FFmpeg
|
libavresample/utils.c
Go to the documentation of this file.
int ff_audio_data_realloc(AudioData *a, int nb_samples)
Reallocate AudioData.
Definition: audio_data.c:153
int input_map[AVRESAMPLE_MAX_CHANNELS]
dest index of each input channel
Definition: libavresample/internal.h:50
Audio buffer used for intermediate storage between conversion phases.
Definition: oss_audio.c:46
memory handling functions
int ff_audio_data_add_to_fifo(AVAudioFifo *af, AudioData *a, int offset, int nb_samples)
Add samples in AudioData to an AVAudioFifo.
Definition: audio_data.c:342
AudioData * ff_audio_data_alloc(int channels, int nb_samples, enum AVSampleFormat sample_fmt, const char *name)
Allocate AudioData.
Definition: audio_data.c:110
int avresample_read(AVAudioResampleContext *avr, uint8_t **output, int nb_samples)
Read samples from the output FIFO.
Definition: libavresample/utils.c:614
double * mix_matrix
mix matrix only used if avresample_set_matrix() is called before avresample_open() ...
Definition: libavresample/internal.h:103
int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in)
Convert audio data from one sample format to another.
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
Definition: libavresample/internal.h:38
int avresample_set_channel_mapping(AVAudioResampleContext *avr, const int *channel_map)
Set a customized input channel mapping.
Definition: libavresample/utils.c:558
int ff_audio_mix(AudioMix *am, AudioData *src)
Apply channel mixing to audio data using the current mixing matrix.
Definition: audio_mix.c:428
void avresample_free(AVAudioResampleContext **avr)
Free AVAudioResampleContext and associated AVOption values.
Definition: libavresample/utils.c:273
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
int av_audio_fifo_read(AVAudioFifo *af, void **data, int nb_samples)
Read data from an AVAudioFifo.
Definition: audio_fifo.c:139
Public dictionary API.
Definition: samplefmt.h:50
AVOptions.
Definition: libavresample/internal.h:37
static int handle_buffered_output(AVAudioResampleContext *avr, AudioData *output, AudioData *converted)
Definition: libavresample/utils.c:282
#define LICENSE_PREFIX
int ff_audio_mix_set_matrix(AudioMix *am, const double *matrix, int stride)
Set the current mixing matrix.
Definition: audio_mix.c:650
enum AVSampleFormat av_get_planar_sample_fmt(enum AVSampleFormat sample_fmt)
Get the planar alternative form of the given sample format.
Definition: samplefmt.c:82
const char * avresample_license(void)
Return the libavresample license.
Definition: libavresample/utils.c:626
int av_audio_fifo_size(AVAudioFifo *af)
Get the current number of samples in the AVAudioFifo available for reading.
Definition: audio_fifo.c:186
AudioConvert * ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map)
Allocate and initialize AudioConvert context for sample format conversion.
void avresample_close(AVAudioResampleContext *avr)
Close AVAudioResampleContext.
Definition: libavresample/utils.c:257
int ff_audio_data_set_channels(AudioData *a, int channels)
Definition: audio_data.c:51
int ff_audio_resample(ResampleContext *c, AudioData *dst, AudioData *src)
Resample audio data.
Definition: libavresample/resample.c:409
Definition: libavresample/internal.h:43
AVAudioFifo * av_audio_fifo_alloc(enum AVSampleFormat sample_fmt, int channels, int nb_samples)
Allocate an AVAudioFifo.
Definition: audio_fifo.c:60
int out_convert_needed
output sample format conversion is needed
Definition: libavresample/internal.h:85
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several the filter must be ready for frames arriving randomly on any input any filter with several inputs will most likely require some kind of queuing mechanism It is perfectly acceptable to have a limited queue and to drop frames when the inputs are too unbalanced request_frame This method is called when a frame is wanted on an output For an input
Definition: filter_design.txt:216
AudioConvert * ac_out
output sample format conversion context
Definition: libavresample/internal.h:94
int ff_audio_data_read_from_fifo(AVAudioFifo *af, AudioData *a, int nb_samples)
Read samples from an AVAudioFifo to AudioData.
