svcore
1.9
|
A model representing image annotations, identified by filename or URI, at a given time, with an optional label. More...
#include <ImageModel.h>
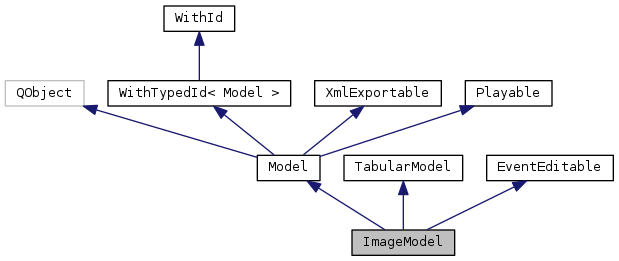
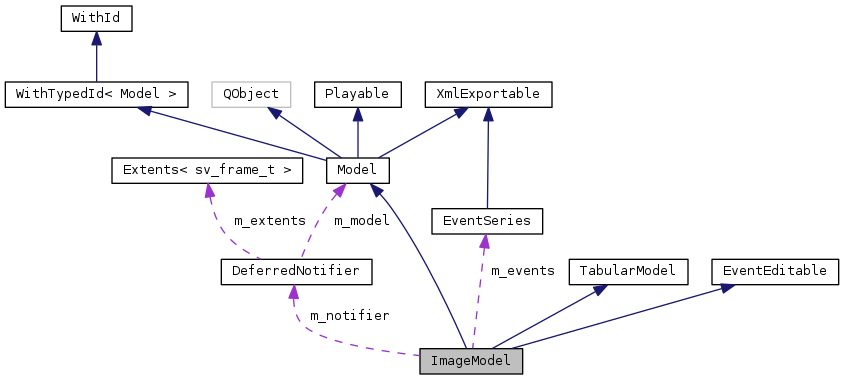
Public Types | |
typedef Id | ModelId |
typedef TypedId< Model > | Id |
enum | { NO_ID = -1 } |
typedef int | ExportId |
enum | { SortRole = Qt::UserRole } |
enum | SortType { SortNumeric, SortAlphabetical } |
Signals | |
void | modelChanged (ModelId myId) |
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached model that generates slowly) More... | |
void | modelChangedWithin (ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame) |
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached model that generates slowly) More... | |
void | completionChanged (ModelId myId) |
Emitted when some internal processing has advanced a stage, but the model has not changed externally. More... | |
void | ready (ModelId myId) |
Emitted when internal processing is complete (i.e. More... | |
void | alignmentCompletionChanged (ModelId myId) |
Emitted when the completion percentage changes for the calculation of this model's alignment model. More... | |
Public Member Functions | |
ImageModel (sv_samplerate_t sampleRate, int resolution, bool notifyOnAdd=true) | |
QString | getTypeName () const override |
Return the type of the model. More... | |
bool | isSparse () const override |
Return true if this is a sparse model. More... | |
bool | isOK () const override |
Return true if the model was constructed successfully. More... | |
sv_frame_t | getStartFrame () const override |
Return the first audio frame spanned by the model. More... | |
sv_frame_t | getTrueEndFrame () const override |
Return the audio frame at the end of the model. More... | |
sv_samplerate_t | getSampleRate () const override |
Return the frame rate in frames per second. More... | |
int | getResolution () const |
int | getCompletion () const override |
Return an estimated percentage value showing how far through any background operation used to calculate or load the model data the model thinks it is. More... | |
void | setCompletion (int completion, bool update=true) |
int | getEventCount () const |
Query methods. More... | |
bool | isEmpty () const |
bool | containsEvent (const Event &e) const |
EventVector | getAllEvents () const |
EventVector | getEventsSpanning (sv_frame_t f, sv_frame_t duration) const |
EventVector | getEventsCovering (sv_frame_t f) const |
EventVector | getEventsWithin (sv_frame_t f, sv_frame_t duration, int overspill=0) const |
EventVector | getEventsStartingWithin (sv_frame_t f, sv_frame_t duration) const |
EventVector | getEventsStartingAt (sv_frame_t f) const |
bool | getNearestEventMatching (sv_frame_t startSearchAt, std::function< bool(Event)> predicate, EventSeries::Direction direction, Event &found) const |
void | add (Event e) override |
Editing methods. More... | |
void | remove (Event e) override |
int | getRowCount () const override |
TabularModel methods. More... | |
int | getColumnCount () const override |
Return the number of columns (values/labels/etc per item). More... | |
bool | isColumnTimeValue (int column) const override |
Return true if the column is the frame time of the item, or an alternative representation of it (i.e. More... | |
sv_frame_t | getFrameForRow (int row) const override |
Return the frame time for the given row. More... | |
int | getRowForFrame (sv_frame_t frame) const override |
Return the number of the first row whose frame time is not less than the given one. More... | |
QString | getHeading (int column) const override |
Return the heading for a given column, e.g. More... | |
SortType | getSortType (int column) const override |
Return the sort type (numeric or alphabetical) for the column. More... | |
QVariant | getData (int row, int column, int role) const override |
Get the value in the given cell, for the given role. More... | |
Command * | getSetDataCommand (int row, int column, const QVariant &value, int role) override |
Return a command to set the value in the given cell, for the given role, to the contents of the supplied variant. More... | |
bool | isEditable () const override |
Return true if the model is user-editable, false otherwise. More... | |
Command * | getInsertRowCommand (int row) override |
Return a command to insert a new row before the row with the given index. More... | |
Command * | getRemoveRowCommand (int row) override |
