)
Decodes LSF (Line Spectral Frequencies) from L0-L3 (3.2.4).
Definition: libavcodec/g729dec.c:200
uint8_t gc_2nd_index_bits
gain codebook (second stage) index (size in bits)
Definition: libavcodec/g729dec.c:97
Definition: libavcodec/g729dec.c:93
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
int16_t lsfq[10]
(2.13) quantized LSF coefficients from previous frame
Definition: libavcodec/g729dec.c:115
Definition: avutil.h:144
void ff_celp_convolve_circ(int16_t *fc_out, const int16_t *fc_in, const int16_t *filter, int len)
Circularly convolve fixed vector with a phase dispersion impulse response filter (D.6.2 of G.729 and 6.1.5 of AMR).
Definition: celp_filters.c:30
static av_cold int decoder_init(AVCodecContext *avctx)
Definition: libavcodec/g729dec.c:347
static const int16_t cb_gain_1st_8k[1<< GC_1ST_IDX_BITS_8K][2]
gain codebook (first stage), 8k mode (3.9.2 of G.729)
Definition: g729data.h:215
uint8_t ac_index_bits[2]
adaptive codebook index for second subframe (size in bits)
Definition: libavcodec/g729dec.c:94
external API header
static const int16_t cb_lsp_2nd[1<< VQ_2ND_BITS][10]
second stage LSP codebook, high and low parts (both 5-dimensional, with 32 entries (3...
Definition: g729data.h:177
void ff_acelp_weighted_vector_sum(int16_t *out, const int16_t *in_a, const int16_t *in_b, int16_t weight_coeff_a, int16_t weight_coeff_b, int16_t rounder, int shift, int length)
weighted sum of two vectors with rounding.
Definition: acelp_vectors.c:172
#define GC_2ND_IDX_BITS_6K4
gain codebook (second stage) index, 6.4k mode (size in bits)
Definition: g729data.h:36
int16_t residual[SUBFRAME_SIZE+RES_PREV_DATA_SIZE]
residual signal buffer (used in long-term postfilter)
Definition: libavcodec/g729dec.c:126
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
int pitch_delay_int_prev
integer part of previous subframe's pitch delay (4.1.3)
Definition: libavcodec/g729dec.c:109
void ff_acelp_update_past_gain(int16_t *quant_energy, int gain_corr_factor, int log2_ma_pred_order, int erasure)
Update past quantized energies.
Definition: acelp_pitch_delay.c:72
void ff_acelp_lp_decode(int16_t *lp_1st, int16_t *lp_2nd, const int16_t *lsp_2nd, const int16_t *lsp_prev, int lp_order)
Interpolate LSP for the first subframe and convert LSP -> LP for both subframes (3.2.5 and 3.2.6 of G.729)
Definition: lsp.c:171
int ma_predictor_prev
switched MA predictor of LSP quantizer from last good frame
Definition: libavcodec/g729dec.c:148
int16_t ff_acelp_decode_gain_code(DSPContext *dsp, int gain_corr_factor, const int16_t *fc_v, int mr_energy, const int16_t *quant_energy, const int16_t *ma_prediction_coeff, int subframe_size, int ma_pred_order)
Decode the adaptive codebook gain and add correction (4.1.5 and 3.9.1 of G.729).
Definition: acelp_pitch_delay.c:93
void ff_g729_postfilter(DSPContext *dsp, int16_t *ht_prev_data, int *voicing, const int16_t *lp_filter_coeffs, int pitch_delay_int, int16_t *residual, int16_t *res_filter_data, int16_t *pos_filter_data, int16_t *speech, int subframe_size)
Signal postfiltering (4.2)
Definition: g729postfilter.c:514
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
#define SHARP_MIN
minimum gain pitch value (3.8, Equation 47) 0.2 in (1.14)
Definition: libavcodec/g729dec.c:67
static void lsf_restore_from_previous(int16_t *lsfq, int16_t *past_quantizer_outputs[MA_NP+1], int ma_predictor_prev)
Restores past LSP quantizer output using LSF from previous frame.
