FFmpeg
|
mlp_parser.c
Go to the documentation of this file.
int channels_thd_stream1
Channel count for substream 1 of TrueHD streams ("6-channel presentation")
Definition: mlp_parser.h:44
Definition: libavcodec/avcodec.h:410
struct MLPParseContext MLPParseContext
uint16_t ff_mlp_checksum16(const uint8_t *buf, unsigned int buf_size)
Definition: mlp.c:64
Definition: parser.h:28
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
Definition: mlp_parser.h:32
static int mlp_parse(AVCodecParserContext *s, AVCodecContext *avctx, const uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size)
Definition: mlp_parser.c:220
bitstream reader API header.
Definition: libavcodec/avcodec.h:426
int ff_combine_frame(ParseContext *pc, int next, const uint8_t **buf, int *buf_size)
Combine the (truncated) bitstream to a complete frame.
Definition: parser.c:214
Definition: libavcodec/avcodec.h:3883
audio channel layout utility functions
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
int overread
the number of bytes which where irreversibly read from the next frame
Definition: parser.h:35
MLP parser prototypes.
Definition: libavcodec/avcodec.h:3747
int access_unit_size_pow2
Next power of two above number of samples per frame.
Definition: mlp_parser.h:51
Definition: mlp_parser.c:203
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
int channels_thd_stream2
Channel count for substream 2 of TrueHD streams ("8-channel presentation")
Definition: mlp_parser.h:45
Definition: get_bits.h:54
uint64_t channel_layout_thd_stream1
Channel layout for substream 1 of TrueHD streams ("6-channel presentation")
Definition: mlp_parser.h:47
uint64_t channel_layout_thd_stream2
Channel layout for substream 2 of TrueHD streams ("8-channel presentation")
Definition: mlp_parser.h:48
int ff_mlp_read_major_sync(void *log, MLPHeaderInfo *mh, GetBitContext *gb)
Read a major sync info header - contains high level information about the stream - sample rate...
Definition: mlp_parser.c:127
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel layout
Definition: filter_design.txt:12
uint64_t request_channel_layout
Request decoder to use this channel layout if it can (0 for default)
Definition: libavcodec/avcodec.h:1929
Generated on Tue Jul 8 2025 06:56:48 for FFmpeg by
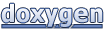