Return a command to delete the row with the given index. More... | |
void | toXml (QTextStream &out, QString indent="", QString extraAttributes="") const override |
XmlExportable methods. More... | |
QVector< QString > | getStringExportHeaders (DataExportOptions options) const override |
Return a label for each column that would be written by toStringExportRows. More... | |
QVector< QVector< QString > > | toStringExportRows (DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override |
Emit events starting within the given range as string rows ready for conversion to an e.g. More... | |
sv_frame_t | getEndFrame () const |
Return the audio frame at the end of the model, i.e. More... | |
void | extendEndFrame (sv_frame_t to) |
Extend the end of the model. More... | |
virtual sv_samplerate_t | getNativeRate () const |
Return the frame rate of the underlying material, if the model itself has already been resampled. More... | |
virtual QString | getTitle () const |
Return the "work title" of the model, if known. More... | |
virtual QString | getMaker () const |
Return the "artist" or "maker" of the model, if known. More... | |
virtual QString | getLocation () const |
Return the location of the data in this model (e.g. More... | |
virtual bool | isReady (int *cp=nullptr) const |
Return true if the model has finished loading or calculating all its data, for a model that is capable of calculating in a background thread. More... | |
virtual const ZoomConstraint * | getZoomConstraint () const |
If this model imposes a zoom constraint, i.e. More... | |
virtual ModelId | getSourceModel () const |
If this model was derived from another, return the id of the model it was derived from. More... | |
virtual void | setSourceModel (ModelId model) |
Set the source model for this model. More... | |
virtual void | setAlignment (ModelId alignmentModel) |
Specify an alignment between this model's timeline and that of a reference model. More... | |
virtual const ModelId | getAlignment () const |
Retrieve the alignment model for this model. More... | |
virtual const ModelId | getAlignmentReference () const |
Return the reference model for the current alignment timeline, if any. More... | |
virtual sv_frame_t | alignToReference (sv_frame_t frame) const |
Return the frame number of the reference model that corresponds to the given frame number in this model. More... | |
virtual sv_frame_t | alignFromReference (sv_frame_t referenceFrame) const |
Return the frame number in this model that corresponds to the given frame number of the reference model. More... | |
virtual int | getAlignmentCompletion () const |
Return the completion percentage for the alignment model: 100 if there is no alignment model or it has been entirely calculated, or less than 100 if it is still being calculated. More... | |
void | setRDFTypeURI (QString uri) |
Set the event, feature, or signal type URI for the features contained in this model, according to the Audio Features RDF ontology. More... | |
QString | getRDFTypeURI () const |
Retrieve the event, feature, or signal type URI for the features contained in this model, if previously set with setRDFTypeURI. More... | |
ExportId | getExportId () const |
Return the numerical export identifier for this object. More... | |
virtual QString | toXmlString (QString indent="", QString extraAttributes="") const |
Convert this exportable object to XML in a string. More... | |
virtual bool | canPlay () const |
virtual QString | getDefaultPlayClipId () const |
virtual bool | getDefaultPlayAudible () const |
Static Public Member Functions | |
static QString | encodeEntities (QString) |
static QString | encodeColour (int r, int g, int b) |
Protected Member Functions | |
Id | getId () const |
Return an id for this object. More... | |
int | getUntypedId () const |
Return an id for this object. More... | |
Static Protected Member Functions | |
static QVariant | adaptFrameForRole (sv_frame_t frame, sv_samplerate_t rate, int role) |
static QVariant | adaptValueForRole (float value, QString unit, int role) |
Protected Attributes | |
sv_samplerate_t | m_sampleRate |
int | m_resolution |
DeferredNotifier | m_notifier |
std::atomic< int > | m_completion |
EventSeries | m_events |
QMutex | m_mutex |
ModelId | m_sourceModel |
ModelId | m_alignmentModel |
QString | m_typeUri |
std::atomic< sv_frame_t > | m_extendTo |
Detailed Description
A model representing image annotations, identified by filename or URI, at a given time, with an optional label.