Definition: libavcodec/g729dec.c:241
uint8_t gc_1st_index_bits
gain codebook (first stage) index (size in bits)
Definition: libavcodec/g729dec.c:96
static int decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: libavcodec/g729dec.c:390
int16_t past_gain_pitch[6]
(1.14) pitch gain of current and five previous subframes
Definition: libavcodec/g729dec.c:135
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
const uint8_t ff_fc_4pulses_8bits_tracks_13[16]
Track|Pulse| Positions 1 | 0 | 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70, 75 2 | 1 | 1, 6, 11, 16, 21, 26, 31, 36, 41, 46, 51, 56, 61, 66, 71, 76 3 | 2 | 2, 7, 12, 17, 22, 27, 32, 37, 42, 47, 52, 57, 62, 67, 72, 77
Definition: acelp_vectors.c:73
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
int16_t was_periodic
whether previous frame was declared as periodic or not (4.4)
Definition: libavcodec/g729dec.c:144
int32_t(* scalarproduct_int16)(const int16_t *v1, const int16_t *v2, int len)
Calculate scalar product of two vectors.
Definition: dsputil.h:274
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: libavcodec/g729dec.c:102
const uint8_t ff_fc_2pulses_9bits_track2_gray[32]
Track|Pulse| Positions 2 | 1 | 0, 7, 14, 20, 27, 34, 1, 21 | | 2, 9, 15, 22, 29, 35, 6, 26 | | 4,10, 17, 24, 30, 37, 11, 31 | | 5,12, 19, 25, 32, 39, 16, 36
Definition: acelp_vectors.c:53
#define LSFQ_MAX
maximum quantized LSF value (3.2.4) 3.135 in Q13
Definition: libavcodec/g729dec.c:52
#define SHARP_MAX
maximum gain pitch value (3.8, Equation 47) (EE) This does not comply with the specification.
Definition: libavcodec/g729dec.c:76
void ff_acelp_interpolate(int16_t *out, const int16_t *in, const int16_t *filter_coeffs, int precision, int frac_pos, int filter_length, int length)
Generic FIR interpolation routine.
Definition: acelp_filters.c:44
#define GC_1ST_IDX_BITS_8K
gain codebook (first stage) index, 8k mode (size in bits)
Definition: g729data.h:32
Definition: get_bits.h:54
common internal api header.
static const int16_t cb_gain_2nd_6k4[1<< GC_2ND_IDX_BITS_6K4][2]
gain codebook (second stage), 6.4k mode (D.3.9.2 of G.729)
Definition: g729data.h:266
int16_t syn_filter_data[10]
previous speech data for LP synthesis filter
Definition: libavcodec/g729dec.c:122
static int g729d_onset_decision(int past_onset, const int16_t *past_gain_code)
Makes decision about onset in current subframe.
Definition: libavcodec/g729dec.c:294
int16_t exc_base[2 *SUBFRAME_SIZE+PITCH_DELAY_MAX+INTERPOL_LEN]
past excitation signal buffer
Definition: libavcodec/g729dec.c:106
DSP utils.
#define GC_1ST_IDX_BITS_6K4
gain codebook (first stage) index, 6.4k mode (size in bits)
Definition: g729data.h:35
static const int16_t cb_ma_predictor_sum[2][10]
15 3 cb_ma_predictor_sum[j][i] = floor( 2 * (1...
Definition: g729data.h:321
static uint16_t g729_prng(uint16_t value)
pseudo random number generator
Definition: libavcodec/g729dec.c:178
#define RES_PREV_DATA_SIZE
Amount of past residual signal data stored in buffer.
Definition: g729postfilter.h:76
int16_t voice_decision
voice decision on previous subframe (0-noise, 1-intermediate, 2-voice), G.729D
Definition: libavcodec/g729dec.c:141
static const G729FormatDescription format_g729d_6k4
Definition: libavcodec/g729dec.c:166
int16_t pos_filter_data[SUBFRAME_SIZE+10]
previous speech data for short-term postfilter
Definition: libavcodec/g729dec.c:132
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
static const int16_t lsp_init[10]
initial LSP coefficients belongs to virtual frame preceding the first frame of the stream ...
Definition: g729data.h:351
static int get_parity(uint8_t value)
Get parity bit of bit 2..7.
Definition: libavcodec/g729dec.c:186
static const int16_t cb_gain_2nd_8k[1<< GC_2ND_IDX_BITS_8K][2]
gain codebook (second stage), 8k mode (3.9.2 of G.729)
Definition: g729data.h:229
static const int16_t cb_lsp_1st[1<< VQ_1ST_BITS][10]
first stage LSP codebook (10-dimensional, with 128 entries (3.24 of G.729)
Definition: g729data.h:42
Definition: libavcodec/avcodec.h:435
Definition: libavcodec/g729dec.c:89
static const int16_t phase_filter[3][40]
additional "phase" post-processing filter impulse response (D.6.2 of G.729)
Definition: g729data.h:361
Generated on Wed Apr 16 2025 06:53:14 for FFmpeg by
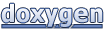