svcore
1.9
|
AlignmentModel.h
Go to the documentation of this file.
bool isOK() const override
Return true if the model was constructed successfully.
Definition: AlignmentModel.cpp:53
int getRelativePitch() const
Get the value set with setRelativePitch.
Definition: AlignmentModel.h:76
bool isReady(int *completion=0) const override
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: AlignmentModel.cpp:107
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: AlignmentModel.h:47
sv_frame_t toReference(sv_frame_t frame) const
Definition: AlignmentModel.cpp:172
void pathSourceCompletionChanged(ModelId)
Definition: AlignmentModel.cpp:218
sv_frame_t fromReference(sv_frame_t frame) const
Definition: AlignmentModel.cpp:191
void pathSourceChangedWithin(ModelId, sv_frame_t startFrame, sv_frame_t endFrame)
Definition: AlignmentModel.cpp:210
AlignmentModel(ModelId reference, ModelId aligned, ModelId path)
Definition: AlignmentModel.cpp:22
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: AlignmentModel.cpp:433
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: AlignmentModel.cpp:81
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: AlignmentModel.cpp:96
sv_frame_t performAlignment(const Path &path, sv_frame_t frame) const
Definition: AlignmentModel.cpp:322
QVector< QVector< QString > > toStringExportRows(DataExportOptions, sv_frame_t, sv_frame_t) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: AlignmentModel.h:88
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
const ZoomConstraint * getZoomConstraint() const override
If this model imposes a zoom constraint, i.e.
Definition: AlignmentModel.cpp:147
Definition: AlignmentModel.h:28
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: AlignmentModel.cpp:66
void setRelativePitch(int cents)
Set the calculated pitch relative to a reference.
Definition: AlignmentModel.h:71
A model representing a wiggly-line plot with points at arbitrary intervals of the model resolution...
Definition: SparseTimeValueModel.h:34
QVector< QString > getStringExportHeaders(DataExportOptions) const override
Return a label for each column that would be written by toStringExportRows.
Definition: AlignmentModel.h:83
ZoomConstraint is a simple interface that describes a limitation on the available zoom sizes for a vi...
Definition: ZoomConstraint.h:32
ModelId getReferenceModel() const
Definition: AlignmentModel.cpp:153
int m_explicitlySetCompletion
Definition: AlignmentModel.h:112
void constructReversePath() const
Definition: AlignmentModel.cpp:291
void completionChanged(ModelId)
Definition: ById.h:115
Generated by
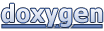