The filename can be empty, in which case we instead have a space to put an image in.
Definition at line 38 of file ImageModel.h.
Member Typedef Documentation
|
inherited |
|
inherited |
Definition at line 33 of file XmlExportable.h.
Member Enumeration Documentation
|
inherited |
Enumerator | |
---|---|
NO_ID |
Definition at line 28 of file XmlExportable.h.
|
inherited |
Enumerator | |
---|---|
SortRole |
Definition at line 57 of file TabularModel.h.
|
inherited |
Enumerator | |
---|---|
SortNumeric | |
SortAlphabetical |
Definition at line 58 of file TabularModel.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 45 of file ImageModel.h.
Member Function Documentation
|
inlineoverridevirtual |
Return the type of the model.
For display purposes only.
Implements Model.
Definition at line 58 of file ImageModel.h.
|
inlineoverridevirtual |
Return true if this is a sparse model.
Reimplemented from Model.
Definition at line 59 of file ImageModel.h.
|
inlineoverridevirtual |
Return true if the model was constructed successfully.
Classes that refer to the model should always test this before use.
Implements Model.
Definition at line 60 of file ImageModel.h.
|
inlineoverridevirtual |
Return the first audio frame spanned by the model.
Implements Model.
Definition at line 62 of file ImageModel.h.
References EventSeries::getStartFrame(), and m_events.
|
inlineoverridevirtual |
Return the audio frame at the end of the model.
This is identical to getEndFrame(), except that it ignores any extended duration set with extendEndFrame().
Implements Model.
Definition at line 65 of file ImageModel.h.
References EventSeries::getEndFrame(), EventSeries::isEmpty(), m_events, and m_resolution.
|
inlineoverridevirtual |
Return the frame rate in frames per second.
Implements Model.
Definition at line 72 of file ImageModel.h.
References m_sampleRate.
Referenced by getData(), and getSetDataCommand().
|
inline |
Definition at line 73 of file ImageModel.h.
References m_resolution.
|
inlineoverridevirtual |
Return an estimated percentage value showing how far through any background operation used to calculate or load the model data the model thinks it is.
Must return 100 when the model is complete.
A model that carries out all its calculation from the constructor or accessor functions might return 0 if isOK() is false and 100 if isOK() is true. Other models may make the return value here depend on the internal completion status.
See also isReady().
Implements Model.
Definition at line 75 of file ImageModel.h.
References m_completion.
|
inline |
Definition at line 77 of file ImageModel.h.
References Model::completionChanged(), WithTypedId< Model >::getId(), m_completion, m_notifier, DeferredNotifier::makeDeferredNotifications(), Model::modelChanged(), DeferredNotifier::NOTIFY_ALWAYS, and DeferredNotifier::switchMode().
|
inline |
Query methods.
!! todo: go through all models, weeding out the methods here !! that are not used
Definition at line 102 of file ImageModel.h.
References EventSeries::count(), and m_events.
|
inline |
Definition at line 105 of file ImageModel.h.
References EventSeries::isEmpty(), and m_events.
|
inline |
Definition at line 108 of file ImageModel.h.
References EventSeries::contains(), and m_events.
|
inline |
Definition at line 111 of file ImageModel.h.
References EventSeries::getAllEvents(), and m_events.
|
inline |
Definition at line 114 of file ImageModel.h.
References EventSeries::getEventsSpanning(), and m_events.
|
inline |
Definition at line 117 of file ImageModel.h.
References EventSeries::getEventsCovering(), and m_events.
|
inline |
Definition at line 120 of file ImageModel.h.
References EventSeries::getEventsWithin(), and m_events.
|
inline |
Definition at line 124 of file ImageModel.h.
References EventSeries::getEventsStartingWithin(), and m_events.
|
inline |
Definition at line 127 of file ImageModel.h.
References EventSeries::getEventsStartingAt(), and m_events.
|
inline |
Definition at line 130 of file ImageModel.h.
References EventSeries::getNearestEventMatching(), and m_events.
|
inlineoverridevirtual |
Editing methods.
Implements EventEditable.
Definition at line 141 of file ImageModel.h.
References EventSeries::add(), Event::getFrame(), m_events, m_notifier, m_resolution, DeferredNotifier::update(), Event::withoutDuration(), Event::withoutLevel(), and Event::withoutValue().
|
inlineoverridevirtual |
Implements EventEditable.
Definition at line 146 of file ImageModel.h.
References WithTypedId< Model >::getId(), m_events, m_resolution, Model::modelChangedWithin(), and EventSeries::remove().
|
inlineoverridevirtual |
TabularModel methods.