Definition: audio_data.c:357
int channel_copy[AVRESAMPLE_MAX_CHANNELS]
dest index to copy from
Definition: libavresample/internal.h:46
enum RemapPoint remap_point
Definition: libavresample/internal.h:106
external API header
int ff_audio_data_init(AudioData *a, uint8_t **src, int plane_size, int channels, int nb_samples, enum AVSampleFormat sample_fmt, int read_only, const char *name)
Initialize AudioData using a given source.
Definition: audio_data.c:65
ChannelMapInfo ch_map_info
Definition: libavresample/internal.h:107
const char * av_get_sample_fmt_name(enum AVSampleFormat sample_fmt)
Return the name of sample_fmt, or NULL if sample_fmt is not recognized.
Definition: samplefmt.c:47
int avresample_set_matrix(AVAudioResampleContext *avr, const double *matrix, int stride)
Set channel mixing matrix.
Definition: libavresample/utils.c:527
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Return number of bytes per sample.
Definition: samplefmt.c:104
int avresample_get_matrix(AVAudioResampleContext *avr, double *matrix, int stride)
Get the current channel mixing matrix.
Definition: libavresample/utils.c:498
int avresample_available(AVAudioResampleContext *avr)
Return the number of available samples in the output FIFO.
Definition: libavresample/utils.c:609
void ff_audio_resample_free(ResampleContext **c)
Free a ResampleContext.
Definition: libavresample/resample.c:238
Definition: libavresample/internal.h:39
enum AVSampleFormat internal_sample_fmt
internal sample format
Definition: libavresample/internal.h:62
const char * avresample_configuration(void)
Return the libavresample build-time configuration.
Definition: libavresample/utils.c:632
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
ResampleContext * ff_audio_resample_init(AVAudioResampleContext *avr)
Allocate and initialize a ResampleContext.
Definition: libavresample/resample.c:146
int av_sample_fmt_is_planar(enum AVSampleFormat sample_fmt)
Check if the sample format is planar.
Definition: samplefmt.c:118
int av_audio_fifo_drain(AVAudioFifo *af, int nb_samples)
Drain data from an AVAudioFifo.
Definition: audio_fifo.c:159
Definition: libavresample/internal.h:40
The official guide to swscale for confused that consecutive non overlapping rectangles of slice_bottom special converter These generally are unscaled converters of common like for each output line the vertical scaler pulls lines from a ring buffer When the ring buffer does not contain the wanted then it is pulled from the input slice through the input converter and horizontal scaler The result is also stored in the ring buffer to serve future vertical scaler requests When no more output can be generated because lines from a future slice would be then all remaining lines in the current slice are converted
Definition: swscale.txt:33
int attribute_align_arg avresample_convert(AVAudioResampleContext *avr, uint8_t **output, int out_plane_size, int out_samples, uint8_t **input, int in_plane_size, int in_samples)
Convert input samples and write them to the output FIFO.
Definition: libavresample/utils.c:325
int in_convert_needed
input sample format conversion is needed
Definition: libavresample/internal.h:84
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: libavresample/internal.h:36
int channel_map[AVRESAMPLE_MAX_CHANNELS]
source index of each output channel, -1 if not remapped
Definition: libavresample/internal.h:44
int ff_audio_data_copy(AudioData *dst, AudioData *src, ChannelMapInfo *map)
Copy data from one AudioData to another.
Definition: audio_data.c:216
common internal and external API header
AudioData * resample_out_buffer
buffer for output from resampler
Definition: libavresample/internal.h:89
these buffered frames must be flushed immediately if a new input produces new output(Example:frame rate-doubling filter:filter_frame must(1) flush the second copy of the previous frame, if it is still there,(2) push the first copy of the incoming frame,(3) keep the second copy for later.) If the input frame is not enough to produce output
AudioMix * ff_audio_mix_alloc(AVAudioResampleContext *avr)
Allocate and initialize an AudioMix context.
Definition: audio_mix.c:341
unsigned avresample_version(void)
Return the LIBAVRESAMPLE_VERSION_INT constant.
Definition: libavresample/utils.c:621
int avresample_open(AVAudioResampleContext *avr)
Initialize AVAudioResampleContext.
Definition: libavresample/utils.c:35
int ff_audio_mix_get_matrix(AudioMix *am, double *matrix, int stride)
Get the current mixing matrix.
Definition: audio_mix.c:483
Generated on Sun Mar 23 2025 06:53:13 for FFmpeg by
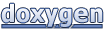