svcore
1.9
|
RegionModel.h
Go to the documentation of this file.
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: RegionModel.h:349
bool getNearestEventMatching(sv_frame_t startSearchAt, std::function< bool(Event)> predicate, EventSeries::Direction direction, Event &found) const
Definition: RegionModel.h:161
bool isColumnTimeValue(int column) const override
Return true if the column is the frame time of the item, or an alternative representation of it (i...
Definition: RegionModel.h:219
EventVector getEventsCovering(sv_frame_t f) const
Definition: RegionModel.h:149
EventVector getEventsStartingAt(sv_frame_t frame) const
Retrieve all events starting at exactly the given frame.
Definition: EventSeries.h:143
static QVariant adaptFrameForRole(sv_frame_t frame, sv_samplerate_t rate, int role)
Definition: TabularModel.h:124
sv_frame_t getFrameForRow(int row) const override
Return the frame time for the given row.
Definition: RegionModel.h:225
Event getEventByIndex(int index) const
Return the event at the given numerical index in the series, where 0 = the first event and count()-1 ...
Definition: EventSeries.cpp:547
int getColumnCount() const override
Return the number of columns (values/labels/etc per item).
Definition: RegionModel.h:215
void update(sv_frame_t frame, sv_frame_t duration)
Definition: DeferredNotifier.h:43
EventVector getAllEvents() const
Retrieve all events, in their natural order.
Definition: EventSeries.cpp:464
EventVector getEventsSpanning(sv_frame_t f, sv_frame_t duration) const
Definition: RegionModel.h:146
sv_frame_t getStartFrame() const
Return the frame of the first event in the series.
Definition: EventSeries.cpp:265
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration, sv_samplerate_t sampleRate, sv_frame_t resolution, Event fillEvent) const
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: EventSeries.cpp:615
Command * getRemoveRowCommand(int row) override
Return a command to delete the row with the given index.
Definition: RegionModel.h:305
RegionModel(sv_samplerate_t sampleRate, int resolution, bool notifyOnAdd=true)
Definition: RegionModel.h:40
Command * getInsertRowCommand(int row) override
Return a command to insert a new row before the row with the given index.
Definition: RegionModel.h:296
Definition: DeferredNotifier.h:25
Definition: TabularModel.h:58
void makeDeferredNotifications()
Definition: DeferredNotifier.h:53
QVector< QString > getStringExportHeaders(DataExportOptions options, Event::ExportNameOptions) const
Return a label for each column that would be written by toStringExportRows.
Definition: EventSeries.cpp:604
void setCompletion(int completion, bool update=true)
Definition: RegionModel.h:112
SortType getSortType(int column) const override
Return the sort type (numeric or alphabetical) for the column.
Definition: RegionModel.h:248
Definition: TabularModel.h:58
int getIndexForEvent(const Event &e) const
Return the index of the first event in the series that does not compare inferior to the given event...
Definition: EventSeries.cpp:557
void toXml(QTextStream &out, QString indent, QString extraAttributes) const override
Emit to XML as a dataset element.
Definition: EventSeries.cpp:567
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: RegionModel.h:110
EventVector getEventsCovering(sv_frame_t frame) const
Retrieve all events that cover the given frame.
Definition: EventSeries.cpp:420
QString getHeading(int column) const override
Return the heading for a given column, e.g.
Definition: RegionModel.h:237
QVariant getData(int row, int column, int role) const override
Get the value in the given cell, for the given role.
Definition: RegionModel.h:253
static QVariant adaptValueForRole(float value, QString unit, int role)
Definition: TabularModel.h:133
EventVector getEventsSpanning(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events any part of which falls within the range in frames defined by the given frame f a...
Definition: EventSeries.cpp:294
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
XmlExportable methods.
Definition: RegionModel.h:318
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
TabularModel is an abstract base class for models that support direct access to data in a tabular for...
Definition: TabularModel.h:35
void completionChanged(ModelId myId)
Emitted when some internal processing has advanced a stage, but the model has not changed externally...
sv_frame_t getEndFrame() const
Return the frame plus duration of the event in the series that ends last.
Definition: EventSeries.cpp:273
EventVector getEventsWithin(sv_frame_t f, sv_frame_t duration) const
Definition: RegionModel.h:152
ExportId getExportId() const
Return the numerical export identifier for this object.
Definition: XmlExportable.cpp:71
bool getNearestEventMatching(sv_frame_t startSearchAt, std::function< bool(const Event &)> predicate, Direction direction, Event &found) const
Return the first event for which the given predicate returns true, searching events with start frames...
Definition: EventSeries.cpp:508
RegionModel(sv_samplerate_t sampleRate, int resolution, float valueMinimum, float valueMaximum, bool notifyOnAdd=true)
Definition: RegionModel.h:58
RegionModel – a model for intervals associated with a value, which we call regions for no very compe...
Definition: RegionModel.h:33
bool isOK() const override
Return true if the model was constructed successfully.
Definition: RegionModel.h:81
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
EventVector getEventsWithin(sv_frame_t frame, sv_frame_t duration, int overspill=0) const
Retrieve all events falling wholly within the range in frames defined by the given frame f and durati...
Definition: EventSeries.cpp:342
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
Definition: Command.h:26
Container storing a series of events, with or without durations, and supporting the ability to query ...
Definition: EventSeries.h:49
EventVector getEventsStartingWithin(sv_frame_t f, sv_frame_t duration) const
Definition: RegionModel.h:155
int getRowForFrame(sv_frame_t frame) const override
Return the number of the first row whose frame time is not less than the given one.
Definition: RegionModel.h:233
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: RegionModel.h:93
QVector< QString > getStringExportHeaders(DataExportOptions options) const override
Return a label for each column that would be written by toStringExportRows.
Definition: RegionModel.h:344
Interface for classes that can be modified through these commands.
Definition: EventCommands.h:26
EventVector getEventsStartingAt(sv_frame_t f) const
Definition: RegionModel.h:158
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: RegionModel.h:83
EventVector getEventsStartingWithin(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events starting within the range in frames defined by the given frame f and duration d...
Definition: EventSeries.cpp:395
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Command to add or remove a series of events to or from an editable, with undo.
Definition: EventCommands.h:115
Command * getSetDataCommand(int row, int column, const QVariant &value, int role) override
Return a command to set the value in the given cell, for the given role, to the contents of the suppl...
Definition: RegionModel.h:273
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: RegionModel.h:86
bool isEditable() const override
Return true if the model is user-editable, false otherwise.
Definition: RegionModel.h:271
Generated by
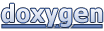