Implements TabularModel.
Definition at line 156 of file ImageModel.h.
References EventSeries::count(), and m_events.
|
inlineoverridevirtual |
Return the number of columns (values/labels/etc per item).
Implements TabularModel.
Definition at line 160 of file ImageModel.h.
|
inlineoverridevirtual |
Return true if the column is the frame time of the item, or an alternative representation of it (i.e.
anything that has the same sort order). Duration is not a time value by this meaning.
Implements TabularModel.
Definition at line 164 of file ImageModel.h.
|
inlineoverridevirtual |
Return the frame time for the given row.
Implements TabularModel.
Definition at line 168 of file ImageModel.h.
References EventSeries::count(), EventSeries::getEventByIndex(), Event::getFrame(), and m_events.
|
inlineoverridevirtual |
Return the number of the first row whose frame time is not less than the given one.
If there is none, return getRowCount().
Implements TabularModel.
Definition at line 176 of file ImageModel.h.
References EventSeries::getIndexForEvent(), and m_events.
|
inlineoverridevirtual |
Return the heading for a given column, e.g.
"Time" or "Value". These are shown directly to the user, so must be translated already.
Implements TabularModel.
Definition at line 180 of file ImageModel.h.
|
inlineoverridevirtual |
Return the sort type (numeric or alphabetical) for the column.
Implements TabularModel.
Definition at line 190 of file ImageModel.h.
References TabularModel::SortAlphabetical, and TabularModel::SortNumeric.
|
inlineoverridevirtual |
Get the value in the given cell, for the given role.
The role is actually a Qt::ItemDataRole.
Implements TabularModel.
Definition at line 195 of file ImageModel.h.
References TabularModel::adaptFrameForRole(), EventSeries::count(), EventSeries::getEventByIndex(), Event::getFrame(), Event::getLabel(), getSampleRate(), Event::getURI(), and m_events.
|
inlineoverridevirtual |
Return a command to set the value in the given cell, for the given role, to the contents of the supplied variant.
If the model is not editable or the cell or value is out of range, return nullptr.
Implements TabularModel.
Definition at line 212 of file ImageModel.h.
References EventSeries::count(), EventSeries::getEventByIndex(), WithTypedId< Model >::getId(), getSampleRate(), m_events, Event::withFrame(), Event::withLabel(), and Event::withURI().
|
inlineoverridevirtual |
Return true if the model is user-editable, false otherwise.
Implements TabularModel.
Definition at line 234 of file ImageModel.h.
|
inlineoverridevirtual |
Return a command to insert a new row before the row with the given index.
If the model is not editable or the index is out of range, return nullptr.
Implements TabularModel.
Definition at line 236 of file ImageModel.h.
References EventSeries::count(), EventSeries::getEventByIndex(), WithTypedId< Model >::getId(), and m_events.
|
inlineoverridevirtual |
Return a command to delete the row with the given index.
If the model is not editable or the index is out of range, return nullptr.
Implements TabularModel.
Definition at line 245 of file ImageModel.h.
References EventSeries::count(), EventSeries::getEventByIndex(), WithTypedId< Model >::getId(), and m_events.
|
inlineoverridevirtual |
XmlExportable methods.
Implements XmlExportable.
Definition at line 257 of file ImageModel.h.
References XmlExportable::getExportId(), m_events, m_resolution, EventSeries::toXml(), Model::toXml(), and Event::ExportNameOptions::uriAttributeName.
|
inlineoverridevirtual |
Return a label for each column that would be written by toStringExportRows.
Implements Model.
Definition at line 279 of file ImageModel.h.
References EventSeries::getStringExportHeaders(), m_events, and Event::ExportNameOptions::uriAttributeName.
|
inlineoverridevirtual |
Emit events starting within the given range as string rows ready for conversion to an e.g.
comma-separated data format.
Implements Model.
Definition at line 286 of file ImageModel.h.
References m_events, m_resolution, m_sampleRate, and EventSeries::toStringExportRows().
|
inlineinherited |
Return the audio frame at the end of the model, i.e.
the final frame contained within the model plus 1 (rounded up to the model's "resolution" granularity, if more than 1). The end frame minus the start frame should yield the total duration in frames (as a multiple of the resolution) spanned by the model. This is broadly consistent with the definition of the end frame of a Selection object.
If the end has been extended by extendEndFrame() beyond the true end frame, return the extended end instead. This is usually the behaviour you want.
Definition at line 87 of file Model.h.
References Model::getTrueEndFrame(), and Model::m_extendTo.
