FFmpeg
|
tedcaptionsdec.c
Go to the documentation of this file.
static int tedcaptions_read_seek(AVFormatContext *avf, int stream_index, int64_t min_ts, int64_t ts, int64_t max_ts, int flags)
Definition: tedcaptionsdec.c:346
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
void ff_subtitles_queue_clean(FFDemuxSubtitlesQueue *q)
Remove and destroy all the subtitles packets.
Definition: subtitles.c:134
Definition: avformat.h:456
AVPacket * ff_subtitles_queue_insert(FFDemuxSubtitlesQueue *q, const uint8_t *event, int len, int merge)
Insert a new subtitle event.
Definition: subtitles.c:26
text(-8, 1,'a)')
int av_bprint_finalize(AVBPrint *buf, char **ret_str)
Finalize a print buffer.
Definition: bprint.c:193
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
static const AVClass tedcaptions_demuxer_class
Definition: tedcaptionsdec.c:43
AVOptions.
int ff_subtitles_queue_read_packet(FFDemuxSubtitlesQueue *q, AVPacket *pkt)
Generic read_packet() callback for subtitles demuxers using this queue system.
Definition: subtitles.c:82
static int tedcaptions_read_packet(AVFormatContext *avf, AVPacket *packet)
Definition: tedcaptionsdec.c:308
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
void av_bprint_init(AVBPrint *buf, unsigned size_init, unsigned size_max)
Init a print buffer.
Definition: bprint.c:68
static int av_bprint_is_complete(AVBPrint *buf)
Test if the print buffer is complete (not truncated).
Definition: bprint.h:166
static int parse_label(AVIOContext *pb, int *cur_byte, AVBPrint *bp)
Definition: tedcaptionsdec.c:141
static int tedcaptions_read_close(AVFormatContext *avf)
Definition: tedcaptionsdec.c:315
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
Definition: subtitles.h:28
static int parse_file(AVIOContext *pb, FFDemuxSubtitlesQueue *subs)
Definition: tedcaptionsdec.c:190
void ff_subtitles_queue_finalize(FFDemuxSubtitlesQueue *q)
Set missing durations and sort subtitles by PTS, and then byte position.
Definition: subtitles.c:72
Definition: tedcaptionsdec.c:29
static av_cold int tedcaptions_read_header(AVFormatContext *avf)
Definition: tedcaptionsdec.c:274
int ff_subtitles_queue_seek(FFDemuxSubtitlesQueue *q, AVFormatContext *s, int stream_index, int64_t min_ts, int64_t ts, int64_t max_ts, int flags)
Update current_sub_idx to emulate a seek.
Definition: subtitles.c:95
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
static int expect_byte(AVIOContext *pb, int *cur_byte, uint8_t c)
Definition: tedcaptionsdec.c:84
int64_t start_time
Decoding: pts of the first frame of the stream in presentation order, in stream time base...
Definition: avformat.h:689
Definition: opt.h:238
static int parse_string(AVIOContext *pb, int *cur_byte, AVBPrint *bp, int full)
Definition: tedcaptionsdec.c:93
static int parse_boolean(AVIOContext *pb, int *cur_byte, int *result)
Definition: tedcaptionsdec.c:154
Definition: avutil.h:146
static av_cold int tedcaptions_read_probe(AVProbeData *p)
Definition: tedcaptionsdec.c:323
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
void av_bprint_chars(AVBPrint *buf, char c, unsigned n)
Append char c n times to a print buffer.
Definition: bprint.c:116
static int parse_int(AVIOContext *pb, int *cur_byte, int64_t *result)
Definition: tedcaptionsdec.c:175
Generated on Thu May 29 2025 06:52:54 for FFmpeg by
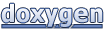