svcore
1.9
|
Model.h
Go to the documentation of this file.
QString getRDFTypeURI() const
Retrieve the event, feature, or signal type URI for the features contained in this model...
Definition: Model.h:271
void alignmentCompletionChanged(ModelId myId)
Emitted when the completion percentage changes for the calculation of this model's alignment model...
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
virtual ModelId getSourceModel() const
If this model was derived from another, return the id of the model it was derived from...
Definition: Model.h:207
virtual sv_frame_t alignFromReference(sv_frame_t referenceFrame) const
Return the frame number in this model that corresponds to the given frame number of the reference mod...
Definition: Model.cpp:116
virtual void setAlignment(ModelId alignmentModel)
Specify an alignment between this model's timeline and that of a reference model. ...
Definition: Model.cpp:45
virtual const ZoomConstraint * getZoomConstraint() const
If this model imposes a zoom constraint, i.e.
Definition: Model.h:196
virtual sv_frame_t getTrueEndFrame() const =0
Return the audio frame at the end of the model.
virtual int getAlignmentCompletion() const
Return the completion percentage for the alignment model: 100 if there is no alignment model or it ha...
Definition: Model.cpp:141
void setRDFTypeURI(QString uri)
Set the event, feature, or signal type URI for the features contained in this model, according to the Audio Features RDF ontology.
Definition: Model.h:264
Definition: XmlExportable.h:25
void alignmentModelCompletionChanged(ModelId)
Definition: Model.cpp:66
virtual QString getLocation() const
Return the location of the data in this model (e.g.
Definition: Model.cpp:195
Definition: ById.h:177
virtual QString getMaker() const
Return the "artist" or "maker" of the model, if known.
Definition: Model.cpp:186
sv_frame_t getEndFrame() const
Return the audio frame at the end of the model, i.e.
Definition: Model.h:87
virtual bool isReady(int *cp=nullptr) const
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: Model.h:169
virtual const ModelId getAlignmentReference() const
Return the reference model for the current alignment timeline, if any.
Definition: Model.cpp:79
virtual QVector< QString > getStringExportHeaders(DataExportOptions options) const =0
Return a label for each column that would be written by toStringExportRows.
Model & operator=(const Model &)=delete
virtual QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const =0
Emit events starting within the given range as string rows ready for conversion to an e...
virtual sv_samplerate_t getSampleRate() const =0
Return the frame rate in frames per second.
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
void completionChanged(ModelId myId)
Emitted when some internal processing has advanced a stage, but the model has not changed externally...
Definition: ById.h:225
Definition: AlignmentModel.h:28
virtual void setSourceModel(ModelId model)
Set the source model for this model.
Definition: Model.cpp:31
virtual sv_frame_t getStartFrame() const =0
Return the first audio frame spanned by the model.
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Definition: Playable.h:21
virtual sv_samplerate_t getNativeRate() const
Return the frame rate of the underlying material, if the model itself has already been resampled...
Definition: Model.h:122
virtual QString getTitle() const
Return the "work title" of the model, if known.
Definition: Model.cpp:177
ZoomConstraint is a simple interface that describes a limitation on the available zoom sizes for a vi...
Definition: ZoomConstraint.h:32
virtual int getCompletion() const =0
Return an estimated percentage value showing how far through any background operation used to calcula...
virtual const ModelId getAlignment() const
Retrieve the alignment model for this model.
Definition: Model.cpp:72
virtual sv_frame_t alignToReference(sv_frame_t frame) const
Return the frame number of the reference model that corresponds to the given frame number in this mod...
Definition: Model.cpp:88
Definition: ById.h:115
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Generated by
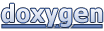