Referenced by Model::alignFromReference(), ReadOnlyWaveFileModel::cacheFilled(), WaveformOversampler::getFixedRatioData(), SparseOneDimensionalModel::getNotes(), NoteModel::getNotes(), ReadOnlyWaveFileModel::isReady(), Model::toXml(), CSVFileWriter::write(), CSVStreamWriter::writeInChunks(), and WavFileWriter::writeModel().
|
inlineinherited |
Extend the end of the model.
If this is set to something beyond the true end of the data within the model, then getEndFrame() will return this value instead of the true end. (This is used by the Tony application.)
Definition at line 109 of file Model.h.
References Model::getSampleRate(), and Model::m_extendTo.
|
inlinevirtualinherited |
Return the frame rate of the underlying material, if the model itself has already been resampled.
Reimplemented in WritableWaveFileModel, ReadOnlyWaveFileModel, and WaveFileModel.
Definition at line 122 of file Model.h.
References Model::getLocation(), Model::getMaker(), Model::getSampleRate(), Model::getTitle(), and Model::getTypeName().
|
virtualinherited |
Return the "work title" of the model, if known.
Reimplemented in WritableWaveFileModel, ReadOnlyWaveFileModel, and WaveFileModel.
Definition at line 177 of file Model.cpp.
References TypedById< Item, Id >::get(), Model::m_mutex, and Model::m_sourceModel.
Referenced by Model::getNativeRate().
|
virtualinherited |
Return the "artist" or "maker" of the model, if known.
Reimplemented in WritableWaveFileModel, ReadOnlyWaveFileModel, and WaveFileModel.
Definition at line 186 of file Model.cpp.
References TypedById< Item, Id >::get(), Model::m_mutex, and Model::m_sourceModel.
Referenced by Model::getNativeRate().
|
virtualinherited |
Return the location of the data in this model (e.g.
source URL). This should not normally be returned for editable models that have been edited.
Reimplemented in WritableWaveFileModel, ReadOnlyWaveFileModel, and WaveFileModel.
Definition at line 195 of file Model.cpp.
References TypedById< Item, Id >::get(), Model::m_mutex, and Model::m_sourceModel.
Referenced by Model::getNativeRate().
|
inlinevirtualinherited |
Return true if the model has finished loading or calculating all its data, for a model that is capable of calculating in a background thread.
If "completion" is non-NULL, return through it an estimated percentage value showing how far through the background operation it thinks it is (for progress reporting). This should be identical to the value returned by getCompletion().
A model that carries out all its calculation from the constructor or accessor functions would typically return true (and completion == 100) as long as isOK() is true. Other models may make the return value here depend on the internal completion status.
See also getCompletion().
Reimplemented in ReadOnlyWaveFileModel, AlignmentModel, BasicCompressedDenseThreeDimensionalModel, AggregateWaveModel, and EditableDenseThreeDimensionalModel.
Definition at line 169 of file Model.h.
References Model::getCompletion(), and Model::isOK().
|
inlinevirtualinherited |
If this model imposes a zoom constraint, i.e.
some limit to the set of resolutions at which its data can meaningfully be displayed, then return it.
Reimplemented in WritableWaveFileModel, ReadOnlyWaveFileModel, AggregateWaveModel, and AlignmentModel.
|
inlinevirtualinherited |
If this model was derived from another, return the id of the model it was derived from.
The assumption is that the source model's alignment will also apply to this model, unless some other property (such as a specific alignment model set on this model) indicates otherwise.
Definition at line 207 of file Model.h.
References Model::alignFromReference(), Model::alignToReference(), Model::getAlignment(), Model::getAlignmentCompletion(), Model::getAlignmentReference(), Model::m_sourceModel, Model::setAlignment(), and Model::setSourceModel().
|
virtualinherited |
Set the source model for this model.
Definition at line 31 of file Model.cpp.
References Model::alignmentCompletionChanged(), TypedById< Item, Id >::get(), Model::m_mutex, and Model::m_sourceModel.
Referenced by RDFImporterImpl::getDataModelsSparse(), and Model::getSourceModel().
|
virtualinherited |
Specify an alignment between this model's timeline and that of a reference model.
The alignment model, of type AlignmentModel, records both the reference and the alignment.
Definition at line 45 of file Model.cpp.
References Model::alignmentModelCompletionChanged(), Model::completionChanged(), TypedById< Item, Id >::get(), Model::m_alignmentModel, Model::m_mutex, and SVDEBUG.
Referenced by Model::getSourceModel().
|
virtualinherited |
Retrieve the alignment model for this model.
This is not a generally useful function, as the alignment you really want may be performed by the source model instead. You should normally use getAlignmentReference, alignToReference and alignFromReference instead of this. The main intended application for this function is in streaming out alignments to the session file.
Definition at line 72 of file Model.cpp.
References Model::m_alignmentModel, and Model::m_mutex.
