FFmpeg
|
g729postfilter.c
Go to the documentation of this file.
130 int16_t gain_long_num,gain_long_den; //filtered through long interpolation filter signal's gain numerator and denominator
376 selected_signal_const = residual + RES_PREV_DATA_SIZE - (best_delay_int + 1 - delayed_signal_offset);
static int16_t get_tilt_comp(DSPContext *dsp, int16_t *lp_gn, const int16_t *lp_gd, int16_t *speech, int subframe_size)
Calculate reflection coefficient for tilt compensation filter (4.2.3).
Definition: g729postfilter.c:424
int ff_celp_lp_synthesis_filter(int16_t *out, const int16_t *filter_coeffs, const int16_t *in, int buffer_length, int filter_length, int stop_on_overflow, int shift, int rounder)
LP synthesis filter.
Definition: celp_filters.c:60
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
#define ANALYZED_FRAC_DELAYS
Number of analyzed fractional pitch delays in second stage of long-term postfilter.
Definition: g729postfilter.h:71
static void residual_filter(int16_t *out, const int16_t *filter_coeffs, const int16_t *in, int subframe_size)
Residual signal calculation (4.2.1 if G.729)
Definition: g729postfilter.c:86
static const int16_t ff_g729_interp_filt_long[(ANALYZED_FRAC_DELAYS+1)*LONG_INT_FILT_LEN]
long interpolation filter (of length 129, according to spec) for computing signal with non-integer de...
Definition: g729postfilter.c:49
static const int16_t formant_pp_factor_num_pow[10]
formant_pp_factor_num_pow[i] = FORMANT_PP_FACTOR_NUM^(i+1)
Definition: g729postfilter.c:63
int16_t ff_g729_adaptive_gain_control(int gain_before, int gain_after, int16_t *speech, int subframe_size, int16_t gain_prev)
Adaptive gain control (4.2.4)
Definition: g729postfilter.c:574
static const int16_t ff_g729_interp_filt_short[(ANALYZED_FRAC_DELAYS+1)*SHORT_INT_FILT_LEN]
short interpolation filter (of length 33, according to spec) for computing signal with non-integer de...
Definition: g729postfilter.c:40
static int16_t apply_tilt_comp(int16_t *out, int16_t *res_pst, int refl_coeff, int subframe_size, int16_t ht_prev_data)
Apply tilt compensation filter (4.2.3).
Definition: g729postfilter.c:476
external API header
void ff_acelp_weighted_vector_sum(int16_t *out, const int16_t *in_a, const int16_t *in_b, int16_t weight_coeff_a, int16_t weight_coeff_b, int16_t rounder, int shift, int length)
weighted sum of two vectors with rounding.
Definition: acelp_vectors.c:172
#define MIN_LT_FILT_FACTOR_A
1.0 / (1.0 + 0.5) in Q15 where 0.5 is the minimum value of weight factor, controlling amount of long-...
Definition: g729postfilter.h:55
#define G729_AGC_FACTOR
gain adjustment factor (G.729, 4.2.4) 0.9875 in Q15
Definition: g729postfilter.h:47
static const int16_t formant_pp_factor_den_pow[10]
formant_pp_factor_den_pow[i] = FORMANT_PP_FACTOR_DEN^(i+1)
Definition: g729postfilter.c:71
void ff_g729_postfilter(DSPContext *dsp, int16_t *ht_prev_data, int *voicing, const int16_t *lp_filter_coeffs, int pitch_delay_int, int16_t *residual, int16_t *res_filter_data, int16_t *pos_filter_data, int16_t *speech, int subframe_size)
Signal postfiltering (4.2)
Definition: g729postfilter.c:514
static int16_t long_term_filter(DSPContext *dsp, int pitch_delay_int, const int16_t *residual, int16_t *residual_filt, int subframe_size)
long-term postfilter (4.2.1)
Definition: g729postfilter.c:110
int32_t(* scalarproduct_int16)(const int16_t *v1, const int16_t *v2, int len)
Calculate scalar product of two vectors.
Definition: dsputil.h:274
#define G729_TILT_FACTOR_PLUS
tilt compensation factor (G.729, k1>0) 0.2 in Q15
Definition: g729postfilter.h:31
void ff_acelp_interpolate(int16_t *out, const int16_t *in, const int16_t *filter_coeffs, int precision, int frac_pos, int filter_length, int length)
Generic FIR interpolation routine.
Definition: acelp_filters.c:44
static int bidir_sal(int value, int offset)
Shift value left or right depending on sign of offset parameter.
Definition: celp_math.h:71
#define RES_PREV_DATA_SIZE
Amount of past residual signal data stored in buffer.
Definition: g729postfilter.h:76
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
#define G729_TILT_FACTOR_MINUS
tilt compensation factor (G.729, k1<0) 0.9 in Q15
Definition: g729postfilter.h:37
Generated on Mon Nov 18 2024 06:51:55 for FFmpeg by
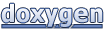