Referenced by Model::getSourceModel().
|
virtualinherited |
Return the reference model for the current alignment timeline, if any.
Definition at line 79 of file Model.cpp.
References Model::m_alignmentModel, and Model::m_mutex.
Referenced by Model::getSourceModel().
|
virtualinherited |
Return the frame number of the reference model that corresponds to the given frame number in this model.
Definition at line 88 of file Model.cpp.
References TypedById< Item, Id >::get(), Model::m_alignmentModel, Model::m_mutex, and Model::m_sourceModel.
Referenced by Model::getSourceModel().
|
virtualinherited |
Return the frame number in this model that corresponds to the given frame number of the reference model.
Definition at line 116 of file Model.cpp.
References TypedById< Item, Id >::get(), Model::getEndFrame(), Model::m_alignmentModel, Model::m_mutex, and Model::m_sourceModel.
Referenced by Model::getSourceModel().
|
virtualinherited |
Return the completion percentage for the alignment model: 100 if there is no alignment model or it has been entirely calculated, or less than 100 if it is still being calculated.
Definition at line 141 of file Model.cpp.
References TypedById< Item, Id >::get(), Model::m_alignmentModel, Model::m_mutex, Model::m_sourceModel, and SVCERR.
Referenced by Model::getSourceModel().
|
inlineinherited |
Set the event, feature, or signal type URI for the features contained in this model, according to the Audio Features RDF ontology.
Definition at line 264 of file Model.h.
References Model::m_typeUri.
Referenced by FeatureExtractionModelTransformer::getAdditionalModel(), and RDFImporterImpl::getDataModelsSparse().
|
inlineinherited |
Retrieve the event, feature, or signal type URI for the features contained in this model, if previously set with setRDFTypeURI.
Definition at line 271 of file Model.h.
References Model::alignmentCompletionChanged(), Model::alignmentModelCompletionChanged(), Model::completionChanged(), Model::getStringExportHeaders(), Model::m_typeUri, Model::modelChanged(), Model::modelChangedWithin(), Model::ready(), Model::toStringExportRows(), and Model::toXml().
|
signalinherited |
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached model that generates slowly)
Referenced by BoxModel::add(), RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), AggregateWaveModel::AggregateWaveModel(), ReadOnlyWaveFileModel::cacheFilled(), AggregateWaveModel::componentModelChanged(), WritableWaveFileModel::componentModelChanged(), Dense3DModelPeakCache::Dense3DModelPeakCache(), FFTModel::FFTModel(), ReadOnlyWaveFileModel::fillTimerTimedOut(), Model::getRDFTypeURI(), WritableWaveFileModel::init(), EditableDenseThreeDimensionalModel::setBinName(), BasicCompressedDenseThreeDimensionalModel::setBinName(), EditableDenseThreeDimensionalModel::setBinNames(), BasicCompressedDenseThreeDimensionalModel::setBinNames(), EditableDenseThreeDimensionalModel::setColumn(), BasicCompressedDenseThreeDimensionalModel::setColumn(), EditableDenseThreeDimensionalModel::setCompletion(), BasicCompressedDenseThreeDimensionalModel::setCompletion(), TextModel::setCompletion(), setCompletion(), SparseOneDimensionalModel::setCompletion(), BoxModel::setCompletion(), RegionModel::setCompletion(), SparseTimeValueModel::setCompletion(), NoteModel::setCompletion(), and WritableWaveFileModel::writeComplete().
|
signalinherited |
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached model that generates slowly)
Referenced by AggregateWaveModel::AggregateWaveModel(), ReadOnlyWaveFileModel::cacheFilled(), AggregateWaveModel::componentModelChangedWithin(), WritableWaveFileModel::componentModelChangedWithin(), FFTModel::FFTModel(), ReadOnlyWaveFileModel::fillTimerTimedOut(), Model::getRDFTypeURI(), WritableWaveFileModel::init(), DeferredNotifier::makeDeferredNotifications(), remove(), TextModel::remove(), SparseOneDimensionalModel::remove(), BoxModel::remove(), RegionModel::remove(), SparseTimeValueModel::remove(), NoteModel::remove(), EditableDenseThreeDimensionalModel::setColumn(), BasicCompressedDenseThreeDimensionalModel::setColumn(), EditableDenseThreeDimensionalModel::setCompletion(), BasicCompressedDenseThreeDimensionalModel::setCompletion(), AlignmentModel::setPathFrom(), and DeferredNotifier::update().
|
signalinherited |
Emitted when some internal processing has advanced a stage, but the model has not changed externally.
Views should respond by updating any progress meters or other monitoring, but not refreshing the actual view.
Referenced by AggregateWaveModel::AggregateWaveModel(), AggregateWaveModel::componentModelCompletionChanged(), Model::getRDFTypeURI(), Model::setAlignment(), EditableDenseThreeDimensionalModel::setCompletion(), BasicCompressedDenseThreeDimensionalModel::setCompletion(), TextModel::setCompletion(), setCompletion(), SparseOneDimensionalModel::setCompletion(), BoxModel::setCompletion(), RegionModel::setCompletion(), SparseTimeValueModel::setCompletion(), and NoteModel::setCompletion().
|
signalinherited |
Emitted when internal processing is complete (i.e.
when isReady() would return true, with completion at 100).
Referenced by ReadOnlyWaveFileModel::cacheFilled(), Model::getRDFTypeURI(), AggregateWaveModel::isReady(), and ReadOnlyWaveFileModel::isReady().
|
signalinherited |
Emitted when the completion percentage changes for the calculation of this model's alignment model.
(The ModelId provided is that of this model, not the alignment model.)
Referenced by Model::alignmentModelCompletionChanged(), Model::getRDFTypeURI(), and Model::setSourceModel().
|
inlineprotectedinherited |
Return an id for this object.
The id is a unique value for this object among all objects that implement WithId within this single run of the application.
Definition at line 193 of file ById.h.
References TypedId< T >::untyped.
Referenced by BoxModel::add(), RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), Model::alignmentModelCompletionChanged(), ReadOnlyWaveFileModel::cacheFilled(), AggregateWaveModel::componentModelChanged(), WritableWaveFileModel::componentModelChanged(), AggregateWaveModel::componentModelChangedWithin(), WritableWaveFileModel::componentModelChangedWithin(), AggregateWaveModel::componentModelCompletionChanged(), ReadOnlyWaveFileModel::fillTimerTimedOut(), getInsertRowCommand(), TextModel::getInsertRowCommand(), SparseOneDimensionalModel::getInsertRowCommand(), RegionModel::getInsertRowCommand(), BoxModel::getInsertRowCommand(), SparseTimeValueModel::getInsertRowCommand(), NoteModel::getInsertRowCommand(), getRemoveRowCommand(), TextModel::getRemoveRowCommand(), SparseOneDimensionalModel::getRemoveRowCommand(), RegionModel::getRemoveRowCommand(), BoxModel::getRemoveRowCommand(), SparseTimeValueModel::getRemoveRowCommand(), NoteModel::getRemoveRowCommand(), getSetDataCommand(), TextModel::getSetDataCommand(), SparseOneDimensionalModel::getSetDataCommand(), RegionModel::getSetDataCommand(), BoxModel::getSetDataCommand(), SparseTimeValueModel::getSetDataCommand(), NoteModel::getSetDataCommand(), WritableWaveFileModel::init(), NoteModel::NoteModel(), AlignmentModel::pathSourceCompletionChanged(), ReadOnlyWaveFileModel::ReadOnlyWaveFileModel(), remove(), TextModel::remove(), SparseOneDimensionalModel::remove(), BoxModel::remove(), RegionModel::remove(), SparseTimeValueModel::remove(), NoteModel::remove(), EditableDenseThreeDimensionalModel::setBinName(), BasicCompressedDenseThreeDimensionalModel::setBinName(), EditableDenseThreeDimensionalModel::setBinNames(), BasicCompressedDenseThreeDimensionalModel::setBinNames(), EditableDenseThreeDimensionalModel::setColumn(), BasicCompressedDenseThreeDimensionalModel::setColumn(), EditableDenseThreeDimensionalModel::setCompletion(), BasicCompressedDenseThreeDimensionalModel::setCompletion(), AlignmentModel::setCompletion(), TextModel::setCompletion(), setCompletion(), SparseOneDimensionalModel::setCompletion(), BoxModel::setCompletion(), RegionModel::setCompletion(), SparseTimeValueModel::setCompletion(), NoteModel::setCompletion(), SparseOneDimensionalModel::SparseOneDimensionalModel(), SparseTimeValueModel::SparseTimeValueModel(), WritableWaveFileModel::writeComplete(), Model::~Model(), NoteModel::~NoteModel(), ReadOnlyWaveFileModel::~ReadOnlyWaveFileModel(), SparseOneDimensionalModel::~SparseOneDimensionalModel(), SparseTimeValueModel::~SparseTimeValueModel(), and WritableWaveFileModel::~WritableWaveFileModel().
|
inlineprotectedinherited |
|
inherited |
Return the numerical export identifier for this object.
It's allocated the first time this is called, so objects on which this is never called do not get allocated one.
Definition at line 71 of file XmlExportable.cpp.
References XmlExportable::m_exportId, and mutex.
Referenced by EditableDenseThreeDimensionalModel::toXml(), BasicCompressedDenseThreeDimensionalModel::toXml(), EventSeries::toXml(), toXml(), TextModel::toXml(), Model::toXml(), SparseOneDimensionalModel::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
virtualinherited |
Convert this exportable object to XML in a string.
The default implementation calls toXml and returns the result as a string. Do not override this unless you really know what you're doing.
Definition at line 25 of file XmlExportable.cpp.
References XmlExportable::toXml().
Referenced by ModelTransformerFactory::getConfigurationForTransform(), RDFTransformFactoryImpl::getTransforms(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 41 of file XmlExportable.cpp.
Referenced by TextMatcher::test(), PluginXml::toXml(), ReadOnlyWaveFileModel::toXml(), Transform::toXml(), WritableWaveFileModel::toXml(), Model::toXml(), Event::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 54 of file XmlExportable.cpp.
Referenced by XmlExportable::~XmlExportable().
|
inlinevirtualinherited |
Reimplemented in NoteModel, SparseTimeValueModel, SparseOneDimensionalModel, and DenseTimeValueModel.
Definition at line 26 of file Playable.h.
|
inlinevirtualinherited |
Reimplemented in NoteModel, SparseOneDimensionalModel, and DenseTimeValueModel.
Definition at line 27 of file Playable.h.
Referenced by PlayParameterRepository::addPlayable().
|
inlinevirtualinherited |
Reimplemented in SparseTimeValueModel.
Definition at line 28 of file Playable.h.
Referenced by PlayParameterRepository::addPlayable().
|
inlinestaticprotectedinherited |
Definition at line 124 of file TabularModel.h.
References RealTime::frame2RealTime(), TabularModel::SortRole, RealTime::toString(), and RealTime::toText().
Referenced by getData(), TextModel::getData(), SparseOneDimensionalModel::getData(), RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), and NoteModel::getData().
|
inlinestaticprotectedinherited |
Definition at line 133 of file TabularModel.h.
References TabularModel::SortRole.
Referenced by RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), and NoteModel::getData().
Member Data Documentation
|
protected |
Definition at line 298 of file ImageModel.h.
Referenced by getSampleRate(), and toStringExportRows().
|
protected |
Definition at line 299 of file ImageModel.h.
Referenced by add(), getResolution(), getTrueEndFrame(), remove(), toStringExportRows(), and toXml().
|
protected |
Definition at line 301 of file ImageModel.h.
Referenced by add(), and setCompletion().
|
protected |
Definition at line 302 of file ImageModel.h.
Referenced by getCompletion(), and setCompletion().
|
protected |
Definition at line 304 of file ImageModel.h.
Referenced by add(), containsEvent(), getAllEvents(), getData(), getEventCount(), getEventsCovering(), getEventsSpanning(), getEventsStartingAt(), getEventsStartingWithin(), getEventsWithin(), getFrameForRow(), getInsertRowCommand(), getNearestEventMatching(), getRemoveRowCommand(), getRowCount(), getRowForFrame(), getSetDataCommand(), getStartFrame(), getStringExportHeaders(), getTrueEndFrame(), isEmpty(), remove(), toStringExportRows(), and toXml().
|
mutableprotectedinherited |
Definition at line 337 of file Model.h.
Referenced by Model::alignFromReference(), Model::alignToReference(), Model::getAlignment(), Model::getAlignmentCompletion(), Model::getAlignmentReference(), Model::getLocation(), Model::getMaker(), SparseTimeValueModel::getScaleUnits(), NoteModel::getScaleUnits(), NoteModel::getStartFrame(), Model::getTitle(), NoteModel::getTrueEndFrame(), Model::setAlignment(), SparseTimeValueModel::setScaleUnits(), NoteModel::setScaleUnits(), and Model::setSourceModel().
|
protectedinherited |
Definition at line 338 of file Model.h.
Referenced by Model::alignFromReference(), Model::alignToReference(), Model::getAlignmentCompletion(), Model::getLocation(), Model::getMaker(), Model::getSourceModel(), Model::getTitle(), and Model::setSourceModel().
|
protectedinherited |
Definition at line 339 of file Model.h.
Referenced by Model::alignFromReference(), Model::alignToReference(), Model::getAlignment(), Model::getAlignmentCompletion(), Model::getAlignmentReference(), and Model::setAlignment().
|
protectedinherited |
Definition at line 340 of file Model.h.
Referenced by Model::getRDFTypeURI(), and Model::setRDFTypeURI().
|
protectedinherited |
Definition at line 341 of file Model.h.
Referenced by Model::extendEndFrame(), and Model::getEndFrame().
The documentation for this class was generated from the following file:
Generated by
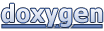